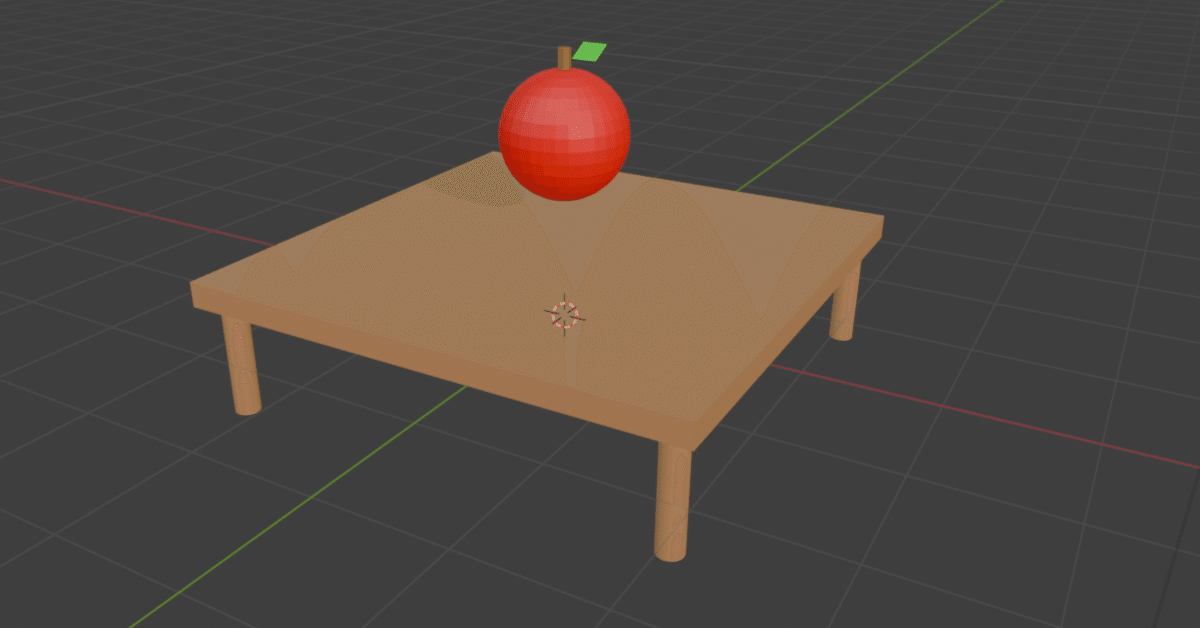
BlenderとDALL-E3で簡単3Dモデル作成
以下でChatGPT(LLM)を使った簡単3D文字作成手順を紹介している。
今回はDALL-E3で生成した画像を3Dモデルとして生成するための手順を紹介する。
ただし、複雑なオブジェクトの生成などにはまったく向かないのと、出力も安定しないのは、ご了承ください。
手順
複雑なモデルでなければなんでもよいので、以下のようなプロンプトで画像を生成する。
blender初心者が作成するのにちょうどよさそうなオブジェクトのサンプル画像を作成して
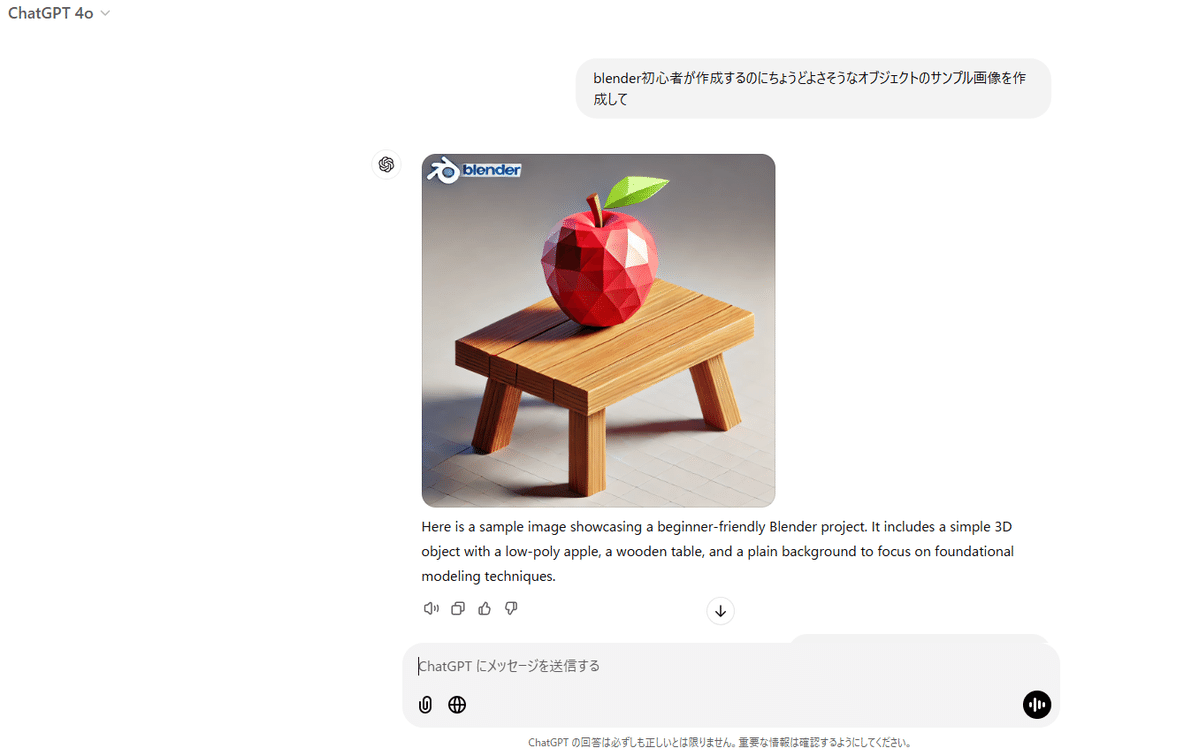
次に、以下のようなプロンプトでプログラムを作成する。
これを作成するためのスクリプトを作成して
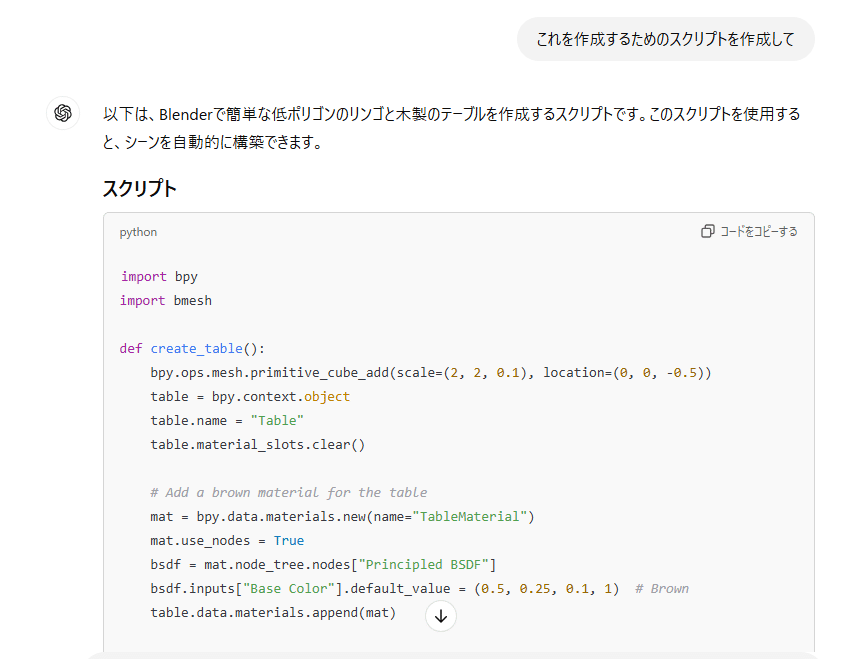
以下のようなプログラムが作成される。
import bpy
import bmesh
def create_table():
bpy.ops.mesh.primitive_cube_add(scale=(2, 2, 0.1), location=(0, 0, -0.5))
table = bpy.context.object
table.name = "Table"
table.material_slots.clear()
# Add a brown material for the table
mat = bpy.data.materials.new(name="TableMaterial")
mat.use_nodes = True
bsdf = mat.node_tree.nodes["Principled BSDF"]
bsdf.inputs["Base Color"].default_value = (0.5, 0.25, 0.1, 1) # Brown
table.data.materials.append(mat)
def create_apple():
bpy.ops.mesh.primitive_uv_sphere_add(radius=0.5, location=(0, 0, 0.5))
apple = bpy.context.object
apple.name = "Apple"
# Add a red material for the apple
mat = bpy.data.materials.new(name="AppleMaterial")
mat.use_nodes = True
bsdf = mat.node_tree.nodes["Principled BSDF"]
bsdf.inputs["Base Color"].default_value = (1, 0, 0, 1) # Red
apple.data.materials.append(mat)
# Add a leaf
bpy.ops.mesh.primitive_plane_add(size=0.2, location=(0, 0.3, 0.8))
leaf = bpy.context.object
leaf.name = "Leaf"
bpy.ops.object.mode_set(mode='EDIT')
bpy.ops.mesh.select_all(action='SELECT')
bpy.ops.transform.rotate(value=-0.5, orient_axis='X')
bpy.ops.object.mode_set(mode='OBJECT')
# Add a green material for the leaf
mat_leaf = bpy.data.materials.new(name="LeafMaterial")
mat_leaf.use_nodes = True
bsdf_leaf = mat_leaf.node_tree.nodes["Principled BSDF"]
bsdf_leaf.inputs["Base Color"].default_value = (0, 1, 0, 1) # Green
leaf.data.materials.append(mat_leaf)
def setup_scene():
# Set background color to white
bpy.data.worlds["World"].node_tree.nodes["Background"].inputs[0].default_value = (1, 1, 1, 1)
# Add a light source
bpy.ops.object.light_add(type='SUN', location=(5, 5, 5))
# Add a camera
bpy.ops.object.camera_add(location=(3, -3, 2), rotation=(1.1, 0, 0.8))
bpy.context.scene.camera = bpy.context.object
# Clear existing objects
bpy.ops.object.select_all(action='SELECT')
bpy.ops.object.delete(use_global=False)
# Create objects
create_table()
create_apple()
setup_scene()
print("Scene setup complete!")
ただ、これだけだと正直画像を再現しているとは言えない。
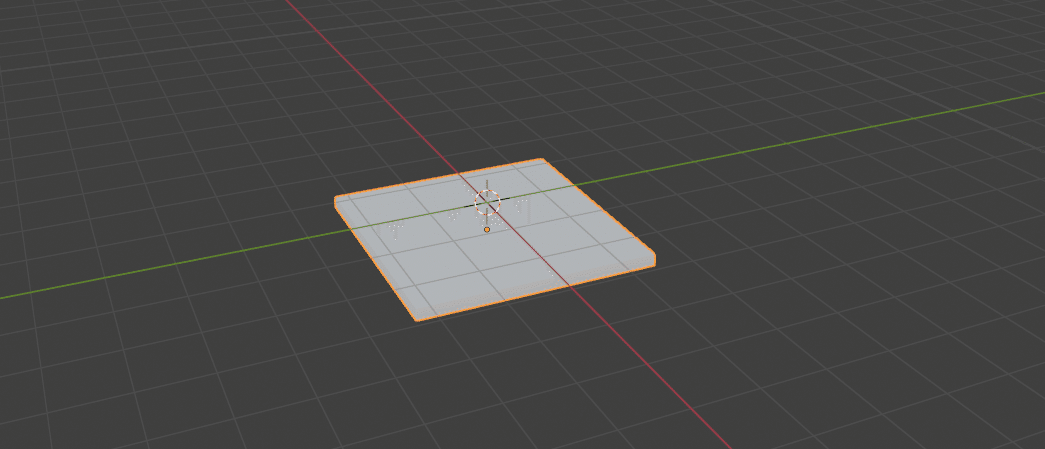
そこで以下のようなパワハラプロンプトで、プログラムを修正させる。
あなたの回答は60点だ。改善した要素を述べた上で100点の回答をしろ。
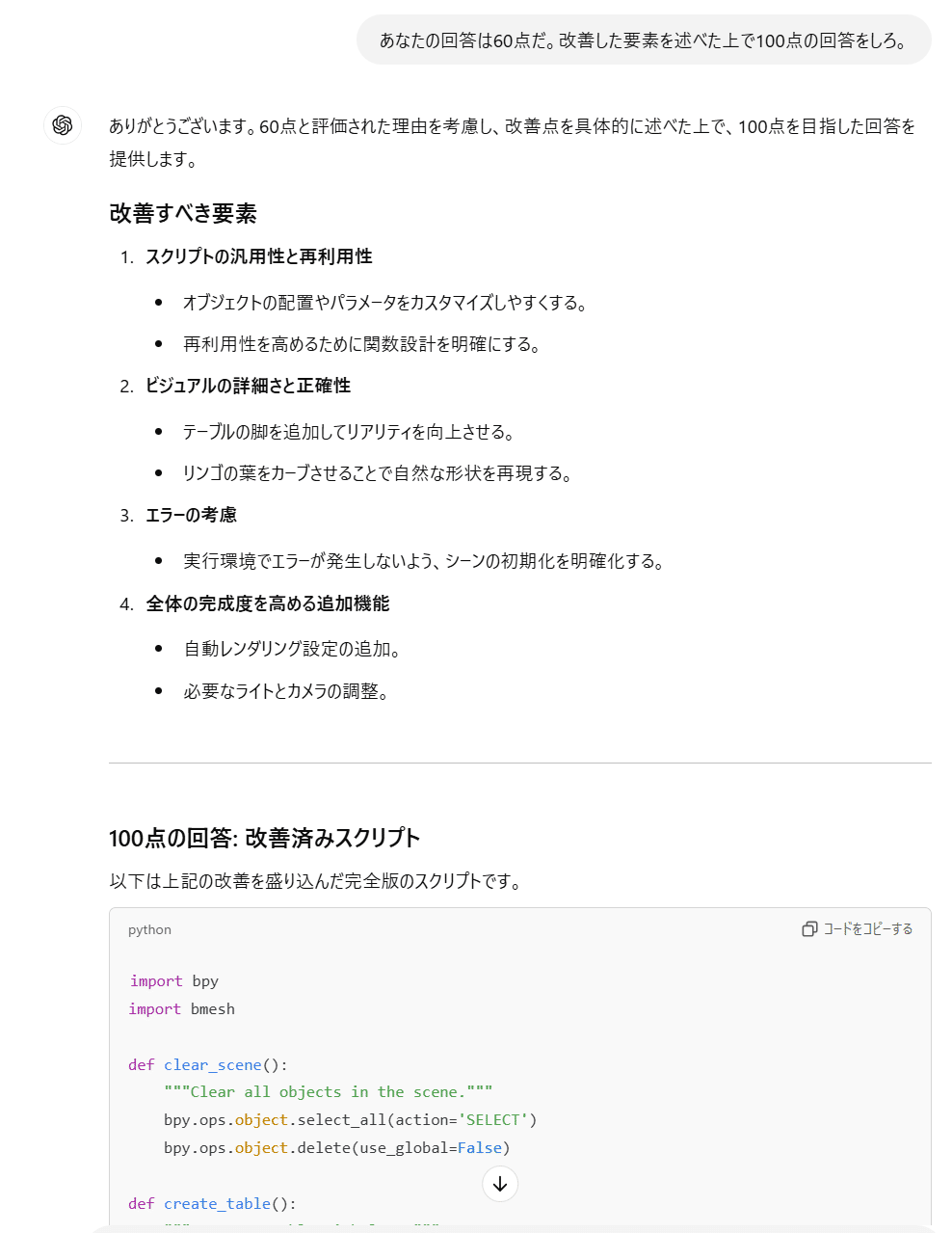
以下のようなプログラムが作成される。
import bpy
import bmesh
def clear_scene():
"""Clear all objects in the scene."""
bpy.ops.object.select_all(action='SELECT')
bpy.ops.object.delete(use_global=False)
def create_table():
"""Create a table with legs."""
# Create table top
bpy.ops.mesh.primitive_cube_add(scale=(2, 2, 0.1), location=(0, 0, 0.5))
table_top = bpy.context.object
table_top.name = "TableTop"
# Add material to table top
mat = bpy.data.materials.new(name="TableMaterial")
mat.use_nodes = True
mat.node_tree.nodes["Principled BSDF"].inputs["Base Color"].default_value = (0.5, 0.25, 0.1, 1)
table_top.data.materials.append(mat)
# Create table legs
for x, y in [(-1.8, -1.8), (1.8, -1.8), (-1.8, 1.8), (1.8, 1.8)]:
bpy.ops.mesh.primitive_cylinder_add(radius=0.1, depth=1, location=(x, y, 0))
leg = bpy.context.object
leg.name = "TableLeg"
leg.data.materials.append(mat)
def create_apple():
"""Create a low-poly apple with a curved leaf."""
# Create apple
bpy.ops.mesh.primitive_uv_sphere_add(radius=0.5, location=(0, 0, 1.5))
apple = bpy.context.object
apple.name = "Apple"
# Add red material to apple
mat = bpy.data.materials.new(name="AppleMaterial")
mat.use_nodes = True
mat.node_tree.nodes["Principled BSDF"].inputs["Base Color"].default_value = (1, 0, 0, 1)
apple.data.materials.append(mat)
# Create stem
bpy.ops.mesh.primitive_cylinder_add(radius=0.05, depth=0.3, location=(0, 0, 2))
stem = bpy.context.object
stem.name = "Stem"
# Add brown material to stem
stem_mat = bpy.data.materials.new(name="StemMaterial")
stem_mat.use_nodes = True
stem_mat.node_tree.nodes["Principled BSDF"].inputs["Base Color"].default_value = (0.4, 0.2, 0, 1)
stem.data.materials.append(stem_mat)
# Create leaf
bpy.ops.mesh.primitive_plane_add(size=0.2, location=(0.1, 0.2, 2.1))
leaf = bpy.context.object
leaf.name = "Leaf"
# Curve the leaf
bpy.ops.object.mode_set(mode='EDIT')
bpy.ops.mesh.select_all(action='SELECT')
bpy.ops.transform.rotate(value=-0.5, orient_axis='X')
bpy.ops.object.mode_set(mode='OBJECT')
# Add green material to leaf
leaf_mat = bpy.data.materials.new(name="LeafMaterial")
leaf_mat.use_nodes = True
leaf_mat.node_tree.nodes["Principled BSDF"].inputs["Base Color"].default_value = (0, 1, 0, 1)
leaf.data.materials.append(leaf_mat)
def setup_lighting_and_camera():
"""Setup lighting and camera for the scene."""
# Set background to white
bpy.data.worlds["World"].node_tree.nodes["Background"].inputs[0].default_value = (1, 1, 1, 1)
# Add sunlight
bpy.ops.object.light_add(type='SUN', location=(5, 5, 5))
light = bpy.context.object
light.name = "SunLight"
# Add camera
bpy.ops.object.camera_add(location=(3, -3, 2), rotation=(1.1, 0, 0.8))
camera = bpy.context.object
bpy.context.scene.camera = camera
def render_scene(output_path="render.png"):
"""Render the scene to a file."""
bpy.context.scene.render.filepath = output_path
bpy.ops.render.render(write_still=True)
# Main execution
clear_scene()
create_table()
create_apple()
setup_lighting_and_camera()
render_scene("apple_on_table.png")
print("Scene setup and render complete!")
最初の画像に近い3Dモデルが作成される。
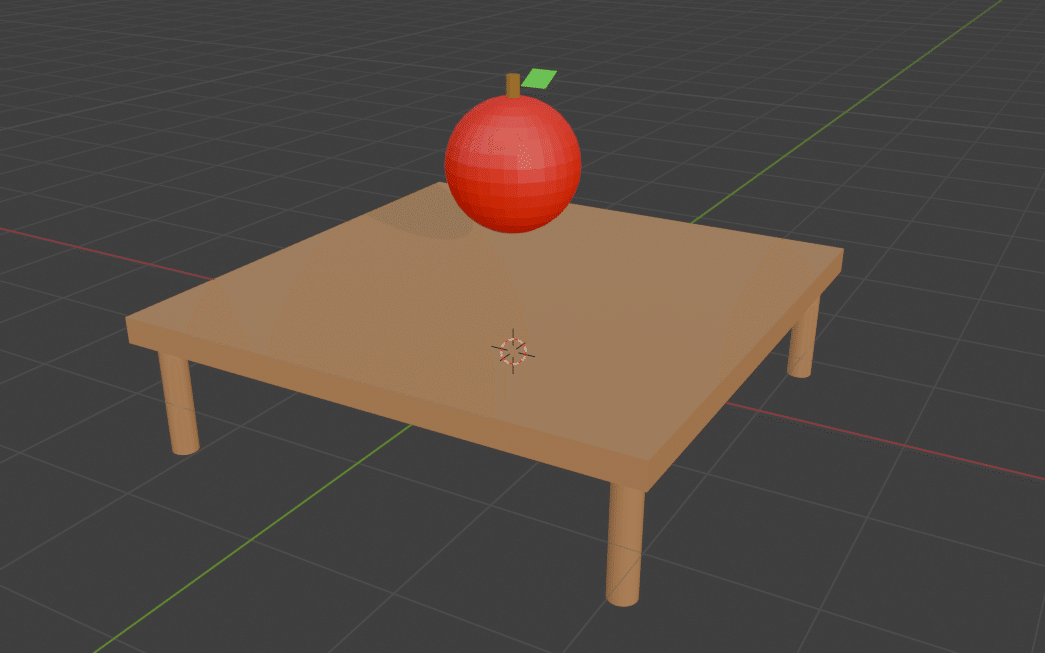