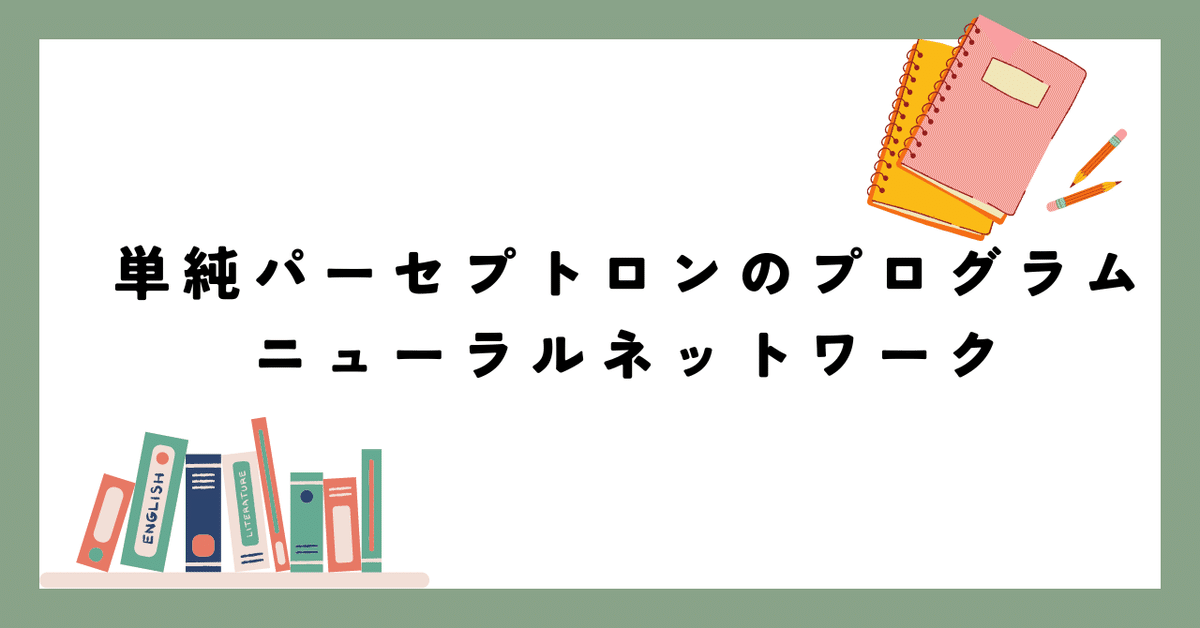
単純パーセプトロン ニューラルネットワーク
プログラム
import numpy as np
import matplotlib.pyplot as plt
# 単純パーセプトロン
class SimplePerceptron(object):
# 重みとバイアスの初期化
def __init__(self, input_dim):
self.input_dim = input_dim
self.w = np.random.normal(size=(input_dim,))
self.b = 0.
# モデルの出力
def forward(self, x):
y = step(np.matmul(self.w,x)+self.b)
return y
# 誤差の計算
def compute_deltas(self, x, t):
y = self.forward(x)
delta = y - t
dw = delta * x
db = delta
return dw, db
# ステップ関数
def step(x):
return 1 * (x>0)
# 乱数シードの固定
np.random.seed(123)
# データの準備
d = 2 # 入力次元
N = 20 # 全データ数
mean = 5 # データ平均値
# 入力
x1 = np.random.randn(N//2, d)+np.array([0, 0])
x2 = np.random.randn(N//2, d)+np.array([mean, mean])
x = np.concatenate((x1, x2), axis=0)
# 出力
t1 = np.zeros(N//2)
t2 = np.ones(N//2)
t = np.concatenate((t1, t2))
# モデルの構築
model = SimplePerceptron(input_dim=d)
# モデルの学習
def compute_loss(dw, db):
# 誤差があるかどうかを判定
return all(dw == 0) * (db == 0)
def train_step(x, t):
# 与えられたデータによりパラメータ更新
dw, db = model.compute_deltas(x,t)
loss = compute_loss(dw, db)
model.w = model.w - dw
model.b = model.b - db
return loss
while True:
classified = True
for i in range(N):
loss = train_step(x[i], t[i])
classified *= loss
# 全データが正しく分類されたら終了
if classified:
break
# モデルの評価
print('w:', model.w)
print('b:', model.b)
# 結果のプロット
plt.figure()
plt.scatter(x1[:,0],x1[:,1])
plt.scatter(x2[:,0],x2[:,1])
# 重みとバイアスから境界線をプロット
x1t = np.linspace(0,8,30)
x2t = (-model.w[0]*x1-model.b)/model.w[1]
x2t1 = (-model.w[0]*x1t-model.b)/model.w[1]
plt.plot(x1,x2t, color="blue")
plt.plot(x1t,x2t1, color="blue")
# 目盛りを内側に設定
plt.tick_params(axis='both', direction='in') # 目盛りの長さも指定可能
plt.grid()
plt.show()
関連記事
サイト
https://sites.google.com/view/elemagscience/%E3%83%9B%E3%83%BC%E3%83%A0