#15 YouTubeの動画情報を自動取得!Pythonでチャンネルの動画データをExcelに保存する方法
1.はじめに
YouTubeで特定のチャンネルの動画情報を一覧で取得したいと思ったことはありませんか? 手作業で動画のタイトルや再生回数を確認するのは手間がかかりますし、大量のデータを扱うのは現実的ではありません。
この記事では、YouTube Data APIを活用して、指定したYouTubeチャンネルの動画情報を取得し、Excelに自動保存するPythonスクリプトを紹介します。
このスクリプトを使うことで、
指定したチャンネルの動画情報(タイトル、投稿日、再生時間、再生回数、URL)を自動で取得
最新の動画データを簡単にExcelファイルに保存
データを集計・分析することで、チャンネルの成長や傾向を確認可能
といったことができるようになります。
2.必要なモジュールのインストール
このスクリプトでは、以下のPythonモジュールを使用しています。インストールされていない場合は、以下のコマンドを実行してインストールしてください。
pip install requests pandas isodate openpyxl
3.YouTube APIキーの取得と設定
このスクリプトは YouTube Data API v3 を使用するため、Google Cloud Console でAPIキーを取得する必要があります。
手順は、こちらの記事をご参考下さい。
APIキーの環境変数設定
スクリプトは os.getenv("YOUTUBE_API_KEY") で環境変数からAPIキーを取得するため、以下のように環境変数を設定してください。
set YOUTUBE_API_KEY=”あなたのAPIキーをここに貼り付ける”
これで、環境変数に"YOUTUBE_API_KEY"が設定されます。
このコマンドは、最初のみ実行します。
4.Pythonコードの実行
APIキーを設定したら、Pythonコードを実行します。
※コードは最後に記述しています。
Python YoutubeVideoScraper_Excel.py
Pythonコードが実行されると、YouTubeのチャンネルURLを入力するよう求められます。
YouTubeチャンネルのURLを入力してください:
YouTubeチャンネルのURLを入力してください:
ここで、調べたいYouTubeチャンネルのURL(例: https://www.youtube.com/@XXXXXXX)を入力すると、動画情報を取得し、Excelファイル (.xlsx) に保存します。
5.実行結果
Pythonコードが保存されているフォルダにExcelファイルが保存されます。
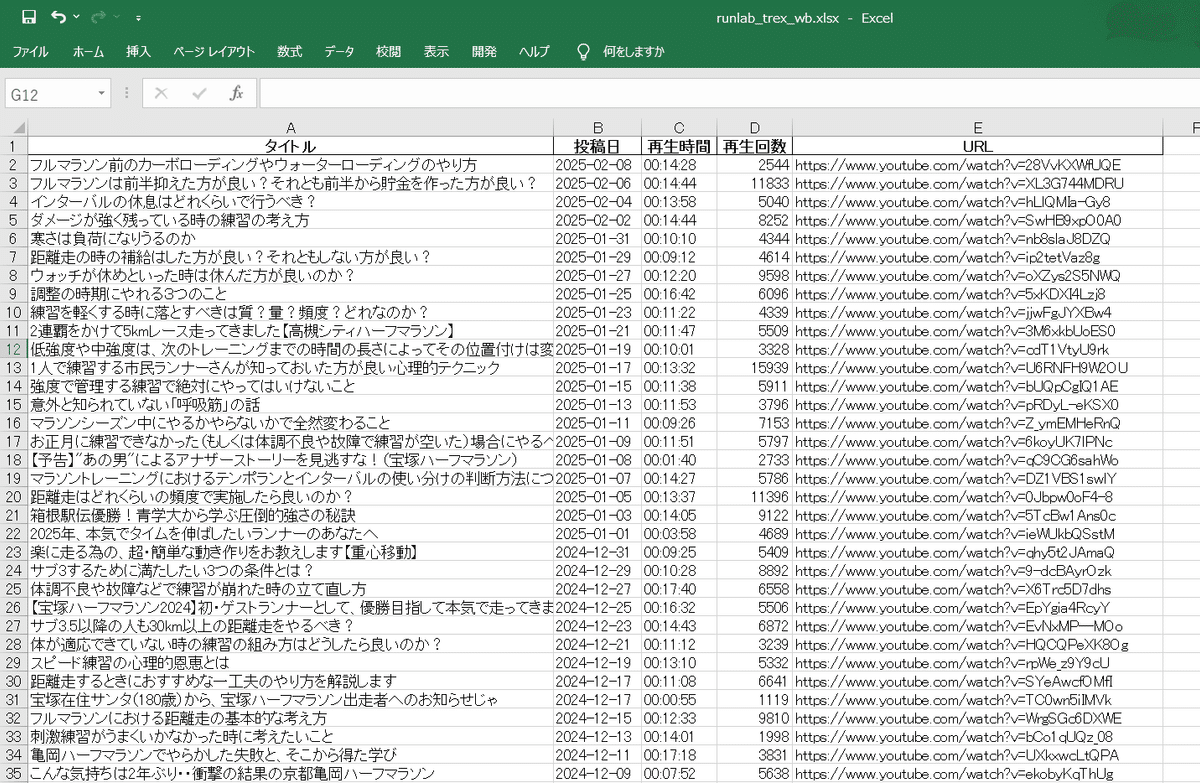
6.おわりに
YouTube Data APIを活用することで、チャンネルの動画情報を手間なく取得し、データ分析やマーケティングに活用することができます。
特に以下のような方におすすめです。
YouTubeチャンネルの分析をしたい人
YouTubeの動画情報を整理・管理したい人
YouTubeのデータをビジネスやリサーチに活用したい人
Pythonを活用することで、手作業では難しい大量のデータ取得や自動化が可能になります。
以下、今回実行したPythonコードです。
Pythonコード
import requests
import re
import pandas as pd
import os
import isodate
# 環境変数からAPIキーを取得
API_KEY = os.getenv("YOUTUBE_API_KEY")
if not API_KEY:
raise ValueError("環境変数 'YOUTUBE_API_KEY' が設定されていません。")
def get_channel_id(channel_url):
"""チャンネルのURLからチャンネルIDを取得"""
handle_match = re.search(r"youtube\.com/@([\w-]+)", channel_url)
if handle_match:
handle = handle_match.group(1)
url = f"https://www.googleapis.com/youtube/v3/channels?part=id&forHandle=@{handle}&key={API_KEY}"
else:
username_match = re.search(r"youtube\.com/(?:c/|user/)([\w-]+)", channel_url)
if username_match:
username = username_match.group(1)
url = f"https://www.googleapis.com/youtube/v3/channels?part=id&forUsername={username}&key={API_KEY}"
else:
channel_id_match = re.search(r"youtube\.com/channel/([\w-]+)", channel_url)
if channel_id_match:
return channel_id_match.group(1)
else:
print("無効なURLです。")
return None
response = requests.get(url).json()
if "items" in response and response["items"]:
return response["items"][0]["id"]
else:
print("チャンネルIDを取得できませんでした。")
return None
def format_duration(duration):
"""ISO 8601形式の再生時間を hh:mm:ss 形式に変換"""
try:
duration_sec = int(isodate.parse_duration(duration).total_seconds())
hours, remainder = divmod(duration_sec, 3600)
minutes, seconds = divmod(remainder, 60)
return f"{hours:02}:{minutes:02}:{seconds:02}"
except:
return "00:00:00"
def get_video_details(video_id):
"""動画の詳細情報を取得"""
url = f"https://www.googleapis.com/youtube/v3/videos?part=snippet,contentDetails,statistics&id={video_id}&key={API_KEY}"
response = requests.get(url).json()
if "items" in response and response["items"]:
item = response["items"][0]
title = item["snippet"]["title"]
published_date = item["snippet"]["publishedAt"][:10] # YYYY-MM-DD形式
duration = format_duration(item["contentDetails"]["duration"]) # hh:mm:ss 形式
view_count = item["statistics"].get("viewCount", "0")
return title, published_date, duration, int(view_count)
return None, None, None, None
def get_video_data(channel_id):
"""チャンネルの動画データを取得"""
url = f"https://www.googleapis.com/youtube/v3/search?key={API_KEY}&channelId={channel_id}&part=id&order=date&maxResults=50"
videos = []
while url:
response = requests.get(url).json()
for item in response.get("items", []):
if "videoId" in item["id"]:
video_id = item["id"]["videoId"]
title, published_date, duration, view_count = get_video_details(video_id)
if title:
videos.append([title, published_date, duration, view_count, f"https://www.youtube.com/watch?v={video_id}"])
next_page_token = response.get("nextPageToken")
if next_page_token:
url = f"https://www.googleapis.com/youtube/v3/search?key={API_KEY}&channelId={channel_id}&part=id&order=date&maxResults=50&pageToken={next_page_token}"
else:
url = None
return videos
def main():
channel_url = input("YouTubeチャンネルのURLを入力してください: ")
channel_id = get_channel_id(channel_url)
if channel_id:
video_data = get_video_data(channel_id)
if video_data:
df = pd.DataFrame(video_data, columns=["タイトル", "投稿日", "再生時間", "再生回数", "URL"])
handle_match = re.search(r"youtube\.com/@([\w-]+)", channel_url)
if handle_match:
filename = f"{handle_match.group(1)}.xlsx"
else:
filename = "youtube_videos.xlsx"
df.to_excel(filename, index=False)
print(f"取得した動画データを {filename} に保存しました。")
else:
print("動画が見つかりませんでした。")
if __name__ == "__main__":
main()
おわり。