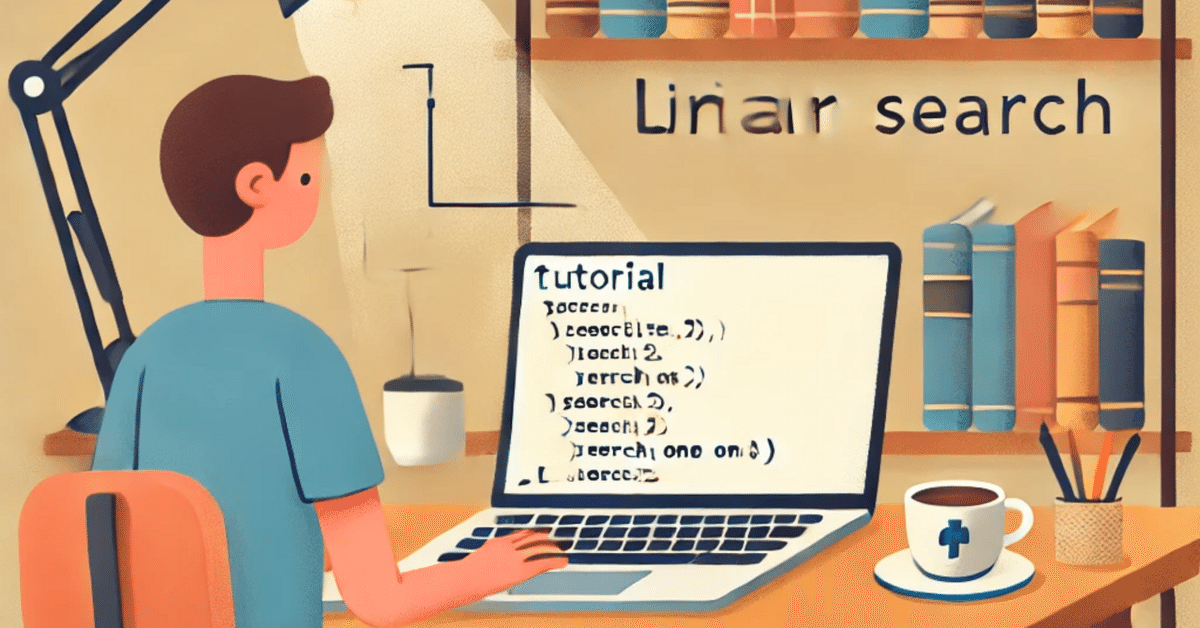
線形探索(Linear Search)アルゴリズム解説
超シンプルな線形探索入門!〜Pythonでゼロからアルゴリズムを学ぼう〜
コード例
※上のコード例を元に、chatGPTが書きました。
プログラミングを始めたばかりの方でも、線形探索(Linear Search)って聞いたことありますか?アルゴリズムの中でも最もシンプルでわかりやすい方法のひとつです。今日は、この線形探索をPythonでゼロから実装してみましょう!コードをひとつずつ分解して解説するので、「なんだ、簡単じゃん!」って思ってもらえるはずです。
線形探索って何?
線形探索とは、リストや文字列の中から「特定の要素」を見つけるために、先頭から順番に一つずつチェックしていく方法です。
イメージは、図書館で本を探しているときに、棚の一冊一冊を手に取って確認する感じ。リストの中で探している要素(ターゲット)が見つかれば、そのインデックス(何番目にあるか)を返します。見つからなかったら「無いよー」と空の結果を返す、そんなシンプルな仕組みです。
(1) 複数要素を考慮しない線形探索
ステップ1: リストの要素を順番に取り出してみよう
まずは、Pythonでリストの要素を順に取り出して表示してみます。
numbers = [0, 2, 4, 9, 21, 5]
for element in numbers:
print(element)
出力:
0
2
4
9
21
5
リストの中身が順番に表示されましたね!これが探索の第一歩です。
ステップ2: 一致したらインデックスを表示
次に、リスト内の要素と探している数字が一致したら、そのインデックスを表示してみましょう。
numbers = [0, 2, 4, 9, 21, 5]
target = 2 # 探す数字
idx = 0
for element in numbers:
if element == target:
print(idx)
idx += 1
出力:
1
「2」はリストの1番目にありました!この仕組みを使えば、リストのどこにあるかが分かりますね。
ステップ3: enumerateでスッキリしたコードに
Pythonには、インデックスと要素を一緒に取り出せる便利な関数、enumerateがあります。これを使ってさらにコードをスッキリさせましょう。
numbers = [0, 2, 4, 9, 21, 5]
target = 2
for idx, element in enumerate(numbers):
if element == target:
print(idx)
出力:
1
簡単でスッキリしたコードになりましたね!
ステップ4: 関数化して何度でも使えるようにしよう
最後に、この探索の流れを関数にして、簡単に使えるようにします。
def linear_search(target, lst):
"""
線形探索でターゲットがリスト内にあれば、そのインデックスを返します。
無ければNoneを返します。
"""
for idx, element in enumerate(lst):
if element == target:
return idx
return None
使い方:
numbers = [0, 2, 45, 3, 18, 8]
result = linear_search(45, numbers)
if result is not None:
print(f"ターゲットは {result} 番目にあります!")
else:
print("ターゲットは見つかりませんでした。")
出力:
ターゲットは 2 番目にあります!
(2) 複数要素を考慮する
次に、リストや文字列の中に同じ要素が複数あった場合、それらすべてのインデックスを返す方法を見てみましょう。
ステップ1: 文字列から探してインデックスを表示
例えば、「python」という文字列の中に「y」という文字が何回出てくるか探してみましょう。
letters = "Let's enjoy python"
target = "y"
for idx, element in enumerate(letters):
if element == target:
print(idx)
出力:
10
13
「y」は10番目と13番目にありましたね!
ステップ2: インデックスをリストにまとめてみよう
見つけたインデックスをリストにまとめて返してみましょう。
letters = "Let's enjoy python"
target = "y"
indices = []
for idx, element in enumerate(letters):
if element == target:
indices.append(idx)
print(indices)
出力:
[10, 13]
ステップ3: 複数のインデックスを返す関数を作ろう
次に、複数のインデックスを返す関数を作ります。
def global_linear_search(target, lst):
"""
線形探索でターゲットがリストや文字列内にあれば、すべてのインデックスを返します。
無ければ空のリストを返します。
"""
indices = []
for idx, element in enumerate(lst):
if element == target:
indices.append(idx)
return indices
使い方:
letters = "Let's enjoy python"
print(global_linear_search("y", letters)) # [10, 13]
print(global_linear_search("e", letters)) # [1, 6]
print(global_linear_search("z", letters)) # []
出力:
[10, 13]
[1, 6]
[]
f-stringで出力をカッコよく!
ところで、出力の部分で f"..." という構文を使いましたよね。これをf-stringといいます。Pythonでは、文字列の中に変数や計算結果を簡単に埋め込むことができます。
例えば:
name = "Alice"
age = 25
print(f"こんにちは!私は{name}で、年齢は{age}歳です。")
出力:
こんにちは!私はAliceで、年齢は25歳です。
f-stringを使うと、コードがシンプルで見やすくなるので、ぜひ覚えておいてください!
線形探索のまとめ
線形探索はシンプルなアルゴリズムで、リストや文字列の中から特定の要素を探します。
複数の要素がある場合も対応可能です。
f-stringを使うと、結果の表示がカッコよくできます!
線形探索が理解できたら、次は「二分探索」など、もう少し効率的なアルゴリズムに挑戦してみるのも良いですね!
プログラミングの世界は楽しいですよ!どんどんチャレンジして、自分だけのコードを作ってみましょう。
おまけ
ついでに英語も学べるように、英語に翻訳もお願いしてみました。
A Super Simple Introduction to Linear Search! ~ Learn Algorithms from Scratch with Python ~
Have you just started programming and heard the term "linear search"? It's one of the simplest and easiest-to-understand algorithms out there. Today, let’s implement this linear search in Python from scratch! By breaking down the code step by step, you'll see how easy it can be!
What is Linear Search?
Linear search is a method where you go through a list or a string one by one from the beginning to find a "specific element".
Think of it like looking for a book in a library: you pick up each book from the shelf one by one to check if it's the one you're looking for. When you find the element (the target), you return its index (the position in the list). If the element isn't there, it simply returns "not found" — it’s that straightforward.
(1) Linear Search without Considering Multiple Elements
Step 1: Extract the Elements from the List One by One
First, let's extract the elements from a list and display them in Python.
numbers = [0, 2, 4, 9, 21, 5]
for element in numbers:
print(element)
Output:
0
2
4
9
21
5
You can see that the elements of the list are printed one by one. This is the first step in the search.
Step 2: Display the Index When There is a Match
Next, let’s display the index when the element in the list matches the number we’re looking for.
numbers = [0, 2, 4, 9, 21, 5]
target = 2 # The number we’re searching for
idx = 0
for element in numbers:
if element == target:
print(idx)
idx += 1
Output:
1
Here, “2” is at the 1st position in the list! Using this method, you can find where the element is located in the list.
Step 3: Simplify the Code Using enumerate
Python has a handy function called enumerate that allows you to extract both the index and the element at the same time. Let’s use this to simplify the code.
numbers = [0, 2, 4, 9, 21, 5]
target = 2
for idx, element in enumerate(numbers):
if element == target:
print(idx)
Output:
1
Now the code is much simpler and cleaner!
Step 4: Turn It Into a Function for Reusability
Finally, let’s turn this search process into a function so it can be reused easily.
def linear_search(target, lst):
"""
This function performs a linear search and returns the index of the target in the list if found.
If not found, it returns None.
"""
for idx, element in enumerate(lst):
if element == target:
return idx
return None
Usage:
numbers = [0, 2, 45, 3, 18, 8]
result = linear_search(45, numbers)
if result is not None:
print(f"The target is at index {result}!")
else:
print("The target was not found.")
Output:
The target is at index 2!
(2) Considering Multiple Elements
Next, let’s look at how to return all the indexes when the same element appears multiple times in a list or string.
Step 1: Search for an Element in a String and Display the Index
For example, let’s find how many times the letter "y" appears in the string "Let's enjoy python."
letters = "Let's enjoy python"
target = "y"
for idx, element in enumerate(letters):
if element == target:
print(idx)
Output:
10
13
The letter "y" appears at the 10th and 13th positions!
Step 2: Store the Found Indexes in a List
Let’s collect all the found indexes into a list.
letters = "Let's enjoy python"
target = "y"
indices = []
for idx, element in enumerate(letters):
if element == target:
indices.append(idx)
print(indices)
Output:
[10, 13]
Step 3: Create a Function to Return Multiple Indexes
Now, let’s create a function that returns all the indexes where the target is found.
def global_linear_search(target, lst):
"""
This function performs a linear search and returns all indexes where the target is found in the list or string.
If not found, it returns an empty list.
"""
indices = []
for idx, element in enumerate(lst):
if element == target:
indices.append(idx)
return indices
Usage:
letters = "Let's enjoy python"
print(global_linear_search("y", letters)) # [10, 13]
print(global_linear_search("e", letters)) # [1, 6]
print(global_linear_search("z", letters)) # []
Output:
[10, 13]
[1, 6]
[]
Styling Your Output with f-strings!
By the way, did you notice the f"..." syntax used in the output? This is called an f-string, a feature in Python that allows you to easily embed variables or calculation results into strings.
For example:
name = "Alice"
age = 25
print(f"Hello! My name is {name}, and I am {age} years old.")
Output:
Hello! My name is Alice, and I am 25 years old.
Using f-strings makes your code cleaner and more readable, so make sure to remember this trick!
Summary of Linear Search
Linear search is a simple algorithm that helps you find a specific element in a list or string.
It can handle multiple elements when needed.
f-strings make displaying results look more polished!
Once you’ve mastered linear search, why not challenge yourself with more efficient algorithms like binary search? The world of programming is fun! Keep challenging yourself and write your own unique code!