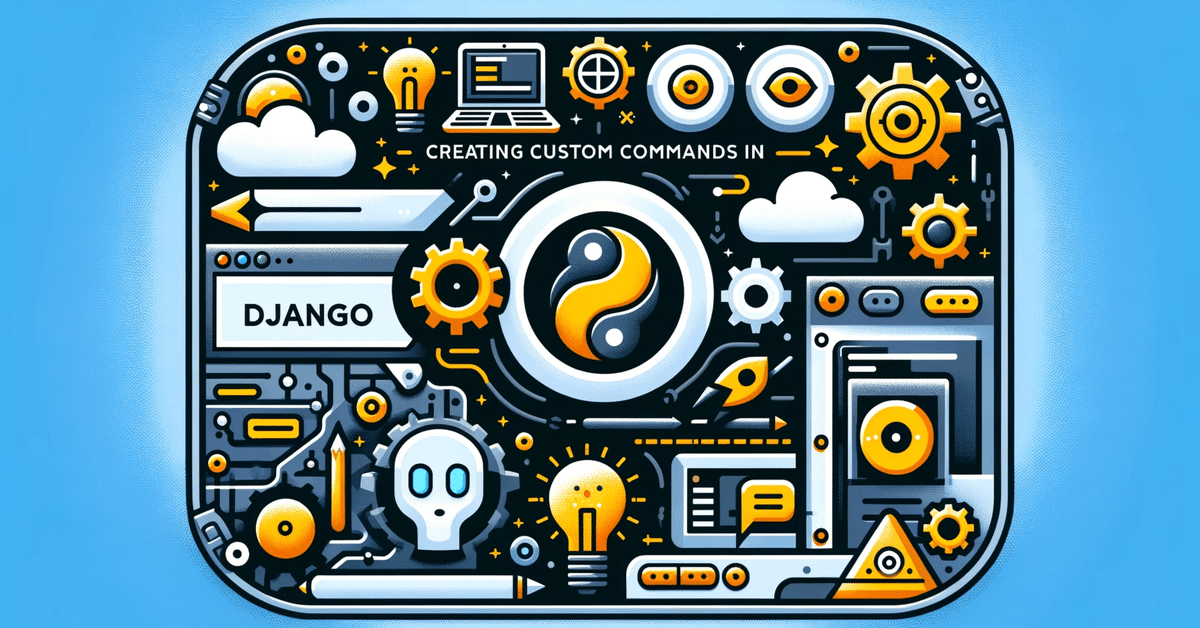
Creating Custom Commands in Django
As a note, I will summarize below the method for creating your own custom commands in Django.
Create app_name/management/commands Directory
create management directory and create __init__py file and commands directory in it.
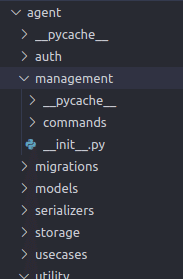
Create Custom Command file in commands directory
Create original command in commands directory.
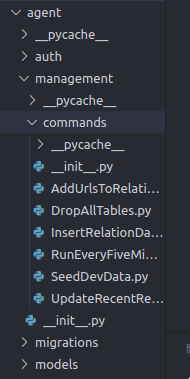
My custom command
# DropAllTables.py
from django.db import connection
from django.core.management.base import BaseCommand
class Command(BaseCommand):
help = "Drop all tables command"
def handle(self, *args, **options):
cursor = connection.cursor()
cursor.execute("DROP SCHEMA public CASCADE;")
cursor.execute("CREATE SCHEMA public;")
cursor.execute("GRANT ALL ON SCHEMA public TO user_name;")
You can use command like this.
$ python manage.py DropAllTables
It's also possible to accept arguments.
You can accept command line arguments like this.
# DropSpecificTable.py
class Command(BaseCommand):
help = "Delete specific table"
def handle(self, *args, **options):
table_name = options['table_name']
cursor = connection.cursor()
cursor.execute("DROP table " + table_name + ";")
$ python manage.py DropSpecificTable --table_name=specific_table_name