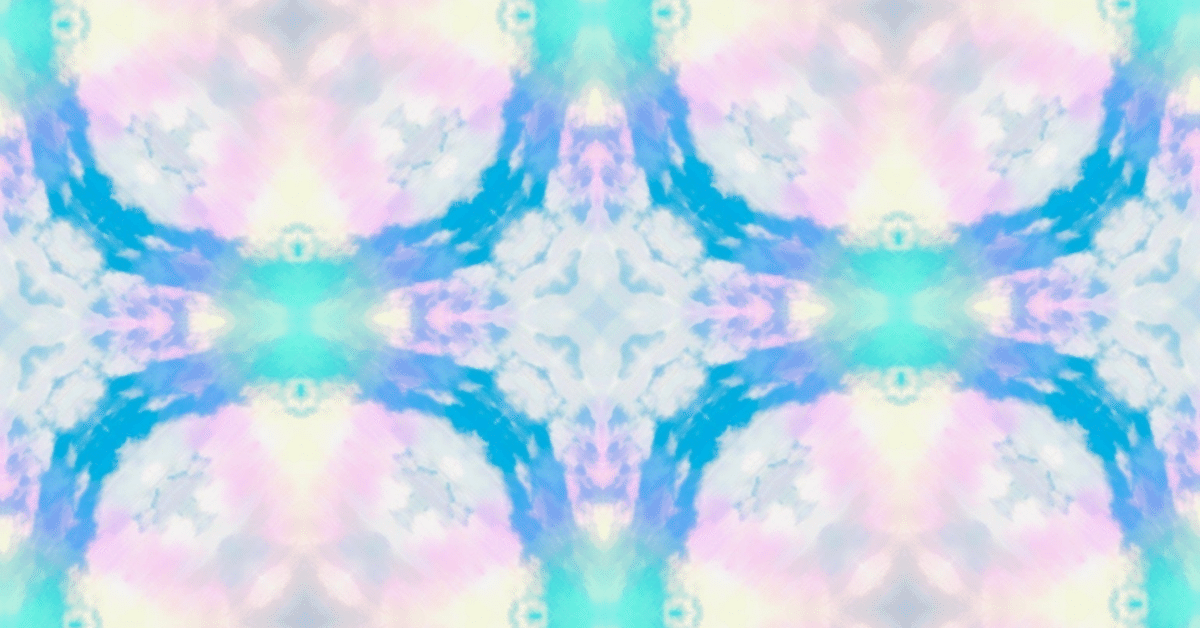
วันนี้ลองใช้ Chat GPT ช่วยคิดแก้ปัญหาการโยนลูกเต๋า ปรากฏว่า เก่งมาก
วันนี้ลองใช้ Chat GPT ช่วยคิดแก้ปัญหาการโยนลูกเต๋า ปรากฏว่า เก่งมาก คำนวณก็ได้ ให้เขียนโค๊ด python ให้ก็ได้ สุดยอดจริงๆ และได้รู้ว่า การอ่านโค๊ด python ที่ Chat GPT เขียนให้ เป็นการเรียนการเขียนโค๊ดที่ดี ได้ด้วย... น่าทึ่งครับ ต้องช่วยกันใช้ในทางที่ถูกต้อง จะช่วยการเรียนรู้และการทำงานได้มาก และต้องระวังด้วยว่า บางที Chat GPTก็ผิดได้ด้วย ในกรณีที่เข้าใจคำขอของเราผิด
เริ่มจากง่ายๆ ก่อน
ให้เขียนโปรแกรม หาค่าความน่าจะเป็น ของการโยนลูกเต๋าสองครั้งแล้วได้ค่าเหมือนกัน
Probability of rolling the same number 2 times in a row (answer= 1/6)
import random
def roll_dice():
return random.randint(1, 6)
def rolls_same_number():
rolls = [roll_dice() for i in range(2)]
return all(r == rolls[0] for r in rolls)
def probability(n):
count = 0
for i in range(n):
if rolls_same_number():
count += 1
return count / n
print("Probability of rolling the same number 2 times in a row:", probability(100000))
Probability of rolling the same number 4 times in a row: 0.16456
ให้เขียนโปรแกรม หาค่าความน่าจะเป็น ของการโยนลูกเต๋าสองครั้งแล้วได้ค่าไม่เหมือนกัน
Probability of rolling different numbers 2 times in a row (answer= 5/6)
import random
def roll_dice():
return random.randint(1, 6)
def rolls_different_numbers():
rolls = [roll_dice() for i in range(2)]
return len(set(rolls)) == 2
def probability(n):
count = 0
for i in range(n):
if rolls_different_numbers():
count += 1
return count / n
print("Probability of rolling different numbers 2 times in a row:", probability(100000))
Probability of rolling different numbers 4 times in a row: 0.83357
ให้เขียนโปรแกรม หาค่าความน่าจะเป็น ของการโยนลูกเต๋า 4 ครั้งแล้วได้ค่าเหมือนกัน
Probability of rolling the same number 4 times in a row (answer= 1/(6 x 6 x 6)
import random
def roll_dice():
return random.randint(1, 6)
def rolls_same_number():
rolls = [roll_dice() for i in range(4)]
return all(r == rolls[0] for r in rolls)
def probability(n):
count = 0
for i in range(n):
if rolls_same_number():
count += 1
return count / n
print("Probability of rolling the same number 4 times in a row:", probability(100000))
Probability of rolling the same number 4 times in a row: 0.00462
ให้เขียนโปรแกรม หาค่าความน่าจะเป็น ของการโยนลูกเต๋า 4 ครั้งแล้วได้ค่า ไม่ เหมือนกัน
Probability of rolling different numbers 4 times in a row
(answer= (5 x4 x3/)6 x6 x6) )
import random
def roll_dice():
return random.randint(1, 6)
def rolls_different_numbers():
rolls = [roll_dice() for i in range(4)]
return len(set(rolls)) == 4
def probability(n):
count = 0
for i in range(n):
if rolls_different_numbers():
count += 1
return count / n
print("Probability of rolling different numbers 4 times in a row:", probability(100000))
Probability of rolling different numbers 4 times in a row: 0.27902
คราวนี้ เอาแบบยาก ๆ ขึ้น
ให้เขียนโปรแกรม หาค่าความน่าจะเป็น ของการโยนลูกเต๋า 4 ครั้งแล้ว หาความเป็นไปได้ของการที่จะมี การออกเลข 1 ติดกันมากกว่าสองครั้ง
(สั่ง ChatGPT สามครั้งเป็นภาษา อังกฤษ ญี่ปุ่น และไทย ปรากฏว่า ให้โค๊ดออกมาเหมือนกัน แต่คอมเมนท์เขียนให้ตามภาษาที่สั่ง อ่านเข้าใจได้ดีด้วย สุดยอดครับ
(answer= 16/(6 x6 x6) )
Here is a Python code that rolls a die 4 times and finds the probability that 1 comes out 2 or more times in a row: In English, Japanese and Thai!
import random
def roll_dice(n):
# Roll a die n times and return the list of outcomes
return [random.randint(1, 6) for i in range(n)]
def has_consecutive_ones(dice_rolls):
# Return True if the list contains two or more consecutive ones
for i in range(len(dice_rolls) - 1):
if dice_rolls[i] == dice_rolls[i+1] == 1:
return True
return False
num_trials = 100000 # The number of times to roll the die
count = 0
for i in range(num_trials):
rolls = roll_dice(4)
if has_consecutive_ones(rolls):
count += 1
probability = count / num_trials
print("The probability of rolling two or more consecutive ones in four rolls is:", probability)
The probability of rolling two or more consecutive ones in four rolls is: 0.07324
นี่ในภาษาญี่ปุ่น
import random
def roll_dice(n):
# サイコロをn回振って、出目のリストを返す
return [random.randint(1, 6) for i in range(n)]
def has_consecutive_ones(dice_rolls):
# 出目のリストに1が2回以上続けて出た場合にTrueを返す
for i in range(len(dice_rolls) - 1):
if dice_rolls[i] == dice_rolls[i+1] == 1:
return True
return False
num_trials = 1000000 # 試行回数
count = 0
for i in range(num_trials):
rolls = roll_dice(4)
if has_consecutive_ones(rolls):
count += 1
probability = count / num_trials
print("The probability of rolling two or more consecutive ones in four rolls is:", probability)
The probability of rolling two or more consecutive ones in four rolls is: 0.074344
ต่อไปเป็นการสั่งเป็นภาษาไทย
Input to ChatGPT:สร้างรหัส python ที่ทอยลูกเต๋า 4 ครั้งและค้นหาความน่าจะเป็นที่ 1 จะออก 2 ครั้งขึ้นไปติดต่อกัน
Response from ChatGPT: นี่คือรหัส Python ที่ทอยลูกเต๋า 4 ครั้งและค้นหาความน่าจะเป็นที่ 1 จะออก 2 ครั้งขึ้นไปติดต่อกัน:
import random
def roll_dice(n):
# ทอยลูกเต๋า n ครั้งและส่งคืนรายการผลลัพธ์
return [random.randint(1, 6) for i in range(n)]
def has_consecutive_ones(dice_rolls):
# คืนค่า True ถ้ารายการมี 1 2 ค่าที่ติดกันหรือมากกว่า
for i in range(len(dice_rolls) - 1):
if dice_rolls[i] == dice_rolls[i+1] == 1:
return True
return False
num_trials = 1000000 # จำนวนครั้งที่ทอยลูกเต๋า
count = 0
for i in range(num_trials):
rolls = roll_dice(4)
if has_consecutive_ones(rolls):
count += 1
probability = count / num_trials
print("ความน่าจะเป็นของการทอยลูกเต๋าแล้วได้ 1 ออก 2 ครั้งขึ้นไปติดต่อกันใน 4 ครั้งคือ:", probability)
ความน่าจะเป็นของการทอยลูกเต๋าแล้วได้ 1 ออก 2 ครั้งขึ้นไปติดต่อกันใน 4 ครั้งคือ: 0.073787
この記事が気に入ったらサポートをしてみませんか?