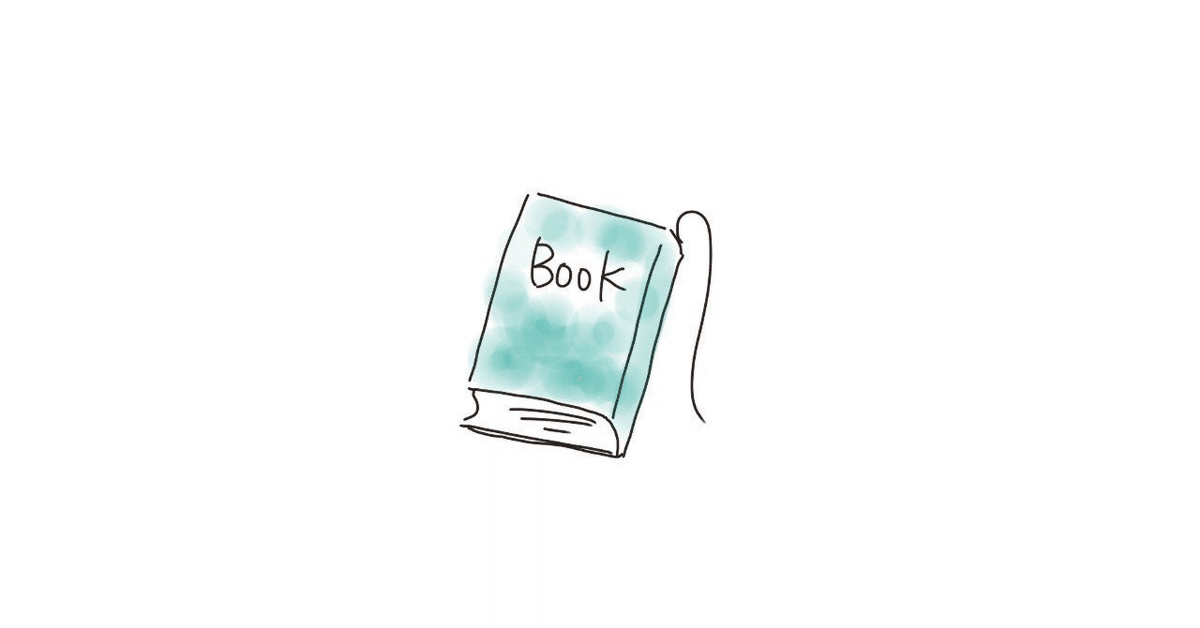
Javascriptについて(3)
プロトタイプとクラス
プロトタイプチェーンの理解
JavaScriptではオブジェクトがプロトタイプチェーンを介して他のオブジェクトを継承します。各オブジェクトには内部プロパティ[[Prototype]]があり、これがチェーンを形成します。
例)
function Person(name) {
this.name = name;
}
Person.prototype.sayHello = function () {
return `Hello, my name is ${this.name}`;
};
const alice = new Person('Alice');
console.log(alice.sayHello()); // "Hello, my name is Alice"
console.log(Object.getPrototypeOf(alice) === Person.prototype); // true
・Object.create()を使用してプロトタイプを明示的に設定。
・プロトタイプチェーンをデバッグするには__proto__やObject.getPrototypeOf()を活用。
ES6クラスと継承
class構文はプロトタイプの扱いを簡素化したもので、extendsを使用すると継承が可能です。
例)
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a noise.`);
}
}
class Dog extends Animal {
speak() {
console.log(`${this.name} barks.`);
}
}
const dog = new Dog('Rex');
dog.speak(); // "Rex barks."
・スーパークラスのメソッドを呼び出す際はsuperを使用。
・staticメソッドでユーティリティ関数を定義。
デザインパターン
シングルトンパターン
シングルトンは特定のクラスのインスタンスが1つしか存在しないことを保証します。
例)
const Singleton = (function () {
let instance;
return {
getInstance() {
if (!instance) {
instance = new Object('I am the instance');
}
return instance;
},
};
})();
const instance1 = Singleton.getInstance();
const instance2 = Singleton.getInstance();
console.log(instance1 === instance2); // true
モジュールパターン
モジュールはデータのカプセル化を行い、外部に公開したいものを明示します。
例)
const Counter = (function () {
let count = 0;
return {
increment() {
count += 1;
return count;
},
getCount() {
return count;
},
};
})();
Counter.increment();
console.log(Counter.getCount()); // 1
Observerパターンの簡単な実装
オブジェクトが他のオブジェクトの状態を監視し、変更があれば通知を受ける仕組み。
例)
class Subject {
constructor() {
this.observers = [];
}
subscribe(observer) {
this.observers.push(observer);
}
unsubscribe(observer) {
this.observers = this.observers.filter(obs => obs !== observer);
}
notify(data) {
this.observers.forEach(observer => observer.update(data));
}
}
class Observer {
update(data) {
console.log(`Observer received data: ${data}`);
}
}
const subject = new Subject();
const observer1 = new Observer();
subject.subscribe(observer1);
subject.notify('Hello, World!'); // Observer received data: Hello, World!
パフォーマンス最適化
デバウンスとスロットリング
・デバウンス: 一定時間イベントが発生しなくなるまで実行を遅延。
・スロットリング: 一定間隔でイベントを実行。
例)
const debounce = (func, delay) => {
let timeout;
return (...args) => {
clearTimeout(timeout);
timeout = setTimeout(() => func(...args), delay);
};
};
const throttle = (func, limit) => {
let inThrottle;
return (...args) => {
if (!inThrottle) {
func(...args);
inThrottle = true;
setTimeout(() => (inThrottle = false), limit);
}
};
};
メモ化の導入
計算結果をキャッシュして再計算を防ぐ。
例)
const memoize = (fn) => {
const cache = {};
return (...args) => {
const key = JSON.stringify(args);
if (cache[key]) {
return cache[key];
}
const result = fn(...args);
cache[key] = result;
return result;
};
};
const slowFunction = (num) => {
console.log('Calculating...');
return num * 2;
};
const fastFunction = memoize(slowFunction);
console.log(fastFunction(10)); // Calculating... 20
console.log(fastFunction(10)); // 20 (cached)
非同期処理
Web Workers
Web Workersはスクリプトをバックグラウンドで実行し、メインスレッドの負荷を軽減します。
例)
// worker.js
onmessage = function (e) {
const result = e.data * 2;
postMessage(result);
};
// main.js
const worker = new Worker('worker.js');
worker.onmessage = function (e) {
console.log('Result:', e.data);
};
worker.postMessage(10);
RxJSの基本
RxJSはリアクティブプログラミングを実現するライブラリ。
例)
import { fromEvent } from 'rxjs';
import { throttleTime } from 'rxjs/operators';
const button = document.getElementById('myButton');
fromEvent(button, 'click')
.pipe(throttleTime(1000))
.subscribe(() => console.log('Button clicked!'));
TypeScriptへの移行
型注釈の基本
例)
let count: number = 10;
let name: string = 'Alice';
let isActive: boolean = true;
function add(a: number, b: number): number {
return a + b;
}
JavaScriptプロジェクトにTypeScriptを導入する手順
1.TypeScriptのインストール
npm install --save-dev typescript
2.tsconfig.jsonを作成
npx tsc --init
3.既存のJavaScriptファイルを.tsまたは.tsxに変換。
4.型チェックとエラー修正。
5.コンパイルとテストの実行
npx tsc
まとめ
一つ一つずつ理解を深めていくと、よりよいコーディングができそうですね。
今回はここまでとなります。最後まで閲覧いただきありがとうございます。