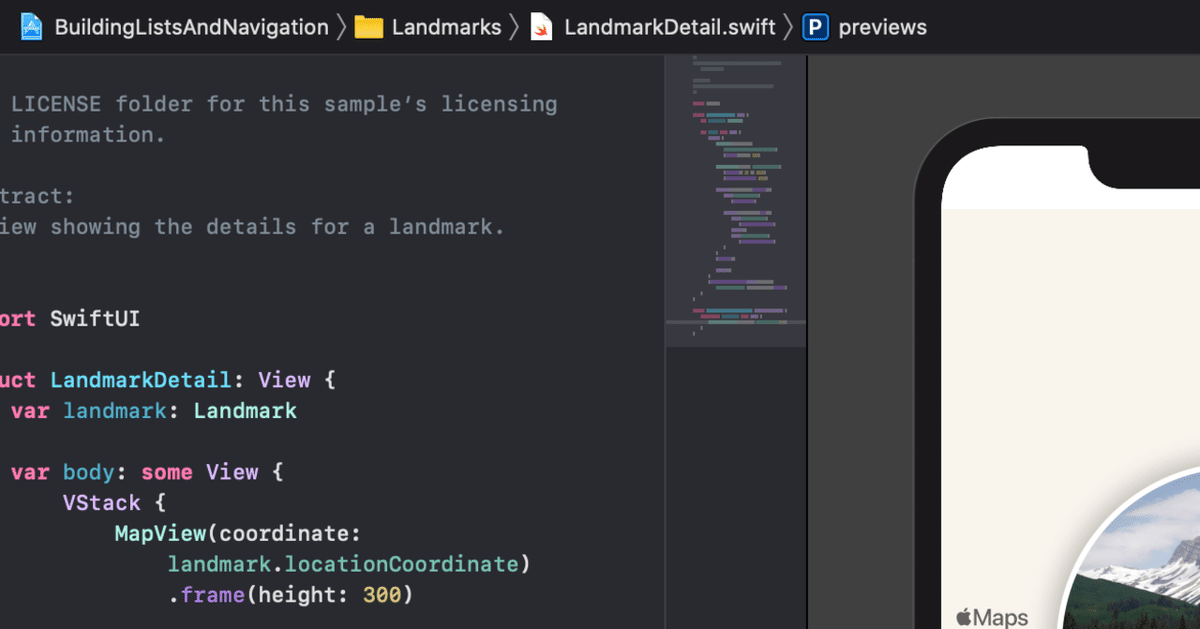
SWiftUIでいこう! - Timer.publish処理 スタート、ストップ
How to use a timer with SwiftUIとCustom Timer With Background Fetch Using SwiftUI - StopWatch Using SwiftUI - SwiftUI Tutorialsを参考に
単純なタイマーを作ります。XcodeのPlaygroundで実行できるようにします。基本的な形は以下でSwiftUIで組んでいきます。表示されるのはボタンのみ、ボタンを押せばコンソールにカウントダウンの数字が出てくるようにします。
import SwiftUI
import PlaygroundSupport
struct TimerView: View {
var body: some View {
Button(action: {
}){
Text("Button")
}
.onReceive(timer) { _ in
}
}
}
PlaygroundPage.current.setLiveView(TimerView())
変数は以下3つ
@State var timeRemaining = 10 ・・・ 残り時間
@State var start = false ボタンの状態を保存
とタイマーの心臓部分です。Timer.publish、と.onReceive()で実行させます。
let timer = Timer.publish(every: 1, on: .main, in: .common).autoconnect()
あとはボタンの実装、.onReceive()で場合わけでカウントダウンとストップの命令を書いて行きます。
Button(action: {
start.toggle()
print(start)
}){
Text("Button")
}
ボタンを押すことで
start.toggle()
最初が falseなのでボタンを押すことでtrueとしてやります。
print(start)
としているのは変数startの状態を見るためです。そして表示部分は
Text("Button")
実行するとButtonと表示されます。そしてボタンを押したあとの実行部分ですが、
.onReceive(timer) { _ in
if start {
if timeRemaining != 0{
timeRemaining -= 1
print( timeRemaining)
}else{
start.toggle()
timeRemaining = 10
}
}
}
としました。まず、場合わけです。
if start { 実行内容 }
変数startがtrueの場合の実行内容を書いています。
import SwiftUI
import PlaygroundSupport
struct TimerView: View {
@State var timeRemaining = 10
@State var start = false
let timer = Timer.publish(every: 1, on: .main, in: .common).autoconnect()
var body: some View {
Button(action: {
start.toggle()
print(start)
}){
Text("Button")
}
.onReceive(timer) { _ in
if start {
if timeRemaining != 0{
timeRemaining -= 1
print( timeRemaining)
}else{
start.toggle()
timeRemaining = 10
}
}
}
}
}
PlaygroundPage.current.setLiveView(TimerView())