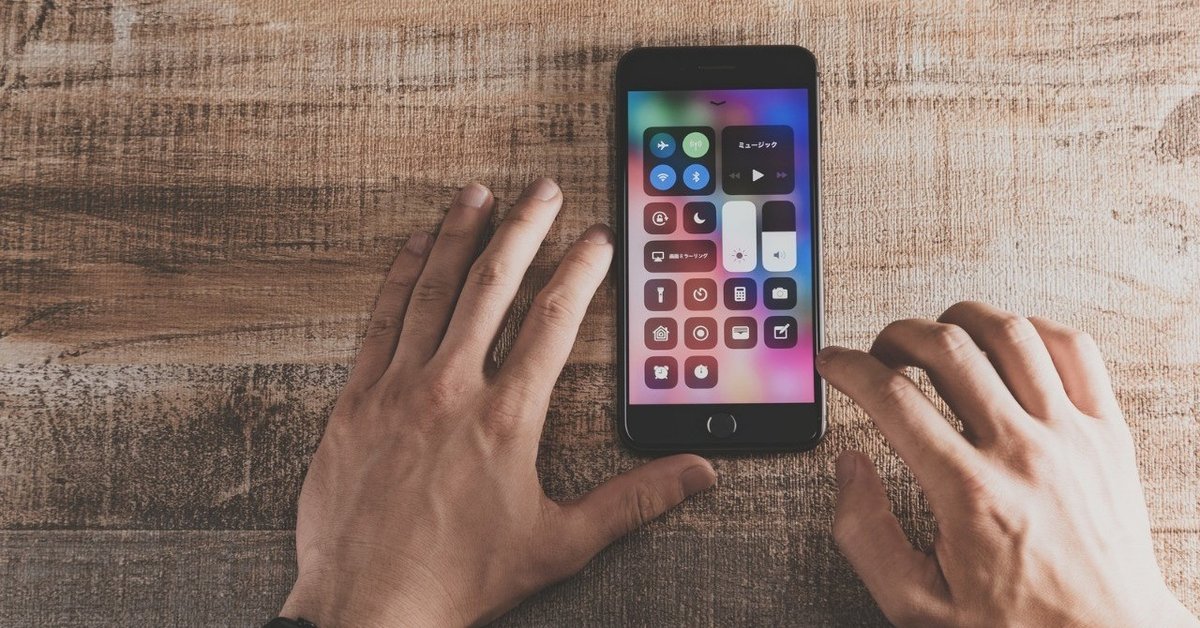
【Objective−C】モーダルビューを呼び出す(描画する)処理の書き方
こういう人に向けて発信しています。
・下から上に出てくるモーダルビュー表示をやりたい人
・コードでナビゲーションバーを表示する方法も知りたい人
・Objective−C 初心者から中級者向け
コード自体は単純です。
TableViewController *tableVC = [[TableViewController alloc] init];
UINavigationController *navigationController = [[UINavigationController alloc] initWithRootViewController:tableVC];
[self presentViewController:navigationController animated:YES completion:nil];
TableViewControllerが表示させたいクラスですね。
それをナビゲーションバー採用した上でモーダル表示しております。
NavigationControllerも実装した方がいい理由
モーダル表示をするとユーザーは帰って来る事が出来ない、
袋小路状態になってしまうので戻るボタンは実装してあげる必要あり。
なので、今回は冗長かもですが、
NavigationBarItemも含めてコード残していきます。
使うクラスについて(今回はxibファイルは不使用)
(1)ViewController.h
(2)ViewController.m
(3)TableViewController.h
(4)TableViewController.m
ViewController.h
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController
@end
ViewController.m
#import "ViewController.h"
#import "TableViewController.h"
@interface ViewController (){
UIButton *btn;
}
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
[self initButton];
}
-(void)initButton{
btn = [[UIButton alloc]initWithFrame:CGRectMake(0, 40.0f,self.view.bounds.size.width, 10.0f)];
[btn setTitle:@"モーダルビューが展開。" forState:UIControlStateNormal];
[btn setTitleColor:[UIColor blueColor] forState:UIControlStateNormal]; //有効時
[btn addTarget:self action:@selector(tappedButton:)
forControlEvents:UIControlEventTouchDown];
[self.view addSubview:btn];
}
-(void)tappedButton:(UIButton*)button{
// ここに何かの処理を記述する
UIAlertController *alertController = [UIAlertController alertControllerWithTitle:@"モーダルを展開してよろしいですか?" message:nil preferredStyle:UIAlertControllerStyleAlert];
//preferredStyle:UIAlertControllerStyleActionSheet = アクションシート
//preferredStyle:UIAlertControllerStyleAlert = アラートビュー
[alertController addAction:[UIAlertAction actionWithTitle:@"はい" style:UIAlertActionStyleDefault handler:^(UIAlertAction *action) {
//yesボタンが押された時の処理
[self showTableViewController];
}]];
[alertController addAction:[UIAlertAction actionWithTitle:@"いいえ" style:UIAlertActionStyleDefault handler:^(UIAlertAction *action) {
// cancelボタンが押された時の処理
}]];
[self presentViewController:alertController animated:YES completion:nil];
}
- (void)showTableViewController{
TableViewController *tableVC = [[TableViewController alloc] init];
UINavigationController *navigationController = [[UINavigationController alloc] initWithRootViewController:tableVC];
[self presentViewController:navigationController animated:YES completion:nil];
}
@end
TableViewController.h
#import <UIKit/UIKit.h>
@interface TableViewController : UITableViewController
@property (nonatomic) NSMutableArray *cellNameArray;
@end
TableViewController.m
#import "TableViewController.h"
@interface TableViewController ()<UINavigationControllerDelegate>
@end
@implementation TableViewController
- (void)viewDidLoad {
[super viewDidLoad];
_cellNameArray = [@[@"value1", @"value2", @"value3"] mutableCopy];
[self loadNavigationBarItem];
}
- (void)loadNavigationBarItem{
self.navigationItem.title = @"TableViewControllerクラス";
UIBarButtonItem *backBtn = [[UIBarButtonItem alloc]
initWithTitle:@"閉じる" // ボタンタイトル名を指定
style:UIBarButtonItemStylePlain
target:self // デリゲートのターゲットを指定
action:@selector(closeModel) // ボタンが押されたときに呼ばれるメソッドを指定
];
self.navigationItem.leftBarButtonItem = backBtn;
}
- (void)closeModel{
[self dismissViewControllerAnimated:YES completion:nil];
}
#pragma mark - Table view data source
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
return _cellNameArray.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
NSString *cellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:cellIdentifier];
if (!cell) {
cell = [[UITableViewCell alloc]initWithStyle:UITableViewCellStyleDefault reuseIdentifier:cellIdentifier];
}
cell.textLabel.text = _cellNameArray[indexPath.row];
return cell;
}
@end