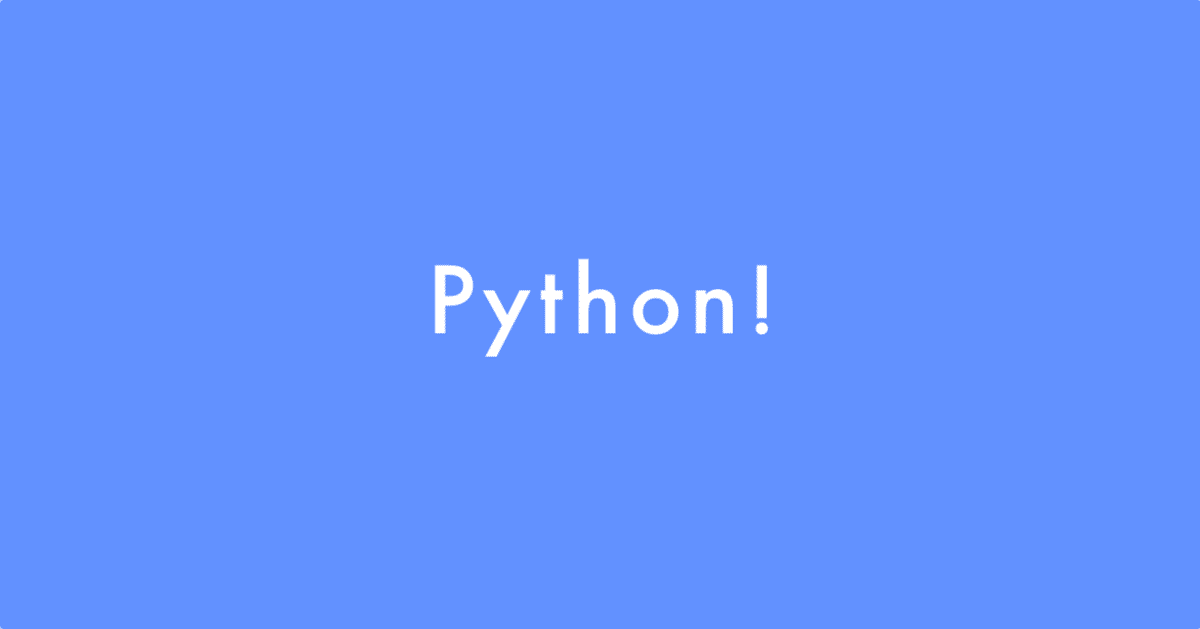
Pythonでプログラミング - Thread(マルチスレッド処理)
モジュールthreadingを使って並列処理(マルチスレッド処理)させるタイマーを作ります。
threadingの公式サイト
Thread オブジェクト
何回か決めて実行させる。関数を定義し、その関数をThreadで使います。引数も利用できます。
import threading
import time
targetTime = 3
targetTime2 = 6
def countUpTimer(target):
for i in range(1 ,target):
print(i)
time.sleep(1)
print("終わり!")
timer1 = threading.Thread(target=countUpTimer,args=(targetTime,))
timer2 = threading.Thread(target=countUpTimer,args=(targetTime2,))
timer1.start()
time.sleep(1)
timer2.start()
Timer オブジェクト
threading.Timerのメソッドのみ使ってスタート、ストップすることが出来ます。シンプルに実装できそうです。
import threading
import time
def hello():
global t
print("Hello")
t = threading.Timer(1,hello)
t.start()
hello()
time.sleep(3)
t.cancel()
t = threading.Timer(1,hello)
ということでタイマーを変数"t"を作って、スタート、キャンセルなど操作していくます。
Event オブジェクト
クラスを作って実行します。関数でrun(self)、 stop(self)を定義するのでスタート、ストップがわかり易いです。
import threading
import time
class TimerClass(threading.Thread):
def __init__(self):
threading.Thread.__init__(self)
self.event = threading.Event()
self.count = 10
def run(self):
while self.count > 0 and not self.event.is_set():
print(self.count)
self.count -= 1
self.event.wait(1)
def stop(self):
self.event.set()
tmr = TimerClass()
tmr.start()
time.sleep(3)
tmr.stop()
例外処理を設置する場合。