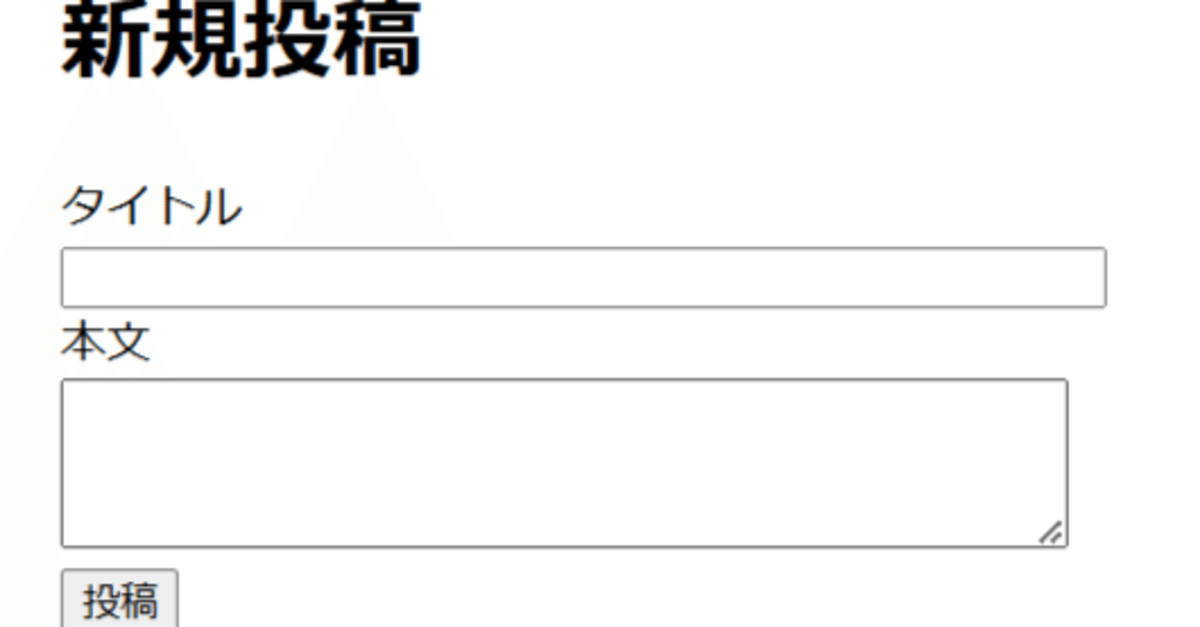
Roo ClineにFlaskでブログ作ってもらった。
VS Codeに入れたRoo Clineで自動でアプリを作ってもらうのが面白くてPythonでFlaskを使ったWEBアプリの簡易ブログを作ってもらいました。
APIはDeepSeekを使ってます。
Roo Clineへ頼んだプロンプトは以下です。Win11でWSL2を使っています。
Q.「Flaskをつかったシンプルなブログシステムを作ってください。
フォルダは/home/garyo/project/flask/blogです。
機能: 記事の投稿、編集、削除、コメント機能
学べること: ORM、リレーショナルデータベースの使い方
主な技術: Flask-SQLAlchemy, Jinja2」
出来上がったのはこんな簡易ブログでタイトル、本文、コメントもつけれます。
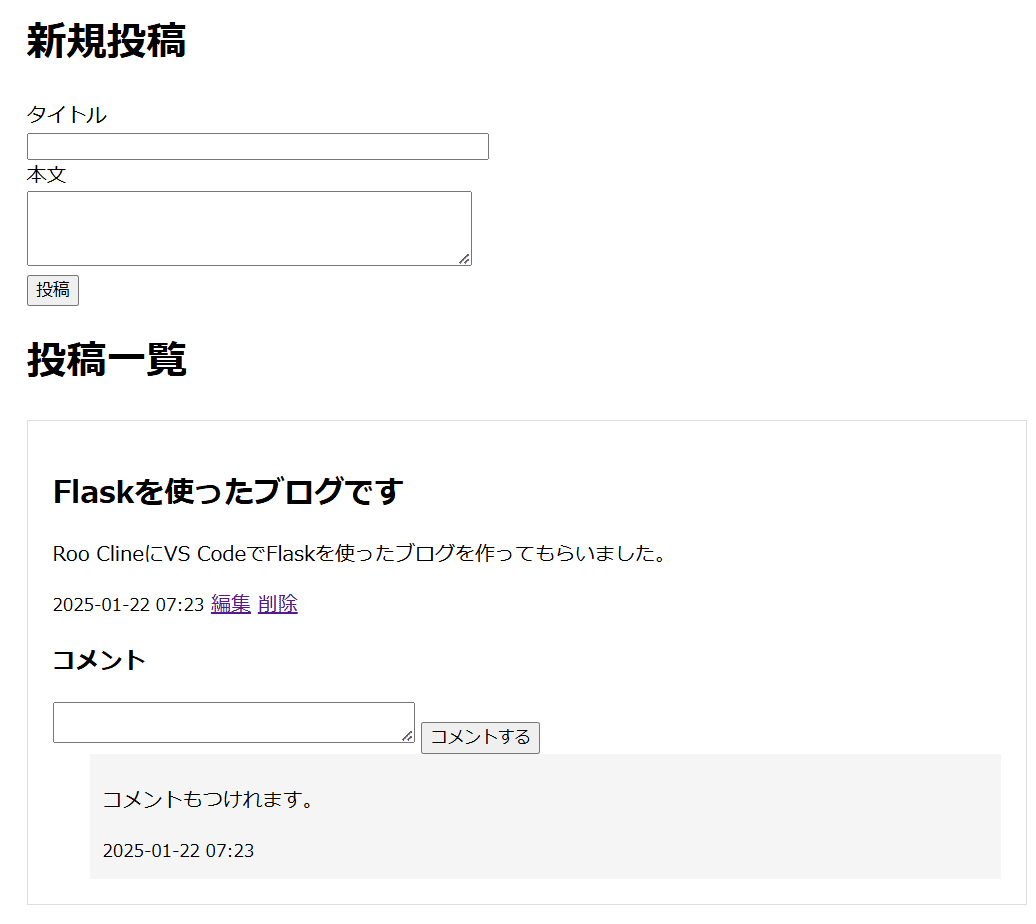
from flask import Flask, render_template, redirect, url_for, request
from flask_sqlalchemy import SQLAlchemy
from flask_wtf import FlaskForm
from wtforms import StringField, TextAreaField, SubmitField
from wtforms.validators import DataRequired
from datetime import datetime
app = Flask(__name__)
app.config['SECRET_KEY'] = 'your-secret-key'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///blog.db'
app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False
db = SQLAlchemy(app)
class Post(db.Model):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(100), nullable=False)
content = db.Column(db.Text, nullable=False)
date_posted = db.Column(db.DateTime, default=datetime.utcnow)
comments = db.relationship('Comment', backref='post', cascade='all, delete-orphan')
class Comment(db.Model):
id = db.Column(db.Integer, primary_key=True)
content = db.Column(db.Text, nullable=False)
date_posted = db.Column(db.DateTime, default=datetime.utcnow)
post_id = db.Column(db.Integer, db.ForeignKey('post.id'), nullable=False)
class PostForm(FlaskForm):
title = StringField('タイトル', validators=[DataRequired()])
content = TextAreaField('本文', validators=[DataRequired()])
submit = SubmitField('投稿')
class CommentForm(FlaskForm):
content = TextAreaField('コメント', validators=[DataRequired()])
submit = SubmitField('コメントする')
@app.route('/')
def index():
posts = Post.query.order_by(Post.date_posted.desc()).all()
post_form = PostForm()
comment_form = CommentForm()
return render_template('index.html',
posts=posts,
post_form=post_form,
comment_form=comment_form)
@app.route('/post', methods=['POST'])
def create_post():
form = PostForm()
if form.validate_on_submit():
post = Post(title=form.title.data, content=form.content.data)
db.session.add(post)
db.session.commit()
return redirect(url_for('index'))
@app.route('/post/<int:id>/edit', methods=['GET', 'POST'])
def edit_post(id):
post = Post.query.get_or_404(id)
form = PostForm()
if form.validate_on_submit():
post.title = form.title.data
post.content = form.content.data
db.session.commit()
return redirect(url_for('index'))
elif request.method == 'GET':
form.title.data = post.title
form.content.data = post.content
return render_template('edit_post.html', form=form)
@app.route('/post/<int:id>/delete')
def delete_post(id):
post = Post.query.get_or_404(id)
db.session.delete(post)
db.session.commit()
return redirect(url_for('index'))
@app.route('/post/<int:post_id>/comment', methods=['POST'])
def add_comment(post_id):
form = CommentForm()
if form.validate_on_submit():
comment = Comment(content=form.content.data, post_id=post_id)
db.session.add(comment)
db.session.commit()
return redirect(url_for('index'))
@ app.cli.command("init-db")
def init_db():
with app.app_context():
db.create_all()
print("Database tables created successfully")
if __name__ == '__main__':
with app.app_context():
db.create_all()
app.run(debug=True)
temlates/base.html
<!DOCTYPE html>
<html>
<head>
<title>Flask Blog</title>
<style>
.container { max-width: 800px; margin: 0 auto; padding: 20px; }
.post { border: 1px solid #ddd; padding: 20px; margin-bottom: 20px; }
.comment { margin-left: 30px; padding: 10px; background: #f5f5f5; }
</style>
</head>
<body>
<div class="container">
{% block content %}{% endblock %}
</div>
</body>
</html>
templates/index.html
{% extends "base.html" %}
{% block content %}
<h1>新規投稿</h1>
<form method="POST" action="{{ url_for('create_post') }}">
{{ post_form.hidden_tag() }}
<div>
{{ post_form.title.label }}<br>
{{ post_form.title(size=50) }}
</div>
<div>
{{ post_form.content.label }}<br>
{{ post_form.content(rows=4, cols=50) }}
</div>
<div>{{ post_form.submit() }}</div>
</form>
<h1>投稿一覧</h1>
{% for post in posts %}
<div class="post">
<h2>{{ post.title }}</h2>
<p>{{ post.content }}</p>
<small>{{ post.date_posted.strftime('%Y-%m-%d %H:%M') }}</small>
<a href="{{ url_for('edit_post', id=post.id) }}">編集</a>
<a href="{{ url_for('delete_post', id=post.id) }}">削除</a>
<h3>コメント</h3>
<form method="POST" action="{{ url_for('add_comment', post_id=post.id) }}">
{{ comment_form.hidden_tag() }}
{{ comment_form.content(rows=2, cols=40) }}
{{ comment_form.submit() }}
</form>
{% for comment in post.comments %}
<div class="comment">
<p>{{ comment.content }}</p>
<small>{{ comment.date_posted.strftime('%Y-%m-%d %H:%M') }}</small>
</div>
{% endfor %}
</div>
{% endfor %}
{% endblock %}
templates/edit_post.html
{% extends "base.html" %}
{% block content %}
<h1>記事編集</h1>
<form method="POST">
{{ form.hidden_tag() }}
<div>
{{ form.title.label }}<br>
{{ form.title(size=50) }}
</div>
<div>
{{ form.content.label }}<br>
{{ form.content(rows=4, cols=50) }}
</div>
<div>{{ form.submit() }}</div>
</form>
{% endblock %}