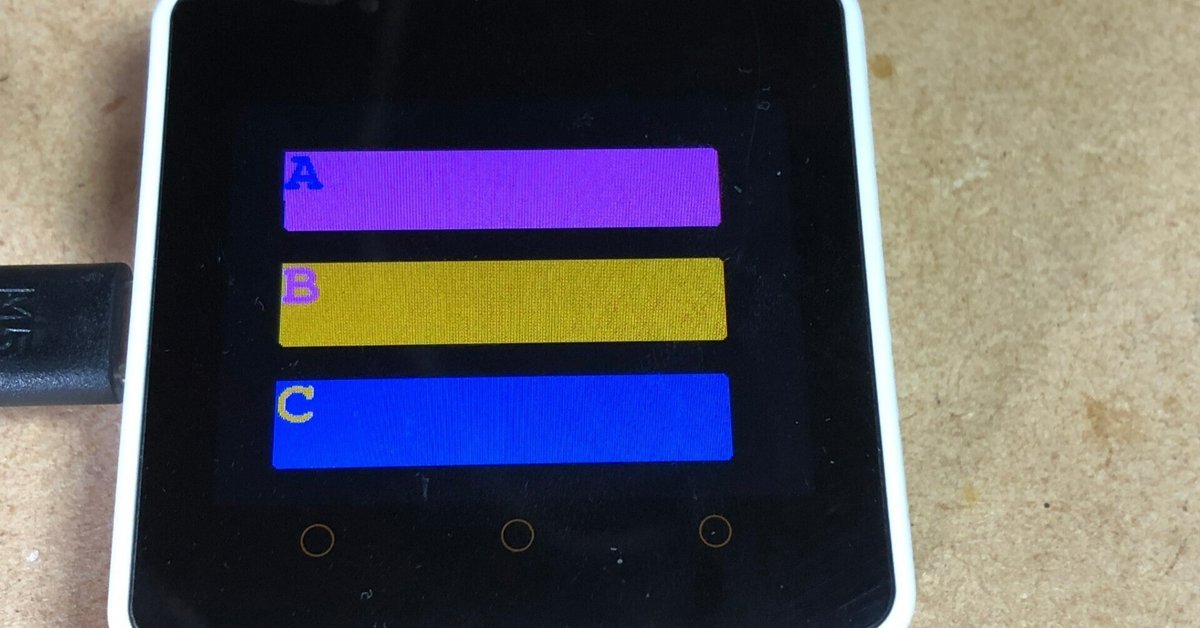
M5Core2 タッチパネルボタン イベント処理
Core2のタッチパネル上にボタンを作りました。
M5Uinfiedライブリーを使ったほうが、後々よさそうですが、
とりあえず、M5Core2ライブラリー+LovyanGFXライブラリーで
やってます。
<<M5Core2ライブラリ タッチパネル>>
コンストラクタ:
Function: Creates rectangular key area instances.
タッチパネル上にボタンエリアを構築。クラスインスタンス化する。
ボタンの描画は、LovyanGFXを使用します。
注:M5APIの記載にはTouchButtonとされていますが、Buttonでないと
コンパイルがとおりませんでした。改変されているみたいです。
Button(uint16_t x_, uint16_t y_, uint16_t w_, uint16_t h_, const char* name_ = "")
Event Listening:
Add key touch handling events and set the type of trigger event.
ボタンが押されたときに呼び出される関数イベントハンドラを登録。
void addHandler(void (*fn)(TouchEvent&), uint16_t eventMask = E_ALL)
Event Types
ボタンの押され方。
注:M5APIの記載には、TE_xxxxとなっていますが、下記のように先頭のTを
取り除くとコンパイルエラーになりませんでした。改変されたみたいです。
#define NUM_EVENTS 8
#define E_TOUCH 0x0001
#define E_RELEASE 0x0002
#define E_MOVE 0x0004
#define E_GESTURE 0x0008
#define E_TAP 0x0010
#define E_DBLTAP 0x0020
#define E_DRAGGED 0x0040
#define E_PRESSED 0x0080
#define E_ALL 0x0FFF
#define E_BTNONLY 0x1000
Get Status
ボタンの状態を取得するメソッド群
Setting Key Status bool setState(bool)
button isPressed isPressed bool isPressed()
button isPressed isRelease bool isReleased()
button isPressed wasPressed bool wasPressed()
button isPressed wasReleased bool wasReleased()
button isPressed pressedFor bool pressedFor(uint32_t ms)
button isPressed pressedFor bool pressedFor(uint32_t ms, uint32_t continuous_time)
button isPressed releasedFor bool releasedFor(uint32_t ms)
button isPressed wasReleasefor bool wasReleasefor(uint32_t ms)
Get attributes
使い方がよくわかりません。
int32_t lastChange()
uint8_t finger;
bool changed;
char name[16];
TouchEvent Structures
液晶にタッチした時に、イベントリスナーに渡される構造体。
When the touch event is triggered, the handler function bound to the region using addHandler is automatically called, and the TouchEvent construct is passed in as a parameter.
struct TouchEvent {
uint8_t finger; //Touch point serial number, maximum support two points
uint16_t type; //Event Types
TouchPoint from; //Event initial touch point coordinates-->x,y
TouchPoint to; //End of event touch point coordinates-->x,y
uint16_t duration; //Event Duration
TouchButton* button; //Event Trigger Object
Gesture* gesture; //Event Trigger Gestures
};
<コード>
#ifndef _MYGRAPHIC_H_
#define _MYGRAPHIC_H_
#include <M5Core2.h>
# define LGFX_USE_V1
#define LGFX_M5STACK_CORE2 // M5Stack M5Stack Core2
#include <LovyanGFX.hpp>
#include <LGFX_AUTODETECT.hpp>
static LGFX lcd; // LGFXのインスタンスを作成。
static LGFX_Sprite sprite(&lcd); // スプライトを使う場合はLGFX_Spriteのインスタンスを作成。
typedef struct
{
int x;
int y;
int32_t color;
}_Point;
typedef struct
{
_Point pt0;
_Point pt1;
uint32_t color;
}_AxisPt;
typedef struct
{
_Point Pt0;
_Point Pt1;
uint32_t color;
}_LinePt;
#endif
#include <M5Core2.h>
#include "myGraphic.h"
/// @brief タッチイベント構造体表示
/// @param e
void Write_TouchEventStructures(Event& e);
/// @brief タッチボタンクラス インスタンス
Button topButton(30, 30, 250, 50,false,"top");
Button middleButton(30, 30+50+20, 250, 50,false, "middle");
Button bottomButton(30, 30+50*2+20*2, 250, 50,false, "bottom");
/// @brief タッチイベントハンドラ top, middle
/// @param e
void TouchButtonEvent(Event& e)
{
int color;
Write_TouchEventStructures(e);//タッチイベント構造体内容表示
Button& b = *e.button; //タッチボタンを取り出し。
if(b == topButton)//topボタンの処理
{
lcd.fillRoundRect (b.x, b.y, b.w, b.h,3, MAGENTA); // 角丸の矩形の塗り
color=b.isPressed() ? 0xFC9F : MAGENTA;
lcd.fillRoundRect(b.x, b.y, b.w, b.h,3, color);
lcd.setCursor(30,30);
lcd.setTextColor(BLUE,color);
lcd.write("A");
}
if(b == middleButton)//middleボタンの処理
{
lcd.fillRoundRect (b.x, b.y, b.w, b.h,3, ORANGE); // 角丸の矩形の塗り
color = b.isPressed() ? YELLOW : ORANGE;
lcd.fillRoundRect(b.x, b.y, b.w, b.h,3, color);
lcd.setCursor(30,30+50+20);
lcd.setTextColor(BLUE,color);
lcd.write("B");
}
}
/// @brief タッチイベントハンドラ bottomボタンの処理
/// @param e タッチイベント構造体
void bottomTapped(Event& e)
{
Button b = *e.button;
static int color=BLUE;
Write_TouchEventStructures(e);//タッチイベント構造体内容表示
Serial.println("BUTTON WAS DOUBLETAPPED");
Serial.println("");
lcd.fillRoundRect (b.x, b.y, b.w, b.h,3, BLUE); // 角丸の矩形の塗り
color=(color==BLUE) ? CYAN : BLUE;
lcd.fillRoundRect(b.x, b.y, b.w, b.h,3, color);
lcd.setCursor(30,30+50*2+20*2);
lcd.setTextColor(ORANGE,color);
lcd.write("C");
}
void setup()
{
M5.begin(true,false,true,false,kMBusModeOutput,false);
// 最初に初期化関数を呼び出します。
lcd.init();
// 回転方向を 0~3 の4方向から設定します。(4~7を使用すると上下反転になります。)
lcd.setRotation(1);
// バックライトの輝度を 0~255 の範囲で設定します。
lcd.setBrightness(128);
// RGB888の24ビットに設定(表示される色数はパネル性能によりRGB666の18ビットになります)
lcd.setColorDepth(24);
//フォント
lcd.setFont(&fonts::FreeMonoBold18pt7b);
lcd.setTextSize(1);
//Topボタン描画
lcd.fillRoundRect ( 30, 30, 250, 50, 3, PINK); // 角丸の矩形の塗り
lcd.setCursor(30,30);
lcd.setTextColor(BLUE,PINK);
lcd.write("A");
//middleボタン描画
lcd.fillRoundRect ( 30, 30+50+20, 250, 50, 3, ORANGE); // 角丸の矩形の塗り
lcd.setCursor(30,30+50+20);
lcd.setTextColor(PINK,ORANGE);
lcd.write("B");
//bottomボタン描画
lcd.fillRoundRect ( 30, 30+50*2+20*2, 250, 50, 3, BLUE); // 角丸の矩形の塗り
lcd.setCursor(30,30+50*2+20*2);
lcd.setTextColor(ORANGE,BLUE);
lcd.write("C");
//タッチイベントハンドラ登録
topButton.addHandler(TouchButtonEvent, E_TOUCH | E_RELEASE);
middleButton.addHandler(TouchButtonEvent, E_TOUCH | E_RELEASE);
bottomButton.addHandler(bottomTapped, E_DBLTAP);
}
void loop()
{
M5.update();
delay(1);
}
/**
* タッチイベント発生時の構造体を表示。
* @param e タッチイベント構造体
*/
void Write_TouchEventStructures(Event& e)
{
Serial.println("-------Touch Event occered--------");
Serial.printf("button name:%-10s\n",e.button->getName());
Serial.printf("%-12s finger%d from(%3d, %3d)",e.typeName(), e.finger, e.from.x, e.from.y);
if (e.type != E_TOUCH && e.type != E_TAP && e.type != E_DBLTAP) {
Serial.printf("--> (%3d, %3d) %5d ms", e.to.x, e.to.y, e.duration);
}
Serial.println();
Serial.println();
}