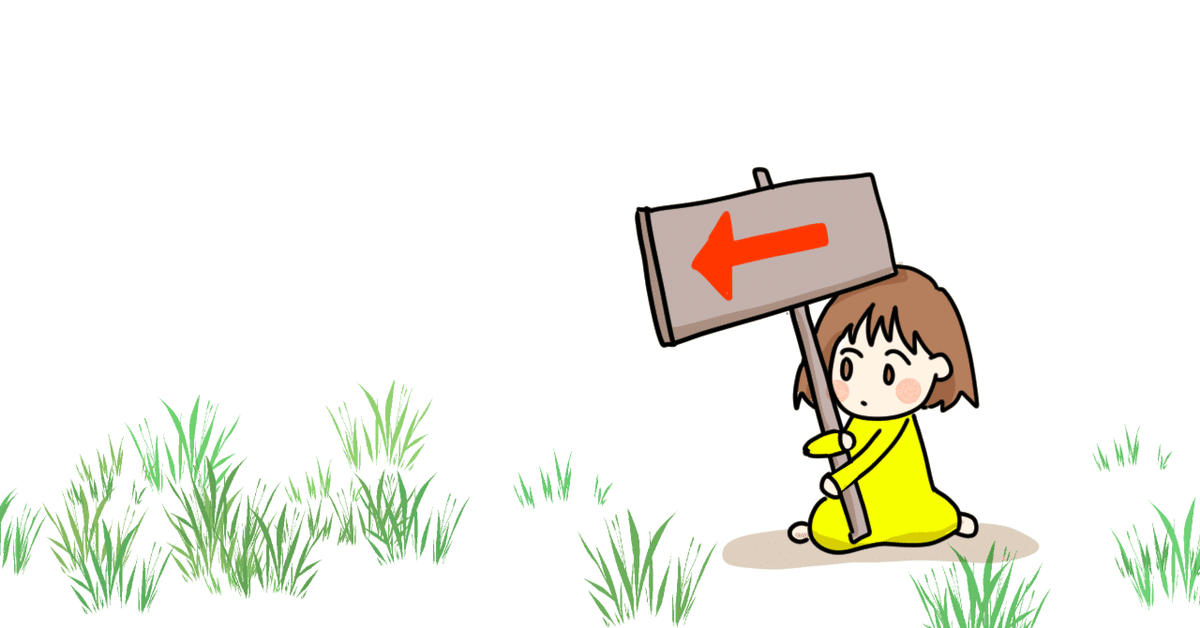
Photo by
katiko
インジケーターをEA化するEAソースコード付き
インジケーターのシグナルでエントリーしてストップリミットで決済するEAです。
インジケーターのシグナルでエントリーするのでインジケーターバッファ番号を指定してください。
データ・ウインドウを表示して"Volume"の下にインジケーターのバッファがあります。上から0,1,2と0番から番号を内部的に振ってあります。
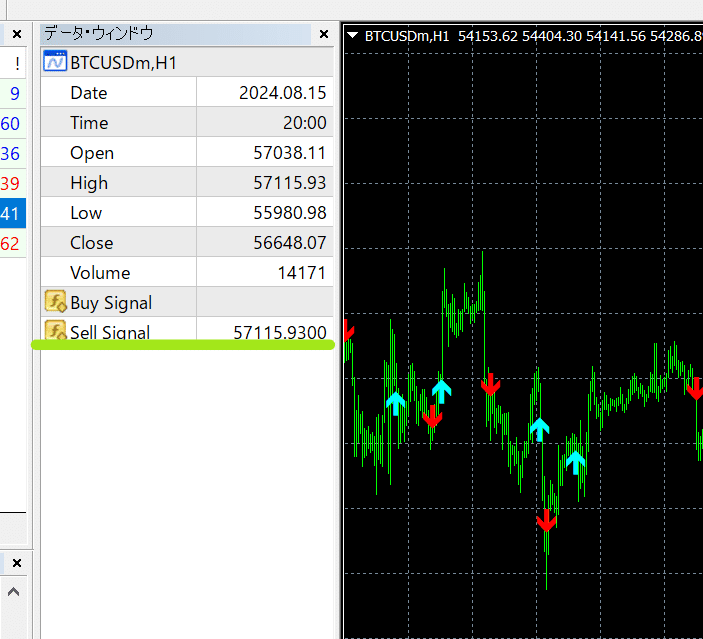
ダウンロード
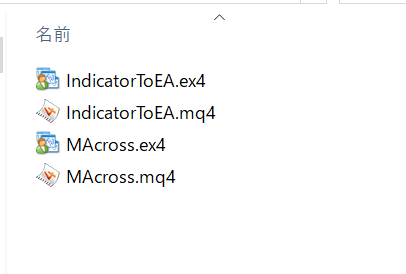
フォルダの設置場所
IndicatorToEAはExpertsフォルダへ
MAcrossはIndicatorsへ
ソースコード
//+------------------------------------------------------------------+
//| ProjectName |
//| Copyright 2018, CompanyName |
//| http://www.companyname.net |
//+------------------------------------------------------------------+
#property strict
// 入力パラメーター
input string InpIndicatorName = "Your_Custom_Indicator"; // インジケーター名
input ENUM_TIMEFRAMES InpCustomTimeframe = PERIOD_CURRENT; // インジケーターのタイムフレーム
input int InpIndicatorShift = 0; // インジケーターのシフト
input int InpBuySignalBuffer = 0; // インジケーターのBUYシグナルバッファー
input int InpSellSignalBuffer = 1; // インジケーターのSELLシグナルバッファー
// インジケーターパラメーター
input double InpParam1 = 0; // インジケーターパラメーター1
input double InpParam2 = 0; // インジケーターパラメーター2
input double InpParam3 = 0; // インジケーターパラメーター3
input double InpParam4 = 0; // インジケーターパラメーター4
input double InpParam5 = 0; // インジケーターパラメーター5
input double InpParam6 = 0; // インジケーターパラメーター6
input double InpParam7 = 0; // インジケーターパラメーター7
input double InpParam8 = 0; // インジケーターパラメーター8
input double InpParam9 = 0; // インジケーターパラメーター9
input double InpParam10 = 0; // インジケーターパラメーター10
// 取引パラメーター
input double InpLotSize = 0.1; // ロットサイズ
input int InpStopLoss = 100; // ストップロス(ポイント)
input int InpTakeProfit = 200; // テイクプロフィット(ポイント)
input bool InpLimitEntryPerCandle = false; // エントリーをローソク足につき一回に制限する
int MagicNumber = 789;
bool entry_on = false;
static datetime last_entry_time = 0;
int OnInit()
{
return INIT_SUCCEEDED;
}
bool is_buy()
{
double signal_value = iCustom(Symbol(), InpCustomTimeframe, InpIndicatorName,
InpParam1, InpParam2, InpParam3, InpParam4, InpParam5,
InpParam6, InpParam7, InpParam8, InpParam9, InpParam10,
InpBuySignalBuffer, InpIndicatorShift);
return (signal_value != EMPTY_VALUE && signal_value != 0);
}
bool is_sell()
{
double signal_value = iCustom(Symbol(), InpCustomTimeframe, InpIndicatorName,
InpParam1, InpParam2, InpParam3, InpParam4, InpParam5,
InpParam6, InpParam7, InpParam8, InpParam9, InpParam10,
InpSellSignalBuffer, InpIndicatorShift);
return (signal_value != EMPTY_VALUE && signal_value != 0);
}
void OnTick()
{
if(InpLimitEntryPerCandle)
{
datetime current_candle_time = iTime(Symbol(), PERIOD_CURRENT, 0);
if(current_candle_time != last_entry_time)
{
entry_on = true;
last_entry_time = current_candle_time;
}
}
else
{
entry_on = true;
}
if(entry_on && position_count(OP_BUY) == 0 && position_count(OP_SELL) == 0)
{
if(is_buy())
{
position_entry(OP_BUY);
entry_on = false;
}
else if(is_sell())
{
position_entry(OP_SELL);
entry_on = false;
}
}
}
int position_count(int side)
{
int count = 0;
for(int i = OrdersTotal() - 1; i >= 0; i--)
{
if(OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
if(OrderType() == side && OrderSymbol() == Symbol() && OrderMagicNumber() == MagicNumber)
{
count++;
}
}
}
return count;
}
void position_entry(int side)
{
double sl = 0, tp = 0;
if(side == OP_BUY)
{
sl = (InpStopLoss == 0) ? 0 : Ask - InpStopLoss * Point;
tp = (InpTakeProfit == 0) ? 0 : Ask + InpTakeProfit * Point;
OrderSend(Symbol(), OP_BUY, InpLotSize, Ask, 0, sl, tp, NULL, MagicNumber, 0, clrGreen);
}
else if(side == OP_SELL)
{
sl = (InpStopLoss == 0) ? 0 : Bid + InpStopLoss * Point;
tp = (InpTakeProfit == 0) ? 0 : Bid - InpTakeProfit * Point;
OrderSend(Symbol(), OP_SELL, InpLotSize, Bid, 0, sl, tp, NULL, MagicNumber, 0, clrRed);
}
}
void position_close(int side)
{
for(int i = OrdersTotal() - 1; i >= 0; i--)
{
if(OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
if(OrderType() == side && OrderSymbol() == Symbol() && OrderMagicNumber() == MagicNumber)
{
OrderClose(OrderTicket(), OrderLots(), OrderClosePrice(), 0, clrBlue);
}
}
}
}
おまけでインジケーター
#property copyright "Copyright 2023, Your Name Here"
#property link "https://www.mql5.com"
#property version "1.00"
#property strict
#property indicator_chart_window
#property indicator_buffers 2
#property indicator_color1 clrAqua // BUYシグナルの色を青に変更
#property indicator_color2 clrRed // SELLシグナルの色を赤に設定
#property indicator_width1 3
#property indicator_width2 3
// インジケーターの入力パラメーター
input int FastMA = 10; // 短期移動平均線の期間
input int SlowMA = 30; // 長期移動平均線の期間
input ENUM_MA_METHOD MAMethod = MODE_SMA; // 移動平均線の計算方法
input ENUM_APPLIED_PRICE AppliedPrice = PRICE_CLOSE; // 適用価格
// インジケーターバッファ
double BuyBuffer[];
double SellBuffer[];
//+------------------------------------------------------------------+
//| Custom indicator initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
// インジケーターバッファの設定
SetIndexStyle(0, DRAW_ARROW);
SetIndexArrow(0, 233); // 上向き矢印
SetIndexBuffer(0, BuyBuffer);
SetIndexLabel(0, "Buy Signal");
SetIndexStyle(1, DRAW_ARROW);
SetIndexArrow(1, 234); // 下向き矢印
SetIndexBuffer(1, SellBuffer);
SetIndexLabel(1, "Sell Signal");
// 矢印のサイズを3倍に設定
SetIndexArrow(0, 233); // デフォルトサイズは5なので、3倍にするには15を設定
SetIndexArrow(1, 234);
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,
const int prev_calculated,
const datetime &time[],
const double &open[],
const double &high[],
const double &low[],
const double &close[],
const long &tick_volume[],
const long &volume[],
const int &spread[])
{
int limit = rates_total - prev_calculated;
if (prev_calculated > 0) limit++;
for (int i = limit - 1; i >= 0; i--)
{
double fastMA_current = iMA(NULL, 0, FastMA, 0, MAMethod, AppliedPrice, i);
double slowMA_current = iMA(NULL, 0, SlowMA, 0, MAMethod, AppliedPrice, i);
double fastMA_prev = iMA(NULL, 0, FastMA, 0, MAMethod, AppliedPrice, i + 1);
double slowMA_prev = iMA(NULL, 0, SlowMA, 0, MAMethod, AppliedPrice, i + 1);
// ゴールデンクロス(GC)の検出
if (fastMA_prev <= slowMA_prev && fastMA_current > slowMA_current)
{
BuyBuffer[i] = low[i]; // 買いシグナル(上向き矢印)
SellBuffer[i] = EMPTY_VALUE;
}
// デッドクロス(DC)の検出
else if (fastMA_prev >= slowMA_prev && fastMA_current < slowMA_current)
{
SellBuffer[i] = high[i]; // 売りシグナル(下向き矢印)
BuyBuffer[i] = EMPTY_VALUE;
}
else
{
BuyBuffer[i] = EMPTY_VALUE;
SellBuffer[i] = EMPTY_VALUE;
}
}
return(rates_total);
}
設定方法
1.データフォルダを開く
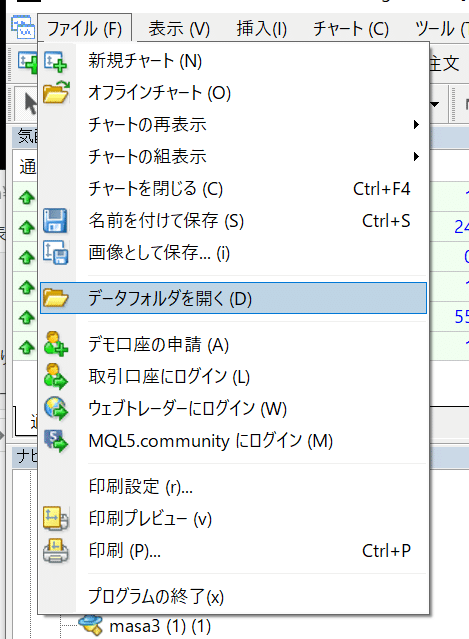
2.MQL4フォルダを開く

3.Indivatorsフォルダを開く

4.インジケーターの名前を変更から
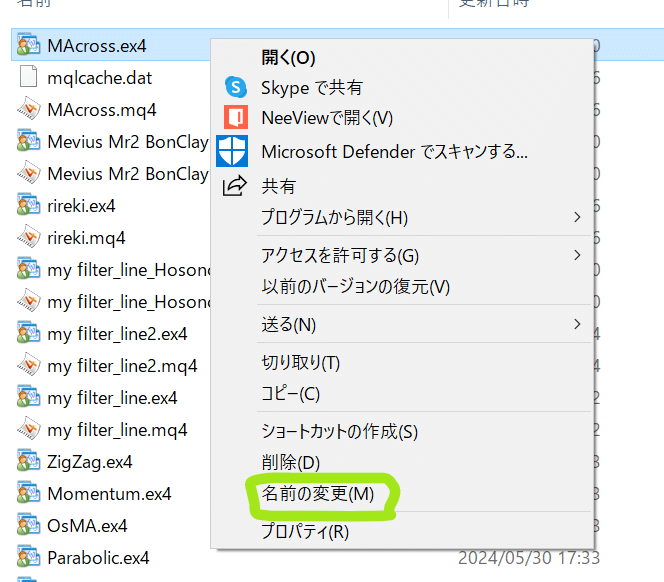
5.名前を選択になるのでコピーする
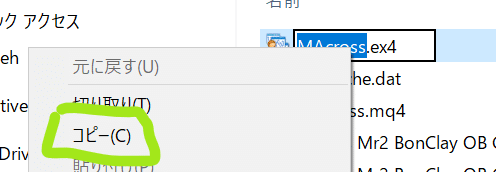
6.EAをセットしてパラメーターに張り付ける
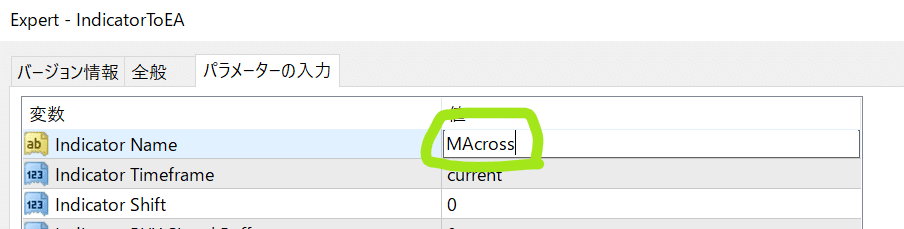
7.インジケーターの設定を入力する(忘れやすいので注意)
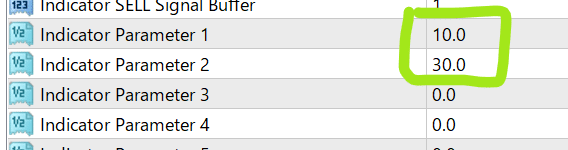
8.一個前に矢印でたらエントリーする場合はシフトを1にする
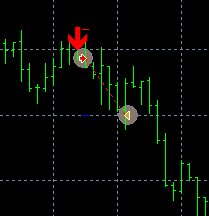

9.同じローソク足でエントリーと決済が複数になるのを阻止する場合は制限機能をtrueにする

10.おわり
設定おわりです。
バックテストでも設定は同じなので試してみてね。
いいなと思ったら応援しよう!
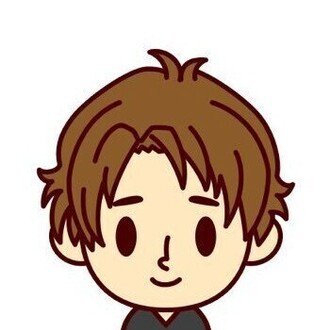