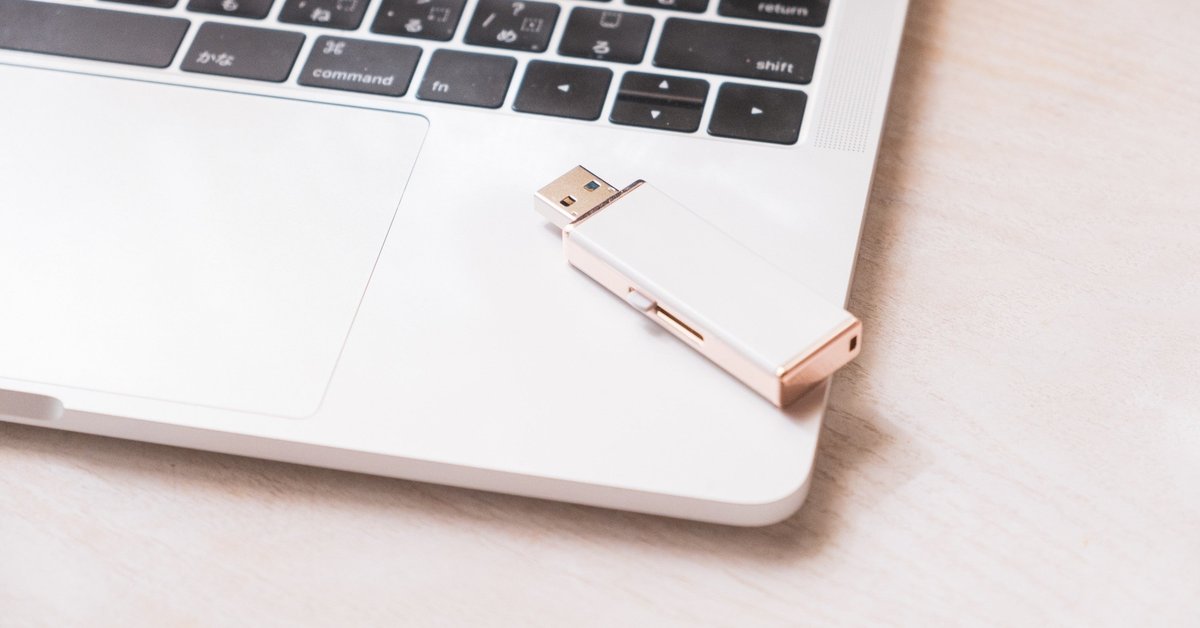
(6)Railsで簡単なメモアプリを作ってみる
今回はSCSSの@mixinで同じような記述をまとめていきます。
また、修飾もしていきます。
以前まとめた記事 → SCSSのmixinが優秀すぎたのでまとめます。
mixinの設定
app/assets/stylesheets/memo.scss
.notification {
.notice {
background-color:blue;
color: white;
text-align: center;
}
.alert {
background-color: orange;
color: white;
text-align: center;
}
.delete {
background-color: red;
color: white;
text-align: center;
}
}
まずはmixin.scssファイルを作成します。
../stylesheets/mixin.scss
@mixin flash($color) {
background-color: $color;
color: white;
text-align: center;
}
背景色のみ違うので引数に$colorを設定し、includeした時に
色指定をします。
../stylesheets/memo.scss
.notification {
.notice {
@include flash(blue);
}
.alert {
@include flash(orange);
}
.delete {
@include flash(red);
}
}
最後にapplication.cssをapplication.scssに変更します。
../stylesheets/application.scss
@import "mixin.scss";
@import "memo.scss";
applicationファイルには元々全てのscssファイルを読み込む記述が書かれていますが、予期せぬファイル読み込みを防ぐためにも
コメントアウトを全て消して、上記の2行を書き込みます。
ちなみに、リンク先があると下線が表示されるのでわかりやすいです。
以前と変わらない表示なら問題なくmixinが設定できています。
scssで修飾
まずはreset.scssを作成します。
../stylesheets/_reset.scss
/*!
* YUI 3.5.0 - reset.css (http://developer.yahoo.com/yui/3/cssreset/)
* http://cssreset.com
* Copyright 2012 Yahoo! Inc. All rights reserved.
* http://yuilibrary.com/license/
*/
/*
TODO will need to remove settings on HTML since we can't namespace it.
TODO with the prefix, should I group by selector or property for weight savings?
*/
html{
color:#000;
background:#FFF;
}
/*
TODO remove settings on BODY since we can't namespace it.
*/
/*
TODO test putting a class on HEAD.
- Fails on FF.
*/
body,
div,
dl,
dt,
dd,
ul,
ol,
li,
h1,
h2,
h3,
h4,
h5,
h6,
pre,
code,
form,
fieldset,
legend,
input,
textarea,
p,
blockquote,
th,
td {
margin:0;
padding:0;
}
table {
border-collapse:collapse;
border-spacing:0;
}
fieldset,
img {
border:0;
}
/*
TODO think about hanlding inheritence differently, maybe letting IE6 fail a bit...
*/
address,
caption,
cite,
code,
dfn,
em,
strong,
th,
var {
font-style:normal;
font-weight:normal;
}
ol,
ul {
list-style:none;
}
caption,
th {
text-align:left;
}
h1,
h2,
h3,
h4,
h5,
h6 {
font-size:100%;
font-weight:normal;
}
q:before,
q:after {
content:'';
}
abbr,
acronym {
border:0;
font-variant:normal;
}
/* to preserve line-height and selector appearance */
sup {
vertical-align:text-top;
}
sub {
vertical-align:text-bottom;
}
input,
textarea,
select {
font-family:inherit;
font-size:inherit;
font-weight:inherit;
}
/*to enable resizing for IE*/
input,
textarea,
select {
*font-size:100%;
}
/*because legend doesn't inherit in IE */
legend {
color:#000;
}
/* YUI CSS Detection Stamp */
#yui3-css-stamp.cssreset { display: none; }
app/assetsフォルダ内にmemoフォルダを作成します。
その中にindex.scssファイルを作成
../stylesheets/memo/index.scss
body {
margin: 0;
padding: 0;
}
a {
text-decoration: none;
color: black;
}
.header {
display: inline-flex;
justify-content: space-evenly;
height: 50px;
width: 100%;
line-height: 50px;
background-color: lavender;
a:hover {
color: red;
}
}
.messages {
margin: 20px auto;
max-width: 500px;
padding: 10px;
text-align: center;
height: 300px;
background-color: aliceblue;
overflow: scroll;
.content {
margin: 15px auto;
width: 80%;
height: 40px;
}
.message {
line-height: 40px;
border: 1px solid lightgray;
display: flex;
justify-content: space-between;
padding: 0 10px;
overflow: auto;
.btn {
font-size: 12px;
line-height: 20px;
.edit-btn:hover {
color: blue;
}
.delete-btn:hover {
color: red;
}
}
}
}
../stylesheets/application.scss
@import "reset";
@import "mixin.scss";
@import "memo.scss";
@import "memos/*";
reset.scssとmemos/*を追加して反映完了。
memos/*はmemosフォルダ内の全てのファイルを読み込むという記述になります。
../views/memo/index.html.erb
<div class="header">
<%= link_to 'Memo app', root_path %>
<%= link_to '新規投稿', new_memo_path %>
</div>
<div class="messages">
<% @posts.each do |post| %>
<ul class="content">
<li class="message">
<%= post.content %>
<ul>
<li class="btn">
<%= link_to "編集", edit_memo_path(post), class: "edit-btn" %>
</li>
<li class="btn">
<%= link_to "削除", memo_path(post.id), class: "delete-btn", method: :delete %>
</li>
</ul>
</li>
</ul>
<% end %>
</div>
こんな感じになっていればビューは完成です。
メッセージの文字数制限(validate)
メモアプリなので短文投稿に指定したいと思います。
../memo/new.html.erbとedit.html.erb
<input type="text" name="content" maxlength='20'>
これで入力フォームには20文字以上入力することはできません。
以上で簡単なメモアプリは完成となります。
入力、編集フォームの修飾をしていませんが、こちらはトップページと同じような記述を行えばいいので割愛します。
初めてこのようなチュートリアルの真似事をしてみましたが、思った以上に大変ですね(汗
ですがその分学びも多いので、続けていきたいと思います。
ここまで見ていただきありがとうございました。
おかしな記述があれば教えていただけるとありがたいです。