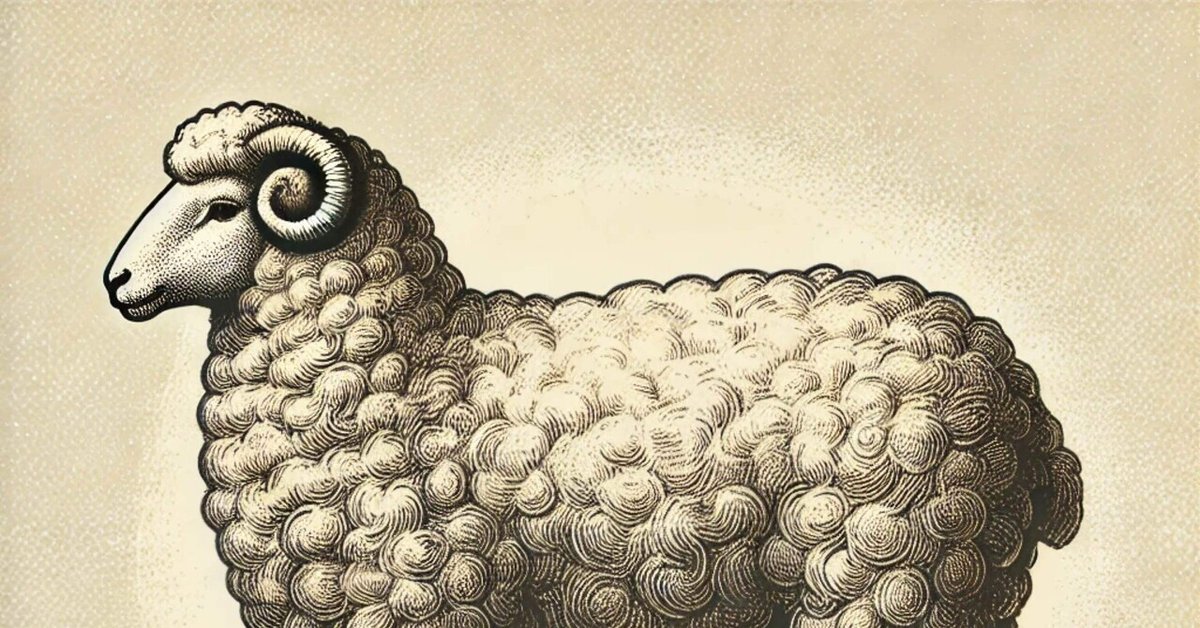
Node.jsで棒倒し法による迷路作成プログラム
棒倒し法を使用して迷路を作成するプログラムをNode.jsで実装します。この手法では、壁で埋めたグリッドにランダムな通路を追加して迷路を生成します。
1. 棒倒し法の概要
棒倒し法のステップは次のとおりです。
壁で埋められたグリッドを用意します。
任意の位置からランダムな方向に「棒」を倒すことで通路を作成します。
特徴
ランダムに選択した方向に1マス分壁を壊すため、迷路に規則性が少なくなります。
2. 環境構築
Node.jsの環境構築は、こちらを確認してください。
3. プログラム実装
maze.jsという名前で以下のコードを保存してください。
class Maze {
constructor(width, height) {
this.width = width;
this.height = height;
this.grid = Array.from({ length: height }, () => Array(width).fill("#"));
}
generate() {
for (let y = 1; y < this.height; y += 2) {
for (let x = 1; x < this.width; x += 2) {
this.grid[y][x] = " ";
this.knockDownWall(x, y);
}
}
// スタート地点とゴール地点を設定
this.grid[1][1] = "☆"; // スタート地点
this.grid[this.height - 2][this.width - 2] = "☆"; // ゴール地点
}
knockDownWall(x, y) {
const directions = [];
if (x > 1) directions.push([-1, 0]); // 左
if (x < this.width - 2) directions.push([1, 0]); // 右
if (y > 1) directions.push([0, -1]); // 上
if (y < this.height - 2) directions.push([0, 1]); // 下
if (directions.length > 0) {
const [dx, dy] =
directions[Math.floor(Math.random() * directions.length)];
this.grid[y + dy][x + dx] = " ";
}
}
print() {
this.grid.forEach((row) => console.log(row.join("")));
}
}
const maze = new Maze(21, 21); // 幅と高さは奇数
maze.generate();
maze.print();
4. 実行
以下のコマンドを使用してプログラムを実行します。
node maze.js
コンソールに生成された迷路が出力されます。
実行するたびに新しい迷路が生まれます。
#####################
#☆ # # # #
# # # ####### # # ###
# # # # # # #
# # ### # ### ### ###
# # # # # # # # #
# # # # ####### # # #
# # # # # # # #
### ##### ######### #
# # # # # # # #
# ##### ######### ###
# # # # # #
##### # ##### ### # #
# # # # # #
####### ##### ##### #
# # # # # # #
# # ####### ##### # #
# # # # # #
# # ### ### # #######
# # # # ☆#
#####################
まとめ
Node.jsの実装を通じて、棒倒し法のシンプルなアルゴリズムを紹介しました。コードの応用や改良を試してみてください。