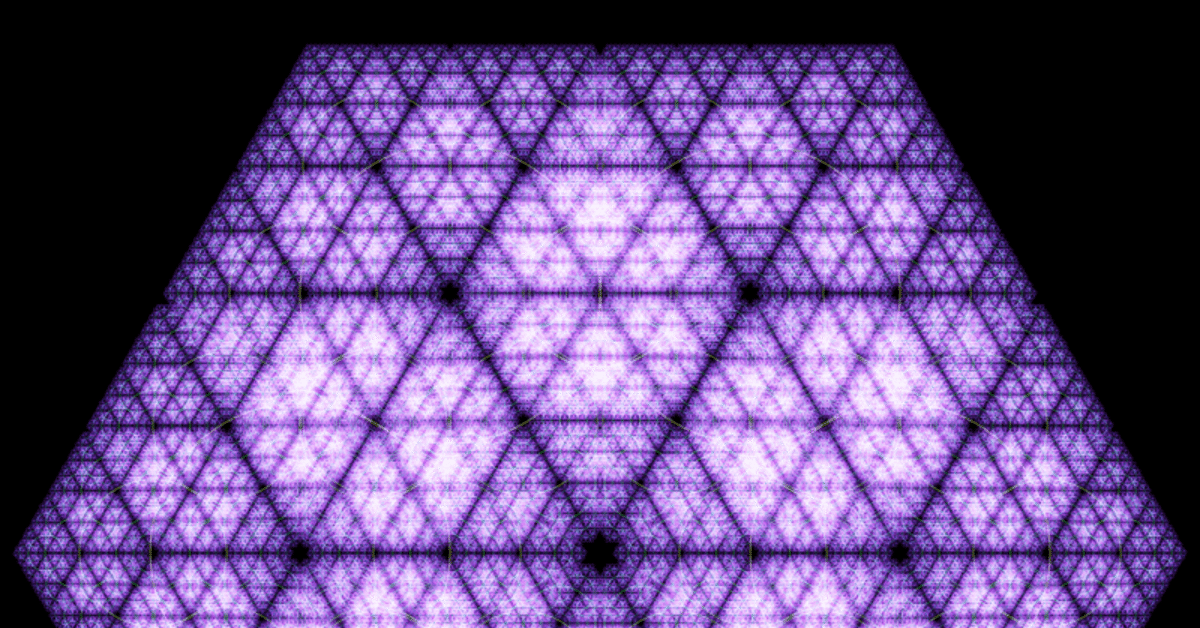
とりあえず100 #5
int n_Vert = 3; //描く多角形の頂点数
int n_Loop = 8; //ループ回数
float radScaleFac = 0.5;//ループの際スケールに掛け算する値
void setup() {
size(800, 800);
background(0);
noFill();
stroke(255, 100);
translate(width/2, height/1.7);
rotate(PI/n_Vert);
//↑図形の底面を水平にしたいときに使います
fractal(width/4, 0);
}
void fractal(float rad, int count) {
for (int i=0; i < n_Vert; i++) {
float ang = TWO_PI/n_Vert * i;
float x = sin(ang) * rad;
float y = cos(ang) * rad;
pushMatrix();
translate(x, y);
ellipse(0, 0, rad, rad);
if (count < n_Loop-1) {
fractal(rad * radScaleFac, count + 1);
}
popMatrix();
}
}
様々なフラクタル図形の作成に使える汎用的なコードを書いてみました。一応簡単な説明を。
ループ回数(n_Loop)を2回にしてみましょう。
大きい円で構成される三角形が一回目のループで描かれるものです。同心円状に頂点数(n_Vert)分、円を描きます。2回目以降は、一つ前のループで描いた各円の中心を中心にして、同じことを繰り返します。
これはベースのコードなので、ぜひ弄り回しちゃってください。
たとえば頂点数(n_Vert)を5にすると...。
五角形でフラクタル図形ができちゃいます!
※頂点数(n_Vert)を増やしすぎると計算回数が膨大になり処理が重たくなるので、ループ回数(n_Loop)を減らすなどして軽量化してください。
下記、派生版です。
個人的メモ
int n_Vert = 6 ; //描く多角形の頂点数
int n_Loop = 7; //ループ回数
float radScaleFac = 0.495;//ループの際スケールに掛け算する値
float startCol = random(360);
float endCol = 360 - startCol;
void setup() {
size(800, 800);
background(0);
blendMode(SCREEN);
colorMode(HSB, 360, 100, 100, 100);
noFill();
translate(width/2, height/2);
//rotate(PI/n_Vert);
fractal(width/4, 0);
}
void fractal(float rad, int count) {
for (int i=0; i < n_Vert; i++) {
rotate(map(i, 0, n_Vert, 0, TWO_PI));
float ang = TWO_PI/n_Vert * i;
float x = sin(ang) * rad;
float y = cos(ang) * rad;
pushMatrix();
translate(x, y);
float alph = map(count, 0, n_Loop, 10, 100);
float col = map(count, 0, n_Loop, startCol, endCol);
stroke(col, 80, 60, alph); strokeWeight(0.5);
ellipse(0, 0, rad, rad);
if (count < n_Loop-1) {
fractal(rad * radScaleFac, count + 1);
}
popMatrix();
}
}
int n_Vert = 6 ; //描く多角形の頂点数
int n_Loop = 7; //ループ回数
float radScaleFac = 0.5;//ループの際スケールに掛け算する値
float startCol = random(360);
float endCol = 360 - startCol;
void setup() {
size(800, 800);
background(0);
blendMode(SCREEN);
colorMode(HSB, 360, 100, 100, 100);
noFill();
translate(width/2, height/2);
rotate(PI/n_Vert);
fractal(width/4, 0);
}
void fractal(float rad, int count) {
for (int i=0; i < n_Vert; i++) {
rotate(map(i, 0, n_Vert, 0, TWO_PI*count));
float ang = TWO_PI/n_Vert * i;
float x = sin(ang) * rad;
float y = cos(ang) * rad;
pushMatrix();
translate(x, y);
float alph = map(count, 0, n_Loop, 10, 100);
float col = map(count, 0, n_Loop, startCol, endCol);
stroke(col, 80, 60, alph); strokeWeight(0.5);
ellipse(0, 0, rad, rad);
if (count < n_Loop-1) {
fractal(rad * radScaleFac, count + 1);
}
popMatrix();
}
}
int n_Vert = 6 ; //描く多角形の頂点数
int n_Loop = 7; //ループ回数
float radScaleFac = 0.5;//ループの際スケールに掛け算する値
float startCol = random(360);
float endCol = 360 - startCol;
void setup() {
size(800, 800);
background(0);
blendMode(SCREEN);
colorMode(HSB, 360, 100, 100, 100);
noFill();
translate(width/2, height/2);
rotate(PI/n_Vert);
fractal(width/4, 0);
}
void fractal(float rad, int count) {
for (int i=0; i < n_Vert; i++) {
rotate(map(i, 0, n_Vert, 0, TWO_PI*count));
float ang = TWO_PI/n_Vert * i;
float x = sin(ang) * rad;
float y = cos(ang) * rad;
pushMatrix();
translate(x, y);
float alph = map(count, 0, n_Loop, 10, 100);
float col = map(count, 0, n_Loop, startCol, endCol);
stroke(col, 80, 60, alph); strokeWeight(0.5);
ellipse(0, 0, rad, rad);
if (count < n_Loop-1) {
fractal(rad * radScaleFac, count + 1);
}
popMatrix();
}
}
int n_Vert = 6 ; //描く多角形の頂点数
int n_Loop = 7; //ループ回数
float radScaleFac = 0.5;//ループの際スケールに掛け算する値
float startCol = random(360);
float endCol = 360 - startCol;
void setup() {
size(800, 800);
background(0);
blendMode(SCREEN);
colorMode(HSB, 360, 100, 100, 100);
noFill();
translate(width/2, height/2);
rotate(PI/n_Vert);
fractal(width/4, 0);
}
void fractal(float rad, int count) {
for (int i=0; i < n_Vert; i++) {
rotate(map(i, 0, n_Vert, 0, TWO_PI*count));
float ang = TWO_PI/n_Vert * i;
float x = sin(ang) * rad;
float y = cos(ang) * rad;
pushMatrix();
translate(x, y);
float alph = map(count, 0, n_Loop, 10, 100);
float col = map(count, 0, n_Loop, startCol, endCol);
stroke(col, 80, 60, alph); strokeWeight(0.5);
ellipse(0, 0, rad, rad);
if (count < n_Loop-1) {
fractal(rad * radScaleFac, count + 1);
}
popMatrix();
}
}
int n_Vert = 6 ; //描く多角形の頂点数
int n_Loop = 7; //ループ回数
float radScaleFac = 0.5;//ループの際スケールに掛け算する値
float startCol = random(360);
float endCol = 360 - startCol;
void setup() {
size(800, 800);
background(0);
blendMode(SCREEN);
colorMode(HSB, 360, 100, 100, 100);
noFill();
translate(width/2, height/2);
rotate(PI/n_Vert);
fractal(width/4, 0);
}
void fractal(float rad, int count) {
for (int i=0; i < n_Vert; i++) {
rotate(map(i, 0, n_Vert, 0, TWO_PI * count * (i*3)));
float ang = TWO_PI/n_Vert * i;
float x = sin(ang) * rad;
float y = cos(ang) * rad;
pushMatrix();
translate(x, y);
float alph = map(count, 0, n_Loop, 10, 100);
float col = map(count, 0, n_Loop, startCol, endCol);
stroke(col, 80, 60, alph);
strokeWeight(0.5);
ellipse(0, 0, rad, rad);
if (count < n_Loop-1) {
fractal(rad * radScaleFac, count + 1);
}
popMatrix();
}
}
int n_Vert = 6 ; //描く多角形の頂点数
int n_Loop = 7; //ループ回数
float radScaleFac = 0.5;//ループの際スケールに掛け算する値
float startCol = random(360);
float endCol = 360 - startCol;
void setup() {
size(800, 800);
background(0);
blendMode(SCREEN);
colorMode(HSB, 360, 100, 100, 100);
noFill();
translate(width/2, height/2);
rotate(PI/n_Vert);
fractal(width/4, 0);
}
void fractal(float rad, int count) {
//rotate(PI * count);
for (int i=0; i < n_Vert; i++) {
rotate(PI * (i));
float ang = TWO_PI/n_Vert * i;
float x = sin(ang) * rad;
float y = cos(ang) * rad;
pushMatrix();
translate(x, y);
float alph = map(count, 0, n_Loop, 10, 100);
float col = map(count, 0, n_Loop, startCol, endCol);
stroke(col, 80, 60, alph);
strokeWeight(0.5);
ellipse(0, 0, rad, rad);
if (count < n_Loop-1) {
fractal(rad * radScaleFac, count + 1);
}
popMatrix();
}
}