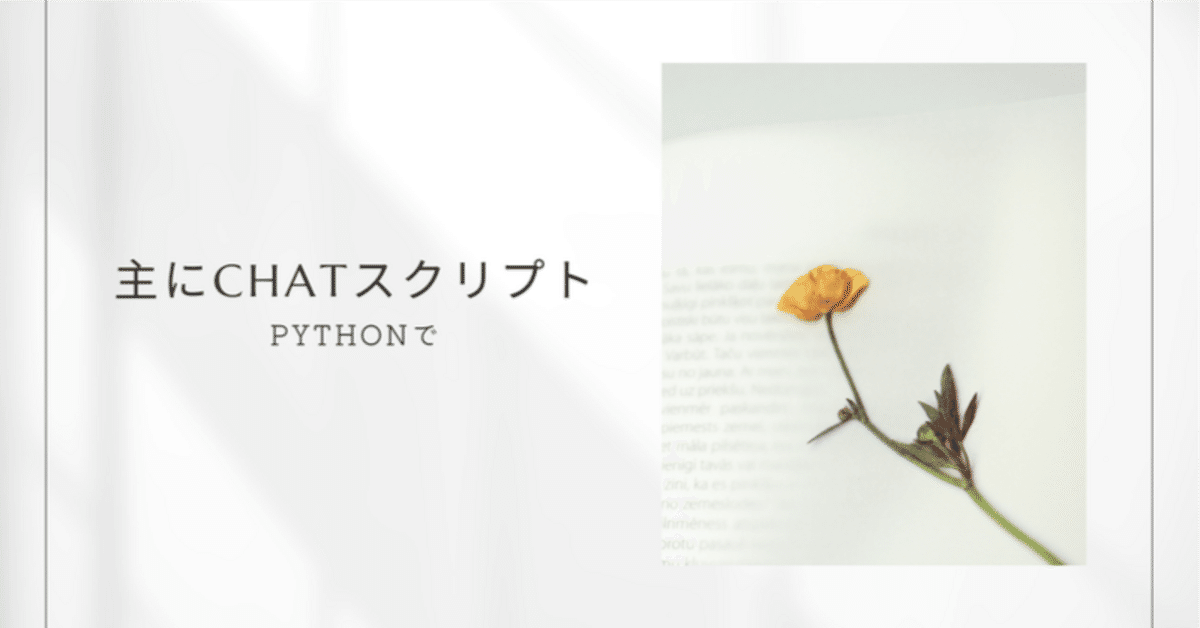
楽天レシピ、x chatgpt。(メンテ)
import json
import os
import requests
import time
import pandas as pd
from pprint import pprint
import openai
# APIキーの設定
openai_key = os.environ.get("OPENAI_API_KEY")
# 引数で質問を受ける
question = input("何が食べたい(レシピなど)ですか? ==> : ")
# AIが使うことができる関数を羅列する
functions = [
{
"name": "get_recipe",
"description": "ランキング上位5位のレシピの情報を得る",
"parameters": {
"type": "object",
"properties": {
# food引数の情報
"a_food": {
"type": "string",
"description": "食べ物の単語です",
},
},
"required": ["food name"],
},
},
]
# 関数の実装
def get_recipe(a_food):
# 1. 楽天レシピのレシピカテゴリ一覧を取得する
print("20秒ほどお待ちください。。。\n")
res = requests.get(
'https://app.rakuten.co.jp/services/api/Recipe/CategoryList/20170426?applicationId=1064593445227237376')
json_data = json.loads(res.text)
parent_dict = {} # mediumカテゴリの親カテゴリの辞書
df = pd.DataFrame(columns=['category1', 'category2', 'category3', 'categoryId', 'categoryName'])
for category in json_data['result']['large']:
df = df._append({'category1': category['categoryId'], 'category2': "", 'category3': "",
'categoryId': category['categoryId'], 'categoryName': category['categoryName']},
ignore_index=True)
for category in json_data['result']['medium']:
df = df._append(
{'category1': category['parentCategoryId'], 'category2': category['categoryId'], 'category3': "",
'categoryId': str(category['parentCategoryId']) + "-" + str(category['categoryId']),
'categoryName': category['categoryName']}, ignore_index=True)
parent_dict[str(category['categoryId'])] = category['parentCategoryId']
for category in json_data['result']['small']:
df = df._append(
{'category1': parent_dict[category['parentCategoryId']], 'category2': category['parentCategoryId'],
'category3': category['categoryId'], 'categoryId': parent_dict[category['parentCategoryId']] + "-" + str(
category['parentCategoryId']) + "-" + str(category['categoryId']),
'categoryName': category['categoryName']}, ignore_index=True)
# 2. キーワードからカテゴリを抽出する
df_keyword = df.query(f'categoryName.str.contains("{a_food}")', engine='python')
if df_keyword.empty:
print("検索結果がありませんでした。")
exit()
# 3. 人気レシピを取得する
df_recipe = pd.DataFrame(
columns=['recipeId', 'recipeTitle', 'foodImageUrl', 'recipeMaterial', 'recipeCost', 'recipeIndication',
'categoryId', 'categoryName'])
for index, row in df_keyword.iterrows():
url = 'https://app.rakuten.co.jp/services/api/Recipe/CategoryRanking/20170426?applicationId=1064593445227237376&categoryId=' + \
row['categoryId']
res = requests.get(url)
json_data = json.loads(res.text)
recipes = json_data['result']
for recipe in recipes[:5]: # 上位4位だけを取得
df_recipe = df_recipe._append({'recipeId': recipe['recipeId'], 'recipeTitle': recipe['recipeTitle'],
'foodImageUrl': recipe['foodImageUrl'],
'recipeMaterial': recipe['recipeMaterial'],
'recipeCost': recipe['recipeCost'],
'recipeIndication': recipe['recipeIndication'],
'categoryId': row['categoryId'], 'categoryName': row['categoryName']},
ignore_index=True)
time.sleep(1) # 連続でアクセスすると先方のサーバに負荷がかかるので少し待つのがマナー
pprint(df_recipe.head(5))
return "{a_food}を使った料理についてコメントする。"
# 1段階目の処理
# AIが質問に対して使う関数と、その時に必要な引数を決める
# 特に関数を使う必要がなければ普通に質問に回答する
client = openai.OpenAI()
response = client.chat.completions.create(
model="gpt-3.5-turbo-0125",
messages=[
{"role": "user", "content": question},
],
functions=functions,
function_call="auto",
)
message = response.choices[0].message
# print(message.content)
if message.function_call:
# 関数を使用すると判断された場合
# 使うと判断された関数名
function_name = message.function_call.name
# その時の引数dict
arguments = json.loads(message.function_call.arguments)
# 2段階目の処理
# 関数の実行
function_response = get_recipe(a_food=arguments["a_food"])
# 3段階目の処理
# 関数実行結果を使ってもう一度質問
second_response = client.chat.completions.create(
model="gpt-3.5-turbo-0125",
messages=[
{"role": "user", "content": question},
message,
{
"role": "function",
"name": function_name,
"content": function_response,
},
],
)
print(second_response.choices[0].message.content)
以前書いた楽天レシピのコードをopenaiのapiの新しい書き方で書き直したので、掲載しておきます。