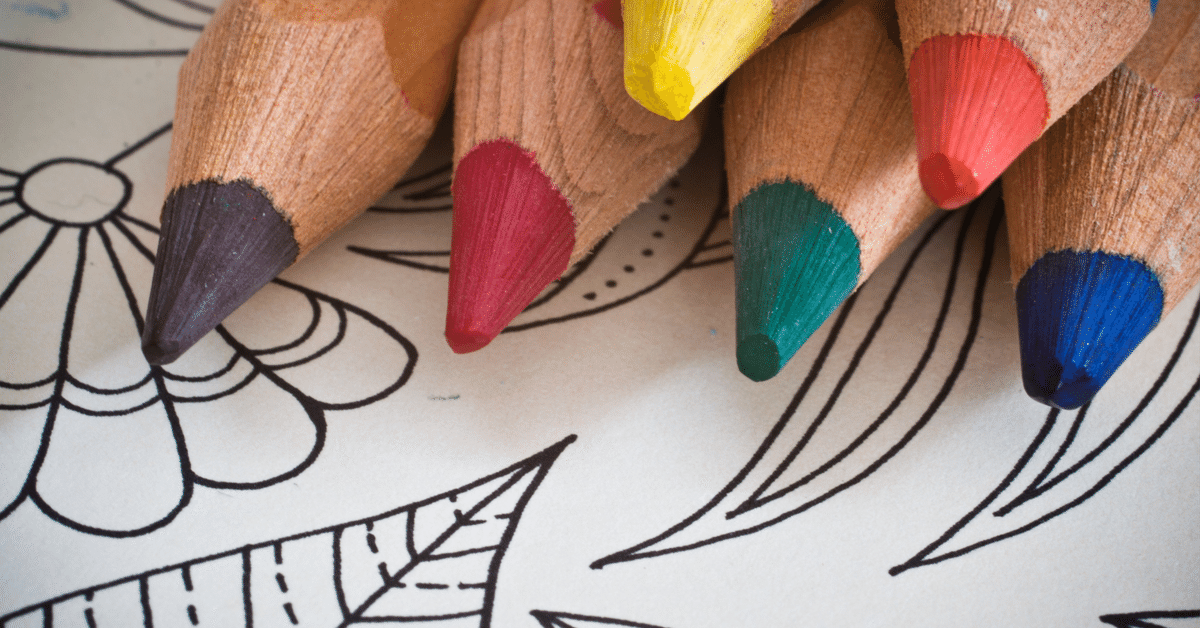
[Python] Googleスプレッドシートのシートタブ色、セルの背景色を設定する
はじめに
Pythonスクリプトを使用して、Googleスプレッドシートのシートタブの色、セルの背景色を設定する方法について説明します。
動作環境
windows11
Jupyter Notebook 6.4.5
Python 3.9.7
手順
1.事前準備
Google Cloud Platformの設定と、使用するGoogleスプレッドシートの共有設定が必要です。設定方法を下記をご参照ください。
2.GoogleスプレッドシートのファイルID、シートIDを取得
ファイルIDとシートIDが、Pythonスクリプトを実装する時に必要となります。
の事前準備の手順で、使用するGoogleスプレッドシートを開くと、ブラウザのURLのアドレスが下記のように表示されています。
https://docs.google.com/spreadsheets/d/xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx/edit#gid=y
この「xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx」の部分がファイルID、「gid=」以下の「y」の部分がシートIDとなります。
後ほど、実装の際に必要になりますので、コピーしてメモっておきます。
3.ライブラリをインストールする
下記のライブラリを使用します。まだインストールされていない場合は、インストールを行ってください。
google-api-python-client
google-auth-httplib2
google-auth-oauthlib
4.Pythonスクリプトを実装する
認証情報の取得
import google.auth
from googleapiclient.discovery import build
from googleapiclient.errors import HttpError
from oauth2client.service_account import ServiceAccountCredentials
scopes = [
'https://www.googleapis.com/auth/spreadsheets',
'https://www.googleapis.com/auth/drive'
]
# 認証情報の取得
credentials = ServiceAccountCredentials.from_json_keyfile_name(
'./service_account_private.json',
scopes=scopes
)
# Google Sheets APIの認証情報の取得
service = build('sheets', 'v4', credentials=credentials)
①OAuthの認証
scopes = [
'<https://www.googleapis.com/auth/spreadsheets>',
'<https://www.googleapis.com/auth/drive>'
]
# 認証情報の取得
credentials = ServiceAccountCredentials.from_json_keyfile_name(
'./service_account_private.json',
scopes=scopes
)
APIで使用する権限を指定します。
今回は、Googleスプレッドシートの権限と、Gogoleドライブ上のファイル操作の権限を指定しています。
②Google Sheets APIの認証情報の取得
# Google Sheets APIの認証情報の取得
service = build('sheets', 'v4', credentials=credentials)
buildメソッドで、Google APIサービスのクライアントオブジェクトを作成することができます。このメソッドには、次の引数を渡します。
service_name:Google APIのサービス名(今回は、Google Sheets APIなので、sheets)
version:Google APIのバージョン(今回は、v4)
credentials:APIサービスにアクセスするための認証情報
③シートタブの色を設定
RGBの設定を、Google Sheets APIに設定可能な辞書型に変換する関数を作成します。
def get_color_dict(origin_color):
color = {
"red": origin_color[0] / 255.0,
"green": origin_color[1] / 255.0,
"blue": origin_color[2] / 255.0
}
return color
続いて、シートタブの色を変更する処理です。今回は、シートタブの色を「赤」に変更します。
SS_ID = 'xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx' # ファイルID
SHEET_ID = 'y' # シートID
# 今回タブの色は赤に設定
tab_color = get_color_dict([255, 0, 0])
#
requests = [
{
"updateSheetProperties": {
"properties": {
"sheetId": SHEET_ID,
"tabColor": tab_color
},
"fields": "tabColor"
}
service.spreadsheets().batchUpdate(spreadsheetId=SS_ID, body={"requests": requests}).execute()
変数:SS_ID、SHEET_IDには、手順2.GoogleスプレッドシートのファイルID、シートIDをそれぞれ設定します。
シートのプロパティを更新する場合、UpdateSheetPropertiesRequestオブジェクトを使用します。
指定するプロパティは、下記です。
fields:更新するプロパティのリストを指定。(今回は、tabColor)
properties:更新するプロパティの値を含むオブジェクトを指定。(今回は、sheetId、tabColor)
作成したリクエストオブジェクトに対して、Googleスプレッドシートの変更を一括で処理するには、service.spreadsheets().batchUpdateメソッドを使用して、リクエストを作成します。
このメソッドには、次の引数を渡します。
spreadsheetId:GoogleスプレッドシートのファイルID
body:リクエストボディ
作成したリクエストを、executeメソッドでHTTPリクエストを実行します。
実行後、シートタブの色が変わったかどうか確認してみます。
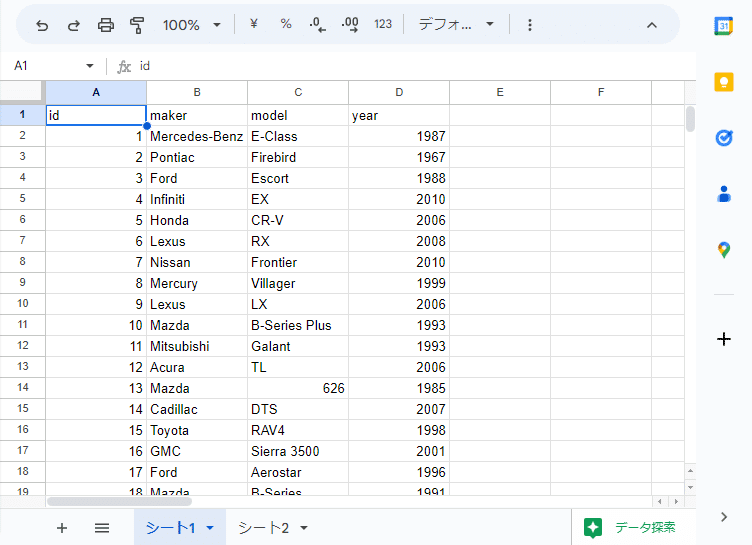
赤に変更されたことが確認できました!
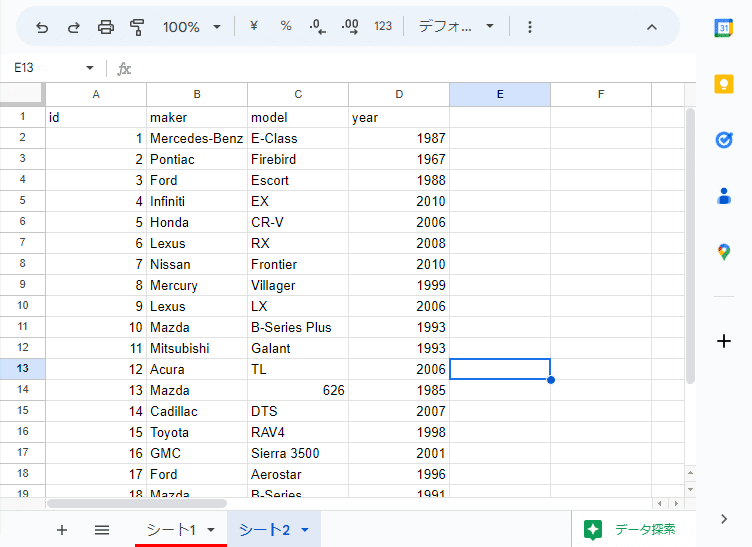
④セルの色を設定
テーブルの見出し行である、A1からD1の範囲のセルの背景色を「グレイ」に設定していきます。
SS_ID = 'xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx' # ファイルID
SHEET_ID = 'y' # シートID
# 色はグレイに設定
color_dict = get_color_dict([204, 204, 204])
#
requests = [
'repeatCell': {
'range': {
'sheetId': SHEET_ID,
'startRowIndex': 0, # 範囲の開始行番号-1
'endRowIndex': 1, # 範囲の終了行番号
'startColumnIndex': 0, # 範囲の開始列番号-1
'endColumnIndex': 4 # 範囲の終了列番号+1
},
'cell': {
'userEnteredFormat': {
'backgroundColor': color_dict
}
},
'fields': 'userEnteredFormat.backgroundColor'
}
}
service.spreadsheets().batchUpdate(spreadsheetId=SS_ID, body={"requests": requests}).execute()
RepeatCellRequestオブジェクトは、指定した範囲内のセルに対して繰り返しパターンを適用するためのリクエストオブジェクトです。
指定するプロパティは、下記です。
range:繰り返しパターンを設定するセル範囲を指定。
sheetIdで、シートを指定します。
セル範囲の指定は、startRowIndex、endRowIndex、startColumnIndex、endColumnIndexで指定を行います。
startRowIndex:範囲の開始行番号から、 -1 した値
endRowIndex:範囲の終了行番号
startColumnIndex:範囲の開始列から、-1 した値
endColumnIndex:範囲の終了列番号+1
今回は、A1からD1セルに対して設定したいので、下記のように設定しました。
'startRowIndex': 0, # 範囲の開始行番号-1
'endRowIndex': 1, # 範囲の終了行番号
'startColumnIndex': 0, # 範囲の開始列番号-1
'endColumnIndex': 4 # 範囲の終了列番号+1
cell:繰り返しパターンの設定を指定。今回は、userEnteredFormatプロパティのbackgroundColorを指定して、背景色を設定します。
properties:更新するプロパティの値を含むオブジェクトを指定。(今回は、userEnteredFormat.backgroundColor)
リクエストを作成したら、先ほどのシートタブの色を設定する処理同様、service.spreadsheets().batchUpdateメソッドを使用して、executeメソッドでHTTPリクエストを実行します。
実行後、A1からD1のセルの背景色が変わったかどうか確認してみます。
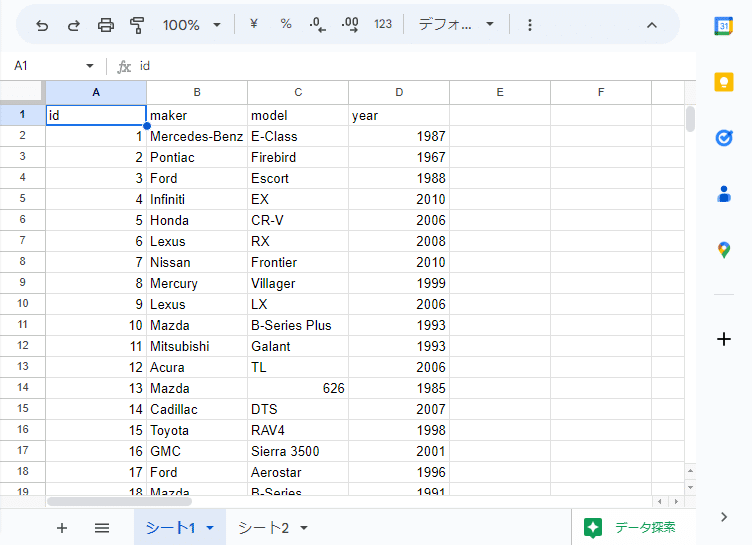
A1からD1セルがグレイに設定されたことが確認できました!
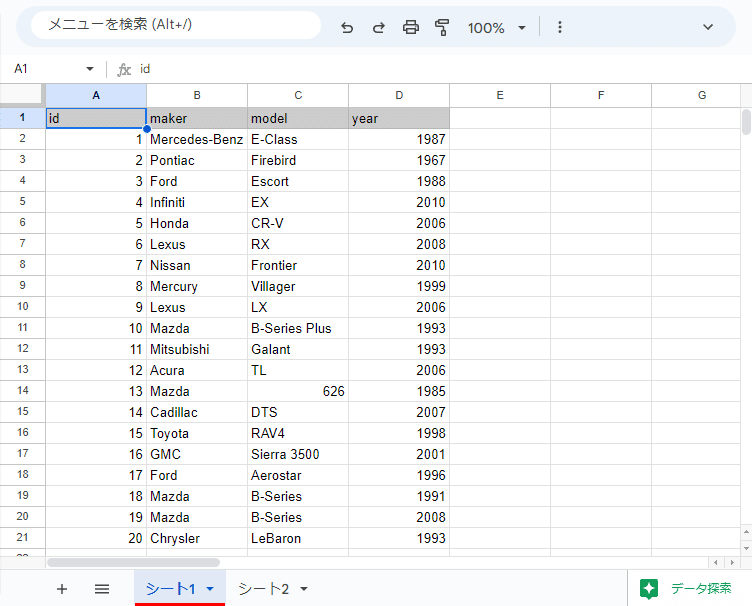
まとめ
今回は、Pythonスクリプトを使用して、Googleスプレッドシートのシートタブの色、セルの背景色を設定する方法についてまとめました。
参考サイト
この記事が気に入ったらサポートをしてみませんか?