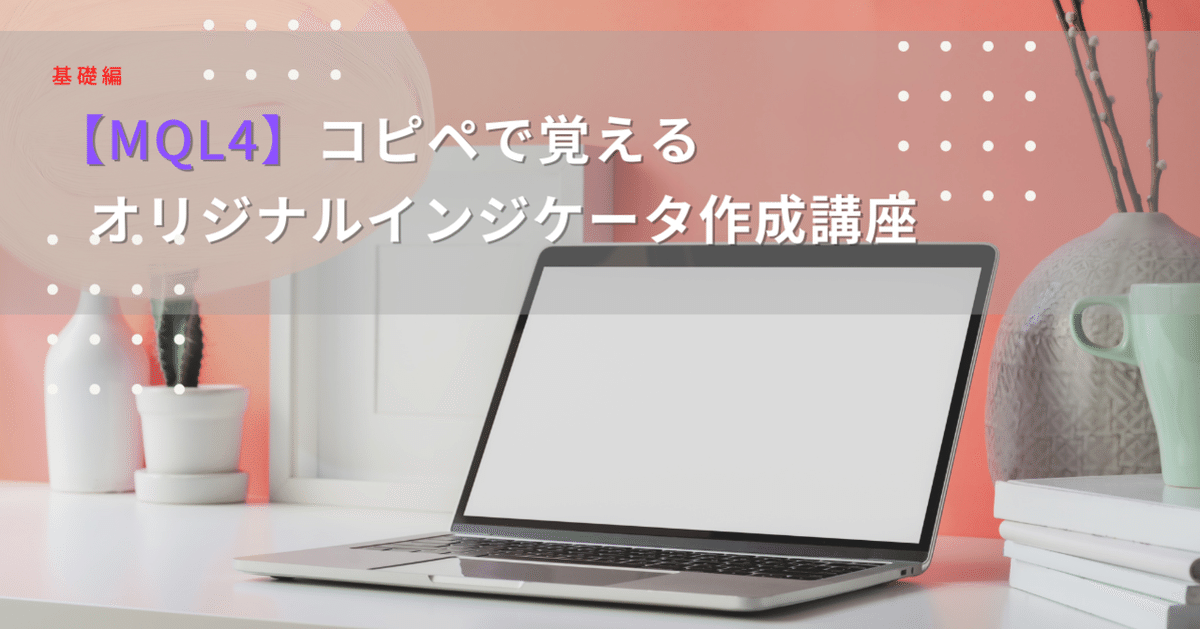
【MQL4】コピペで覚えるオリジナルインジケータ作成講座#02(ボリンジャーバンドと矢印を表示させる)
コピペで覚えるオリジナルインジケータ作成講座。
この作成講座では、コピペでMQL4のコードの書き方を覚えてもらい、オリジナルのインジケータを自分で作成できるようにしてもらうことを目的としています。
実際に私が個人で開発したものをサンプルコードとして載せています。初回のみ無料記事にしていますが、商品として出したものをサンプルコードとして載せていくので、2回目以降は有料記事とさせてください。
今回は「ボリンジャーバンド」を使ったインジケータを作ってみます。「ボリンジャーバンド」はよく逆張りにも使用される手法の一つですね。
それに追加でバンドからはみ出たところに「矢印」が出現するようにしてみます。
完成イメージ
サンプルコードは以下の通りです。
今回のサンプルコードを書けることで、応用して矢印の出し方を自分で作ることができるようになります。
サンプルコード
//+------------------------------------------------------------------+
//| bb_sign.mq4 |
//| Copyright 2022, システム開発屋じゅにこ. |
//| https://note.com/junico02_se_blog/n/nce82a50948f7 |
//+------------------------------------------------------------------+
#property copyright "Copyright 2022, システム開発屋じゅにこ."
#property link "https://note.com/junico02_se_blog/n/nce82a50948f7"
#property version "1.00"
#property strict
#property indicator_chart_window
// インジケータプロパティ設定
#property indicator_buffers 5 // 使用するバッファ: 5
#property indicator_color1 clrSpringGreen // ボリンジャーバンド1の色: SpringGreen
#property indicator_width1 1 // ボリンジャーバンド1の太さ: 1
#property indicator_type1 DRAW_LINE // チャート内に描写するオブジェクトの種類: ライン
#property indicator_color2 clrSpringGreen // ボリンジャーバンド2の色: SpringGreen
#property indicator_width2 1 // ボリンジャーバンド2の太さ: 1
#property indicator_type2 DRAW_LINE // チャート内に描写するオブジェクトの種類: ライン
#property indicator_color3 clrSpringGreen // ボリンジャーバンド3の色: SpringGreen
#property indicator_width3 1 // ボリンジャーバンド3の太さ: 1
#property indicator_type3 DRAW_LINE // チャート内に描写するオブジェクトの種類: ライン
#property indicator_color4 clrLightBlue // サイン1の色の色: LightBlue
#property indicator_width4 1 // サイン1の太さ: 1
#property indicator_type4 DRAW_ARROW // チャート内に描写するオブジェクトの種類: 矢印
#property indicator_color5 clrLightPink // サイン2の色の色: LightPink
#property indicator_width5 1 // サイン2の太さ: 1
#property indicator_type5 DRAW_ARROW // チャート内に描写するオブジェクトの種類: 矢印
// インジケータ表示用動的配列
double _IndBuffer1[]; // インジケータ1(ボリンジャーバンド1)表示用動的配列
double _IndBuffer2[]; // インジケータ2(ボリンジャーバンド2)表示用動的配列
double _IndBuffer3[]; // インジケータ3(ボリンジャーバンド3)表示用動的配列
// サイン表示用動的配列
double BB_sign_up[]; // インジケータ4(サイン1)表示用動的配列
double BB_sign_down[]; // インジケータ5(サイン2)表示用動的配列
//パラメータ設定用
extern int BB_Period = 20; //■平均期間
extern int BB_deviation = 2; //■標準偏差
int OnInit()
{
SetIndexBuffer( 0, _IndBuffer1 ); // インジケータ1表示用動的配列をインジケータ1として登録。
SetIndexBuffer( 1, _IndBuffer2 ); // インジケータ2表示用動的配列をインジケータ2として登録。
SetIndexBuffer( 2, _IndBuffer3 ); // インジケータ3表示用動的配列をインジケータ3として登録。
SetIndexBuffer( 3, BB_sign_up ); // インジケータ4表示用動的配列をインジケータ4として登録。
SetIndexBuffer( 4, BB_sign_down ); // インジケータ5表示用動的配列をインジケータ5として登録。
SetIndexArrow( 3, 233 ); // 上矢印
SetIndexArrow( 4, 234 ); // 下矢印
return( INIT_SUCCEEDED ); // 戻り値:初期化成功
}
int OnCalculate(const int rates_total, // 入力された時系列のバー数
const int prev_calculated, // 計算済み(前回呼び出し時)のバー数
const datetime &time[], // 時間
const double &open[], // 始値
const double &high[], // 高値
const double &low[], // 安値
const double &close[], // 終値
const long &tick_volume[], // Tick出来高
const long &volume[], // Real出来高
const int &spread[]) // スプレッド
{
int end_index = Bars - prev_calculated; // バー数取得(未計算分)
for( int icount = 0 ; icount < end_index ; icount++ ) {
// テクニカルインジケータ算出
double result1 = iBands(
NULL, // 通貨ペア
0, // 時間軸
20, // 平均期間
2, // 標準偏差
0, // バンドシフト
PRICE_CLOSE, // 適用価格
MODE_MAIN, // ラインインデックス
icount // シフト
);
double result2 = iBands(
NULL, // 通貨ペア
0, // 時間軸
20, // 平均期間
2, // 標準偏差
0, // バンドシフト
PRICE_CLOSE, // 適用価格
MODE_UPPER, // ラインインデックス
icount // シフト
);
double result3 = iBands(
NULL, // 通貨ペア
0, // 時間軸
20, // 平均期間
2, // 標準偏差
0, // バンドシフト
PRICE_CLOSE, // 適用価格
MODE_LOWER, // ラインインデックス
icount // シフト
);
_IndBuffer1[icount] = result1; // インジケータ1に算出結果を設定
_IndBuffer2[icount] = result2; // インジケータ2に算出結果を設定
_IndBuffer3[icount] = result3; // インジケータ3に算出結果を設定
if( close[icount] < _IndBuffer3[icount] ){
BB_sign_up[icount] = low[icount] - Point * 10; // インジケータ4に算出結果を設定
}else if( close[icount] > _IndBuffer2[icount] ){
BB_sign_down[icount] = high[icount] + Point * 10; // インジケータ5に算出結果を設定
}
}
return( rates_total );
}
サンプルコード解説
#property indicator_chart_window
⇨ この設定でチャート画面に表示させます。
#property indicator_buffers 5
⇨ 今回、表示させるものは全部で5つです。
#property indicator_color1 clrSpringGreen
#property indicator_width1 1
#property indicator_type1 DRAW_LINE
⇨ ボリンジャーバンドの上のライン
#property indicator_color2 clrSpringGreen
#property indicator_width2 1
#property indicator_type2 DRAW_LINE
⇨ ボリンジャーバンドの中央のライン
#property indicator_color3 clrSpringGreen
#property indicator_width3 1
#property indicator_type3 DRAW_LINE
⇨ ボリンジャーバンドの下のライン
#property indicator_color4 clrLightBlue
#property indicator_width4 1
#property indicator_type4 DRAW_ARROW
⇨ 上矢印
#property indicator_color5 clrLightPink
#property indicator_width5 1
#property indicator_type5 DRAW_ARROW
⇨ 下矢印
double result1~3 = iBands(NULL,0,20,2,0,PRICE_CLOSE,XXXXX,icount);
⇨ 終値を基準に対してボリンジャーバンドの上、中央、下のラインを計算して、それぞれを変数に格納。
//iBandsのパラメータについては以下の通り。
double iBands(
string symbol, // 通貨ペア
int timeframe, // 時間軸
int period, // 平均期間
double deviation, // 標準偏差
int bands_shift, // バンドシフト
int applied_price, // 適用価格
int mode, // ラインインデックス
int shift // シフト
);
//(period:20、deviation:2)
// ↑のパラメータに関しては、上部で↓の様に設定している。
//extern int BB_Period = 20; //■平均期間
//extern int BB_deviation = 2; //■標準偏差
_IndBuffer1[icount] = BB_upper; // インジケータ1に算出結果を設定
_IndBuffer2[icount] = BB_main; // インジケータ2に算出結果を設定
_IndBuffer3[icount] = BB_lower; // インジケータ3に算出結果を設定
⇨ 格納されたそれぞれの変数を各インジケータに格納(ここでラインの描画が行われる)。
if(close[icount] < _IndBuffer3[icount]){
BB_sign_up[icount] = low[icount] - Point * 10;
}
⇨ 終値がボリンジャーバンドの上だった場合に、上矢印を描写。
(矢印の表示位置を安値 ー(1point分の値幅)*10)
else if(close[icount] > _IndBuffer2[icount]){
BB_sign_down[icount] = high[icount] + Point * 10;
}
⇨ 終値がボリンジャーバンドの上だった場合に、下矢印を描写。
(矢印の表示位置を高値 +(1point分の値幅)*10)