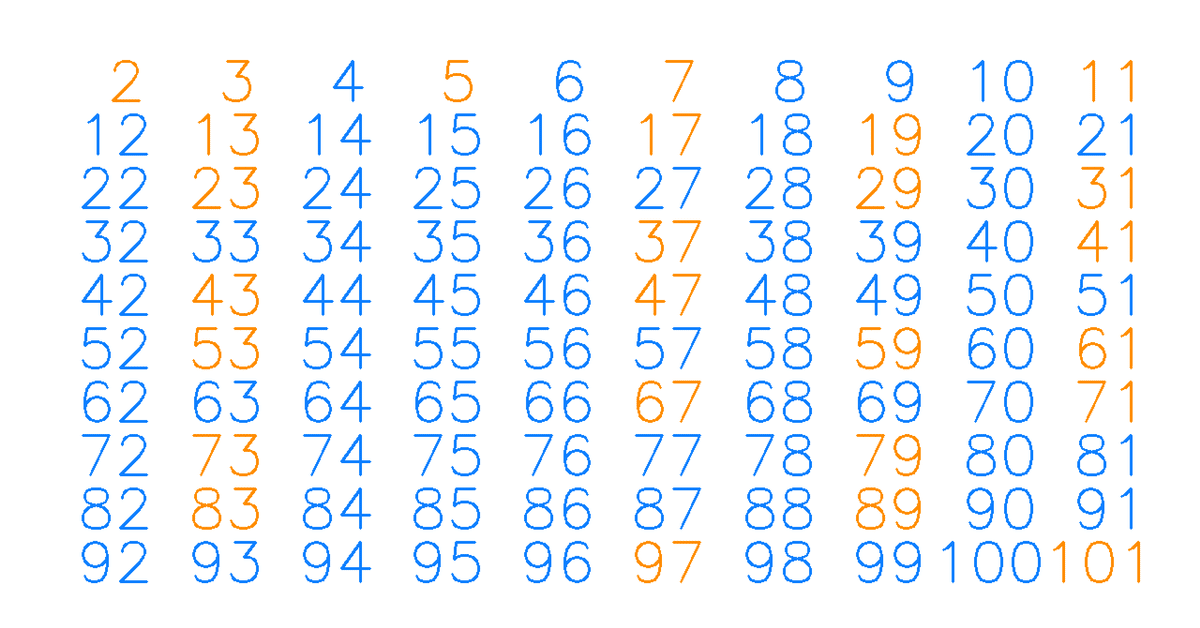
フォトギャラリー用画像#28
2,3,4,5,6… と自然数を並べて、素数をオレンジ色、それ以外を青色で塗ったものがアイキャッチの画像です。エラトステネスの篩という方法で簡単に作れます。素数判定のプログラムよりも、色や位置の調整の方が面倒だったくらいです。
並べる自然数を大きくしていった時の様子を図にしたくて、この題材を選びました。縦横の数を10から16に増やすとこんな感じです。
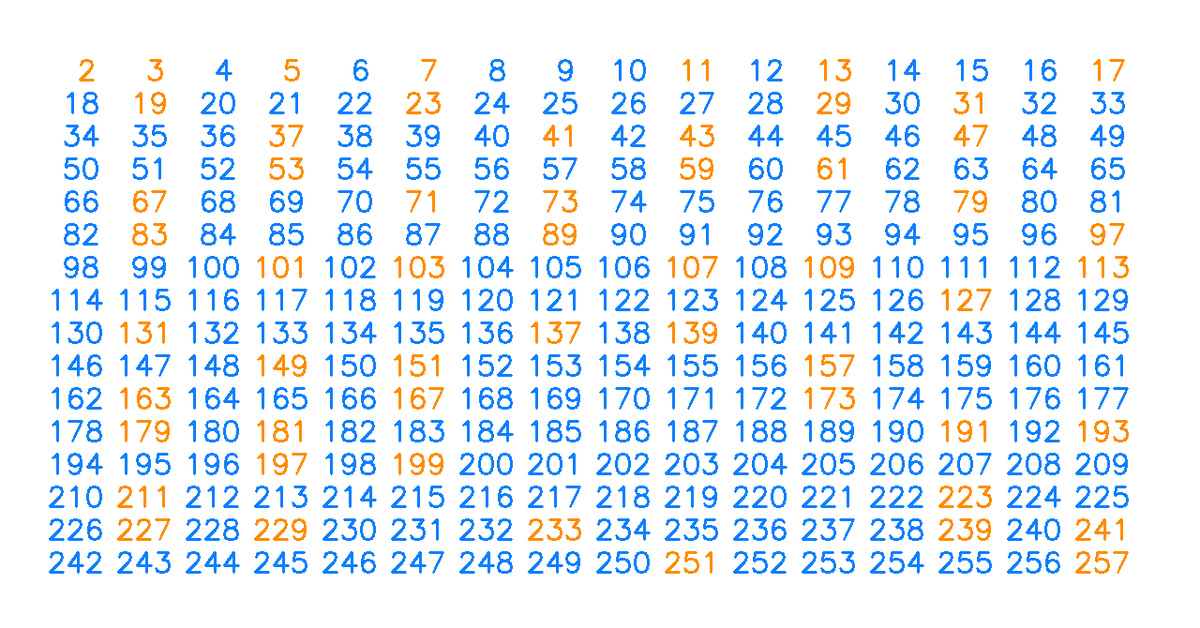
縦32個、横32個に増やすと、次のようになります。
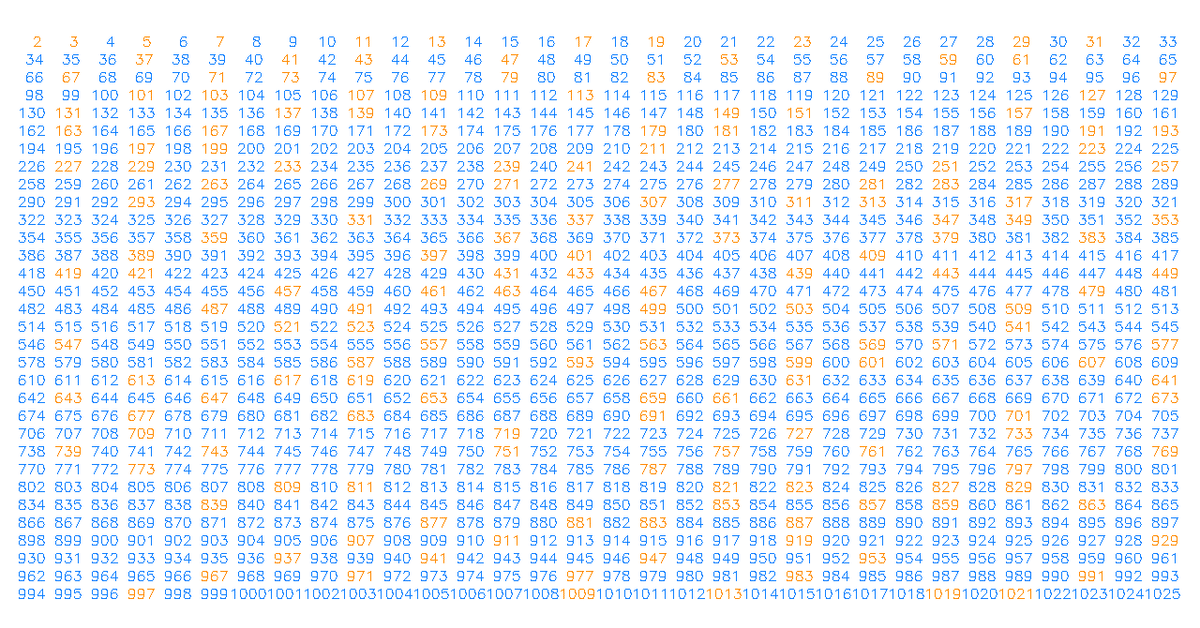
これ以上増やすと数字が読めなくなってしまうので、素数を単に黒の点、それ以外の点を白で表すことにします。そのルールで縦670個、横1280個の場合を描いたものがコチラです。
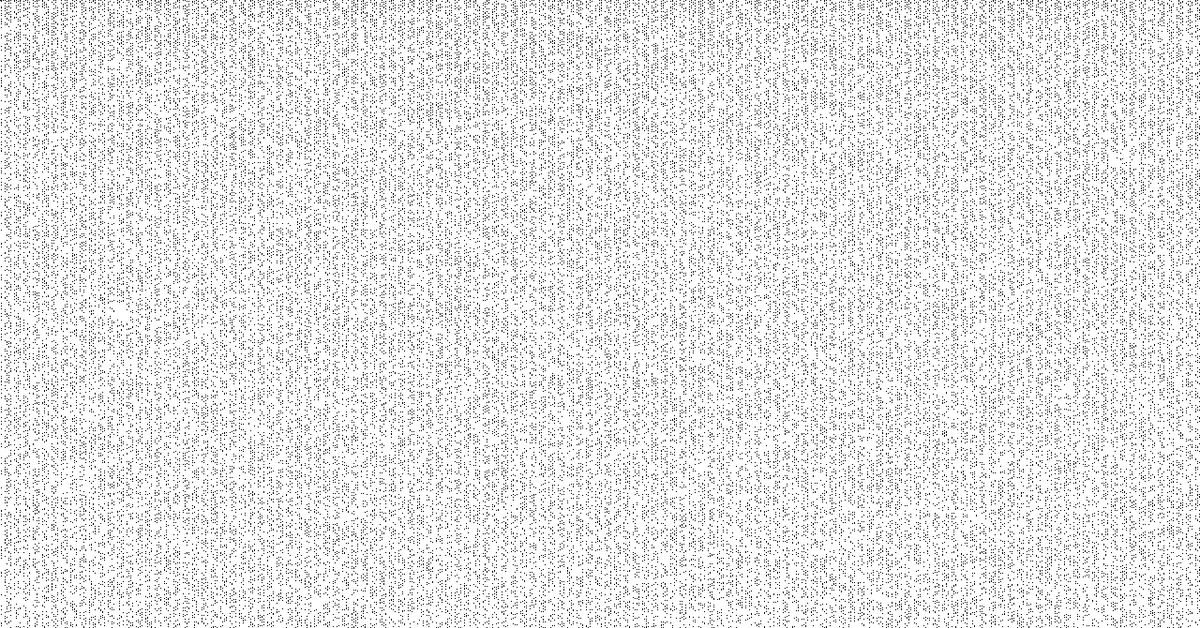
ソースコード
Google Collaboratoryの共有リンク
#!/usr/bin/env python
# coding: utf-8
import numpy as np
import cv2
import matplotlib.pyplot as plt
# 「みんなのフォトギャラリー」用のサイズ
width = 1280; height = 670
# エラトステネスの篩で素数の一覧を返す
def Sieve_of_Eratosthenes (N):
s = np.zeros(N-1)
primes = []
pt = 0
while pt < N-1:
if s[pt] == 0:
val = pt+2
primes.append (val)
pt2 = pt + val
while pt2 < N-1:
s[pt2] = 1
pt2 += val
pt+=1
return primes, s
N = 10000
primes, s = Sieve_of_Eratosthenes (N)
n = 10
offset_w = 50
offset_h = 50
w = (width-2*offset_w) // n
h = (height-2*offset_h) // n
img = np.full((height,width,3),255, dtype=np.uint8)
for i in range(n):
for j in range(n):
val = str(n*i+j+2).rjust(3,' ')
if s[n*i+j] == 0:
col = (0xff,0x8c,0x00)
else :
col = (0x0e,0x7f,0xff)
cv2.putText(img, val,
(w * j+offset_w,
h *(i+1)+offset_h),
cv2.FONT_HERSHEY_SIMPLEX,
2.0, col, thickness=2)
plt.imsave('no+e_photo_gallery_028_1.png', img, cmap='gray',dpi=1000 )
plt.imshow( cv2.cvtColor(img, cv2.COLOR_BGR2RGB) )
n = 16
offset_w = 50
offset_h = 50
w = (width-2*offset_w) // n
h = (height-2*offset_h) // n
img = np.full((height,width,3),255, dtype=np.uint8)
for i in range(n):
for j in range(n):
val = str(n*i+j+2).rjust(3,' ')
if s[n*i+j] == 0:
col = (0xff,0x8c,0x00)
else :
col = (0x0e,0x7f,0xff)
cv2.putText(img, val,
(w * j+offset_w,
h *(i+1)+offset_h),
cv2.FONT_HERSHEY_SIMPLEX,
1.0, col, thickness=2)
plt.imshow( cv2.cvtColor(img, cv2.COLOR_BGR2RGB) )
plt.imsave('no+e_photo_gallery_028_2.png', img, cmap='gray',dpi=1000 )
n = 32
offset_w = 10
offset_h = 20
w = (width-2*offset_w) // n
h = (height-2*offset_h) // n
img = np.full((height,width,3),255, dtype=np.uint8)
for i in range(n):
for j in range(n):
val = str(n*i+j+2).rjust(4,' ')
if s[n*i+j] == 0:
col = (0xff,0x8c,0x00)
else :
col = (0x0e,0x7f,0xff)
cv2.putText(img, val,
(w * j+offset_w,
h *(i+1)+offset_h+10),
cv2.FONT_HERSHEY_SIMPLEX,
0.5, col, thickness=1)
plt.imshow( cv2.cvtColor(img, cv2.COLOR_BGR2RGB) )
plt.imsave('no+e_photo_gallery_028_3.png', img, cmap='gray',dpi=1000 )
# Arrange the integers greater than one from top left to bottom right.
# If an integer is prime, the colour of the cell is black.
N = width * height + 2
p, s = Sieve_of_Eratosthenes (N)
im = s[:len(s)-1].reshape((height,width))
#画像の表示と保存
plt.imshow(im, cmap='gray')
plt.axis('off')
plt.show()
plt.imsave('no+e_photo_gallery_028.png', im, cmap='gray',dpi=1000 )
この記事が気に入ったらサポートをしてみませんか?