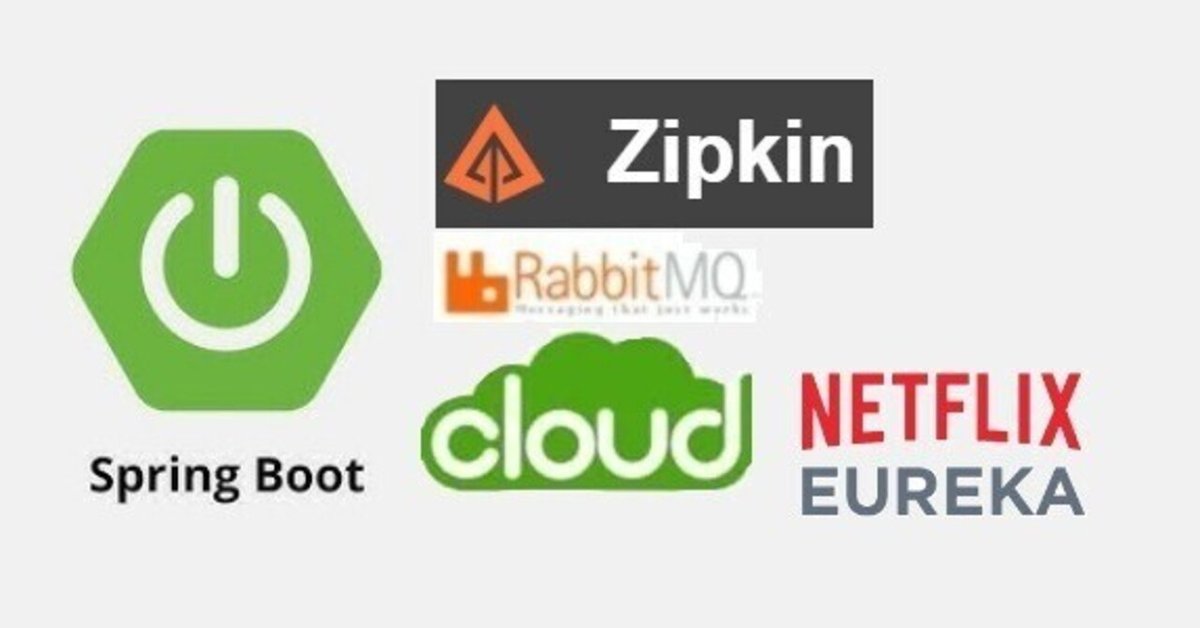
【マイクロサービスの拡張実装】API構築から有機的結合まで - Springboot3 第9回
1. はじめに
こんにちは、今日は既存のマイクロサービスに一つのサービスを追加して、マイクロサービスがどうやって拡張されるのか、勉強・実習します!
今まで勉強したマイクロサービスの要素を全部復習します。
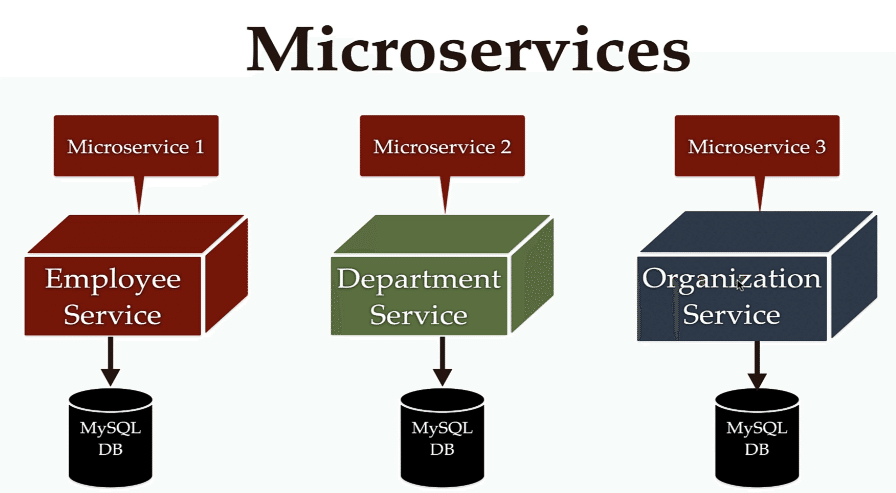
2. API構築過程
サービス作成とDB設定
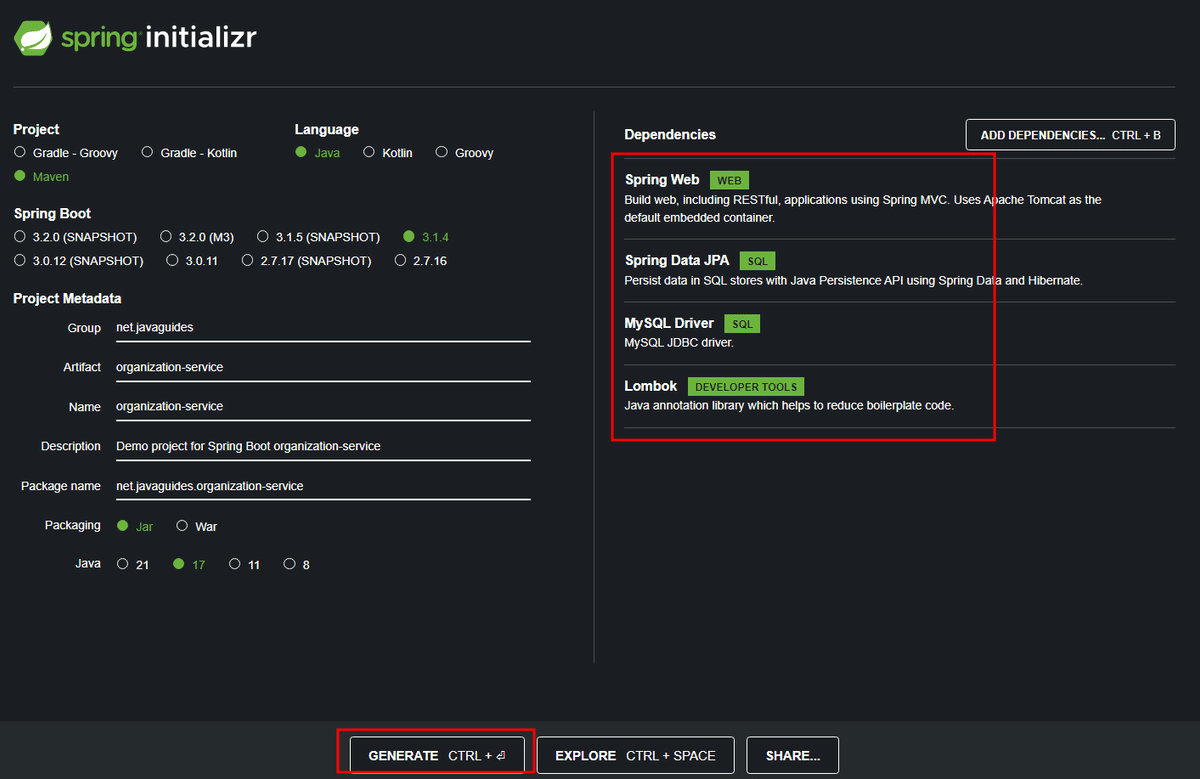
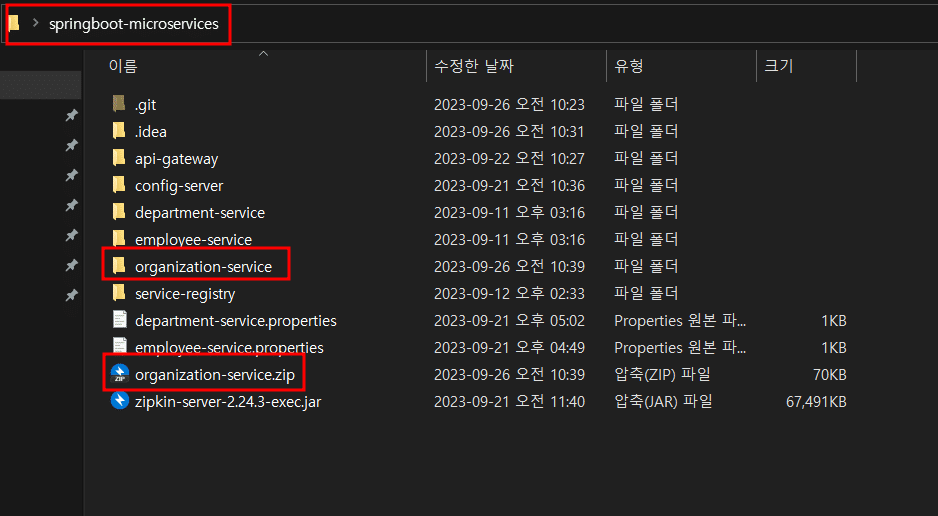
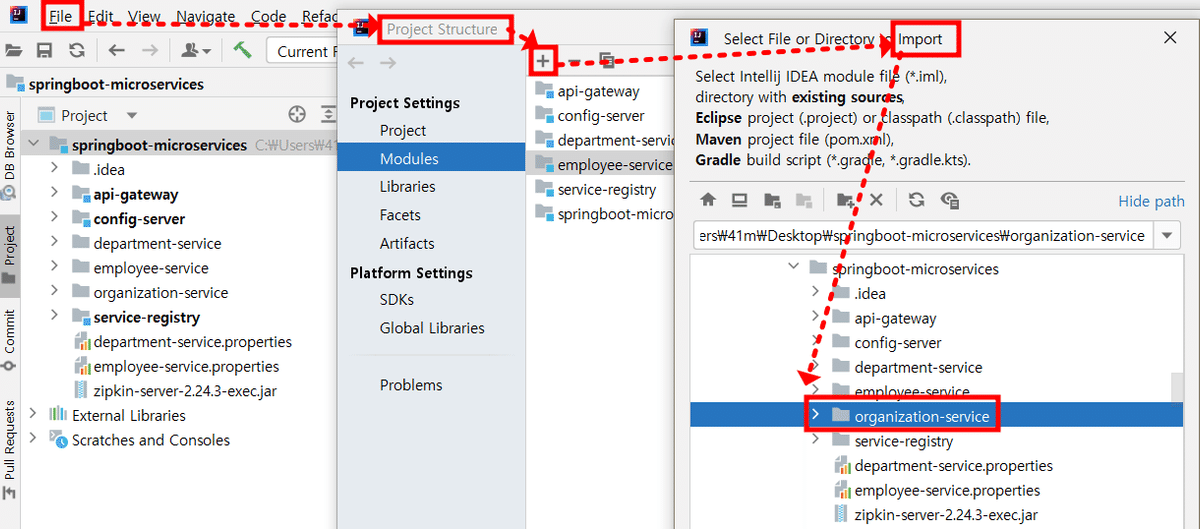
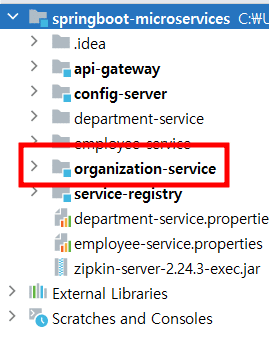
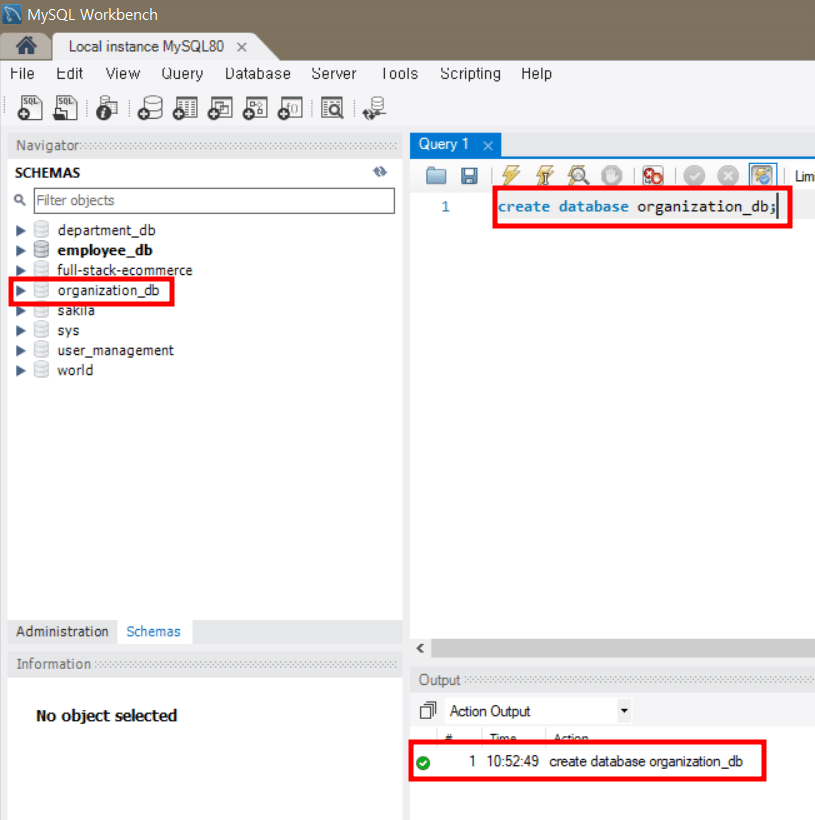
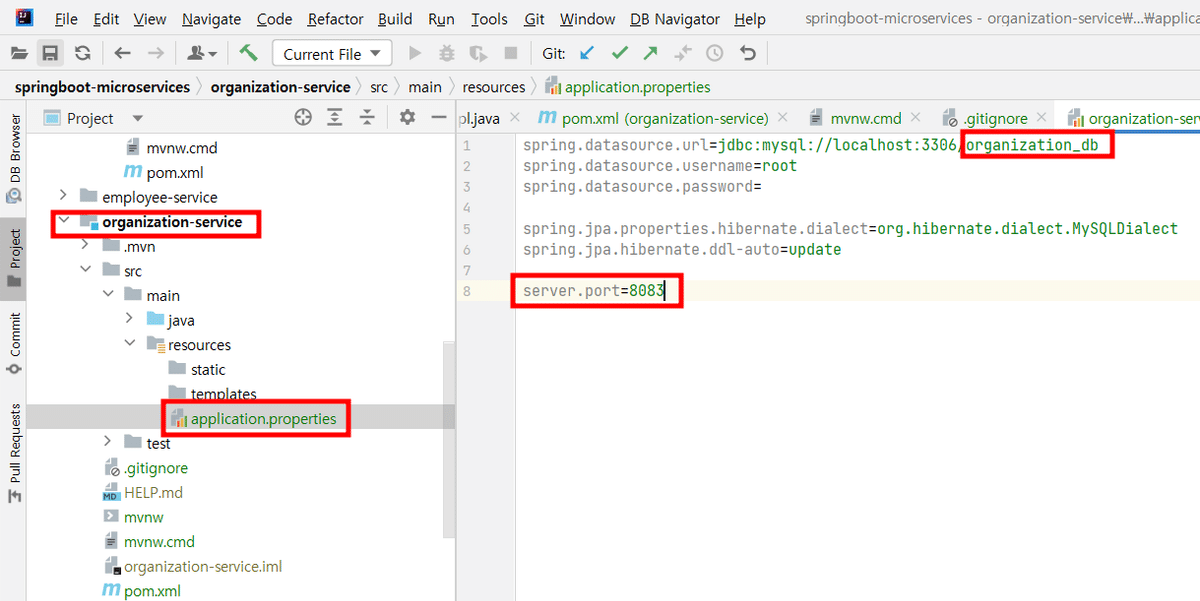
spring.datasource.url=jdbc:mysql://localhost:3306/department_db
spring.datasource.username=root
spring.datasource.passwod=自分の暗号
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQLDialect
spring.jpa.hibernate.ddl-auto=update
server.port=8083
JPA EntityとJPA Repositoryの作成
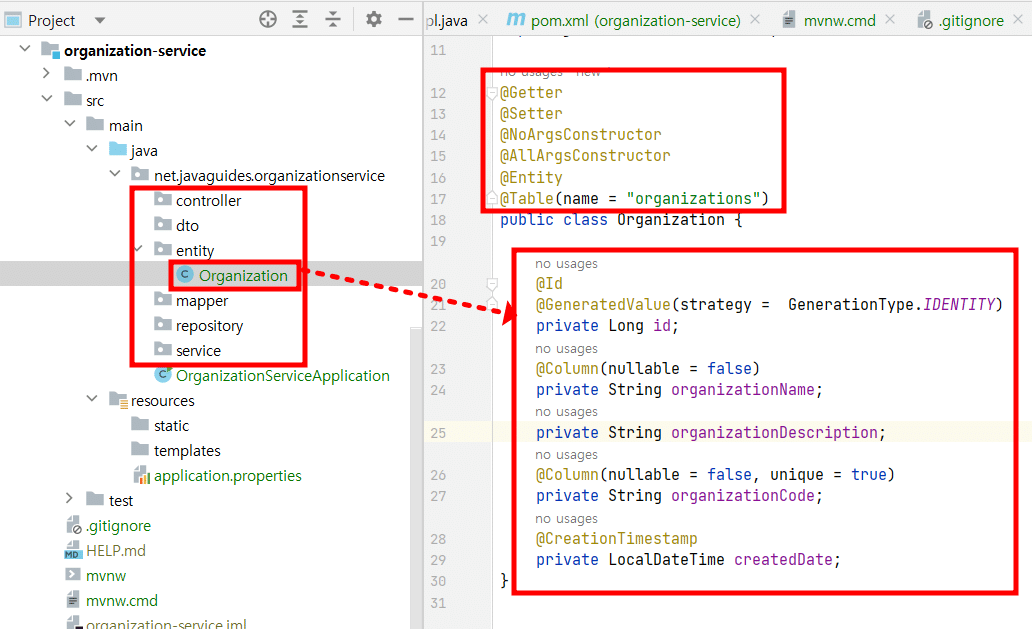
2. Organizationエンティティを作成し、アノテーションとテーブルやフィールドを定義するコードを入力します。
@Getter
@Setter
@NoArgsConstructor
@AllArgsConstructor
@Entity
@Table(name = "organizations")
public class Organization {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String organizationName;
private String organizationDescription;
@Column(nullable = false, unique = true)
private String organizationCode;
@CreationTimestamp
private LocalDateTime createdDate;
}
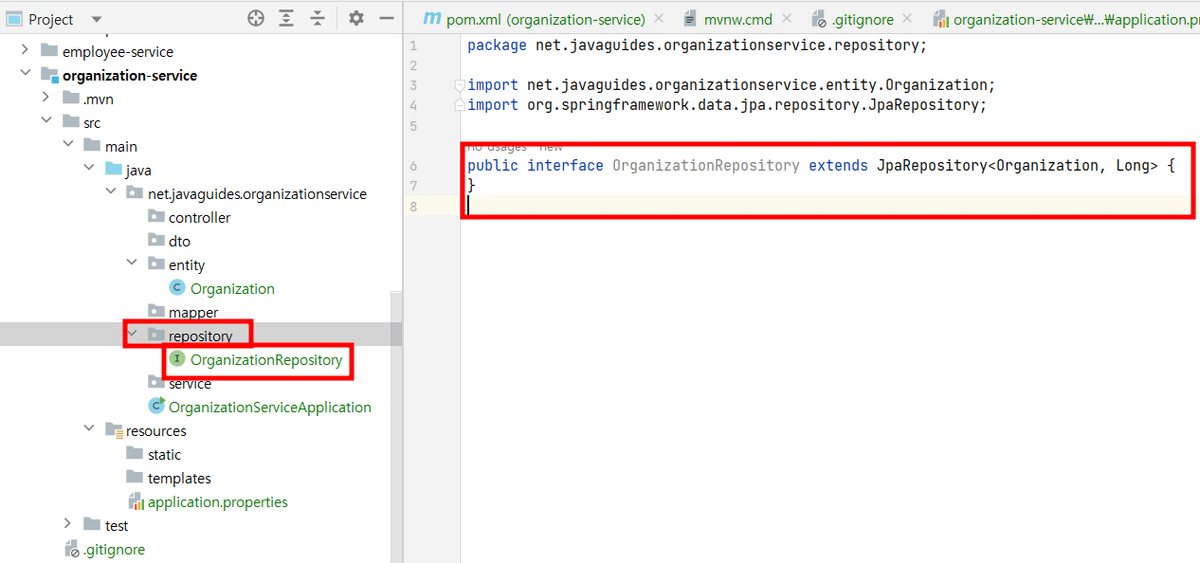
public interface OrganizationRepository extends JpaRepository<Organization, Long> {
}
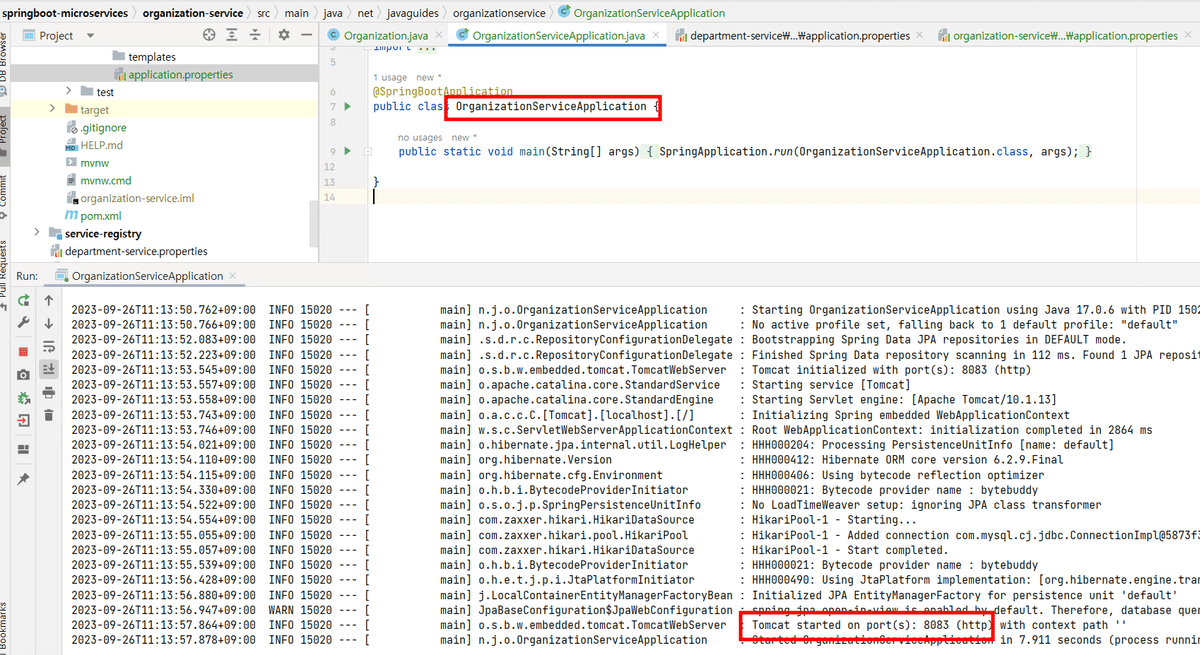
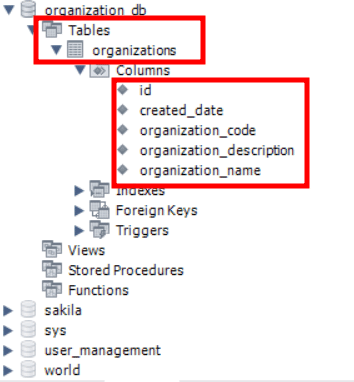
テーブルとカラムがうまく生成されたことが確認できます。
DTOとMapperの生成
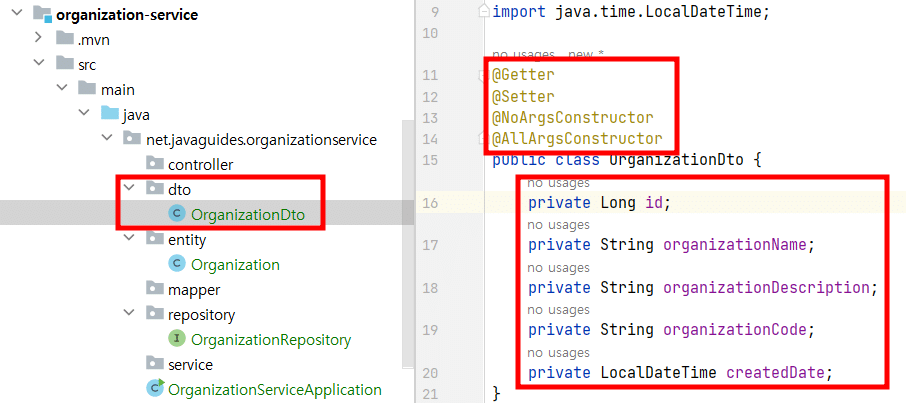
Lombokのアノテーションを追加します。
Orgaizationにある同じフィールドを追加します。
@Getter
@Setter
@NoArgsConstructor
@AllArgsConstructor
public class OrganizationDto {
private Long id;
private String organizationName;
private String organizationDescription;
private String organizationCode;
private LocalDateTime createdDate;
}
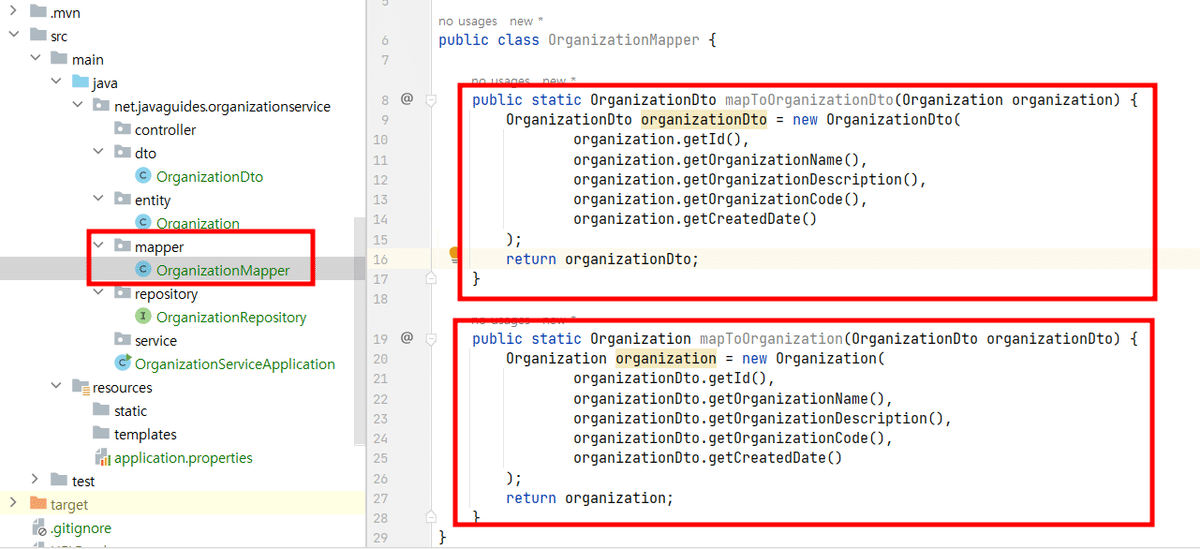
OrgaizationをOrgaizationDtoに、
OrgaizationDtoをOrganizationに変えるコードを作成します。
public class OrganizationMapper {
public static OrganizationDto mapToOrganizationDto(Organization organization) {
OrganizationDto organizationDto = new OrganizationDto(
organization.getId(),
organization.getOrganizationName(),
organization.getOrganizationDescription(),
organization.getOrganizationCode(),
organization.getCreatedDate()
);
return organizationDto;
}
public static Organization mapToOrganization(OrganizationDto organizationDto) {
Organization organization = new Organization(
organizationDto.getId(),
organizationDto.getOrganizationName(),
organizationDto.getOrganizationDescription(),
organizationDto.getOrganizationCode(),
organizationDto.getCreatedDate()
);
return organization;
}
}
REST API(登録・照会)
1.登録
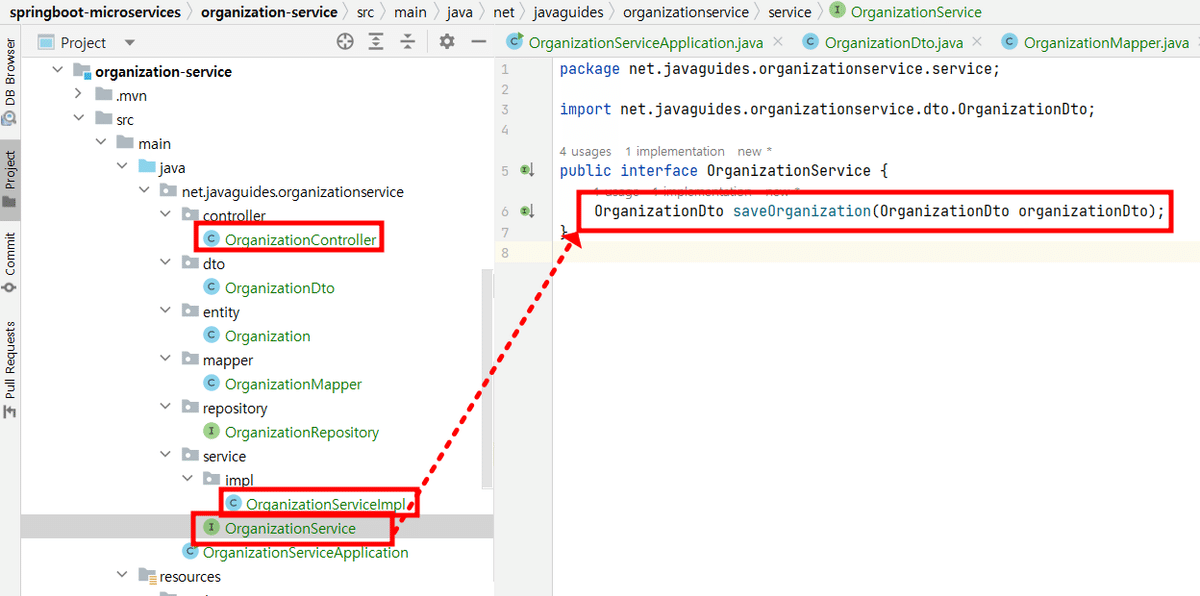
public interface OrganizationService {
OrganizationDto saveOrganization(OrganizationDto organizationDto);
}
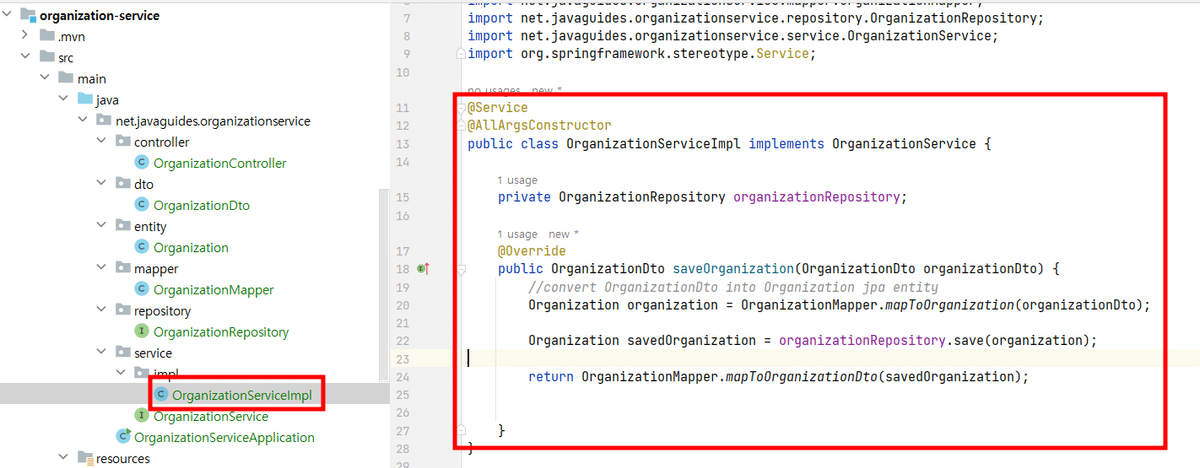
@Service
@AllArgsConstructor
public class OrganizationServiceImpl implements OrganizationService {
private OrganizationRepository organizationRepository;
@Override
public OrganizationDto saveOrganization(OrganizationDto organizationDto) {
//convert OrganizationDto into Organization jpa entity
Organization organization = OrganizationMapper.mapToOrganization(organizationDto);
Organization savedOrganization = organizationRepository.save(organization);
return OrganizationMapper.mapToOrganizationDto(savedOrganization);
}
}
注意すべき点は、OrganizationServiceImplクラスに@AllArgsConstructorアノテーションを付けないと、OrganizationRepositoryが使えなくなるので、必ず忘れずに入力することです。
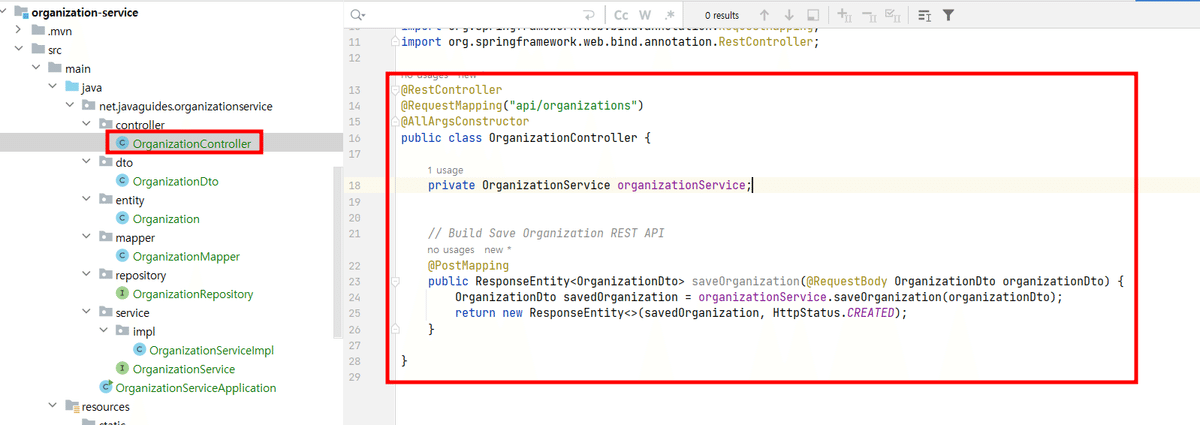
@RestController
@RequestMapping("api/organizations")
@AllArgsConstructor
public class OrganizationController {
private OrganizationService organizationService;
// Build Save Organization REST API
@PostMapping
public ResponseEntity<OrganizationDto> saveOrganization(@RequestBody OrganizationDto organizationDto) {
OrganizationDto savedOrganization = organizationService.saveOrganization(organizationDto);
return new ResponseEntity<>(savedOrganization, HttpStatus.CREATED);
}
}
ここにもOrganizationControllerクラスに@AllArgsConstructorアノテーションを付けないとOrganizationServiceが使えなくなるので、必ず忘れずに入力する。
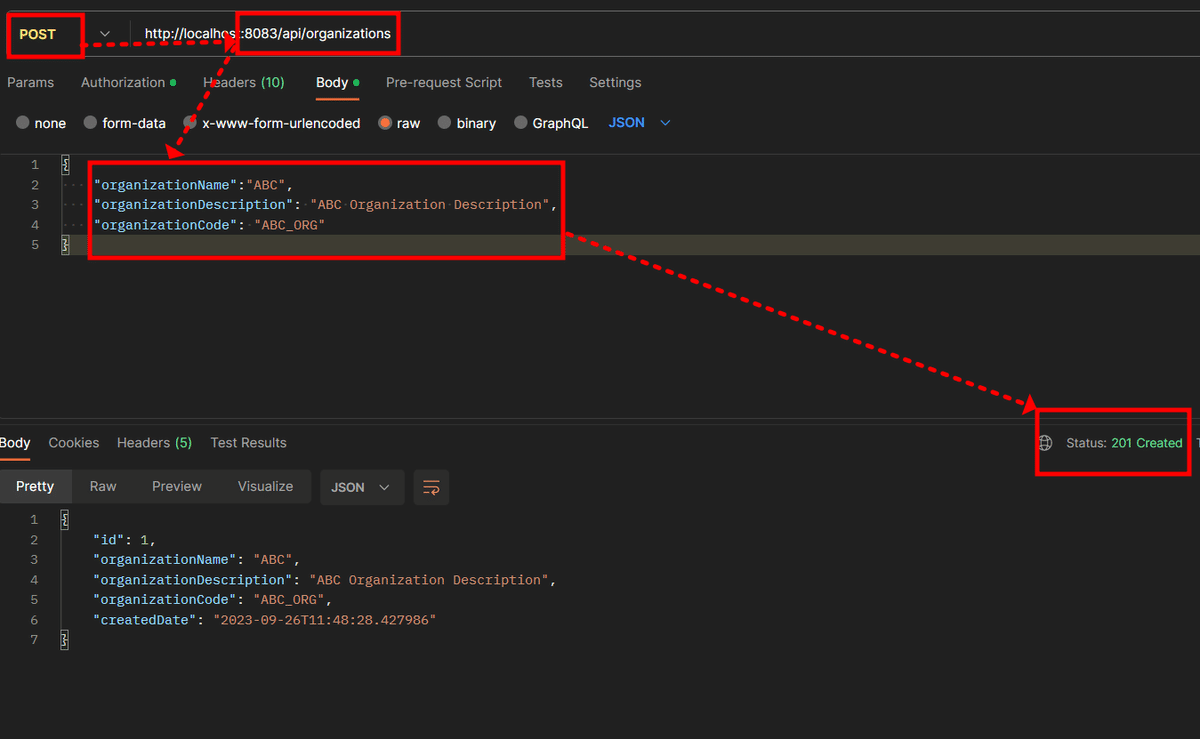
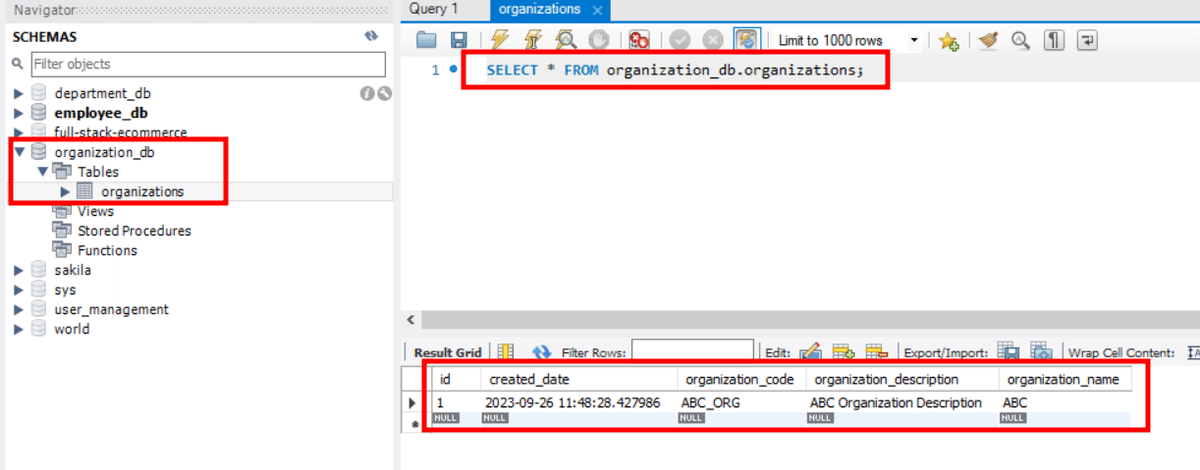
2.照会
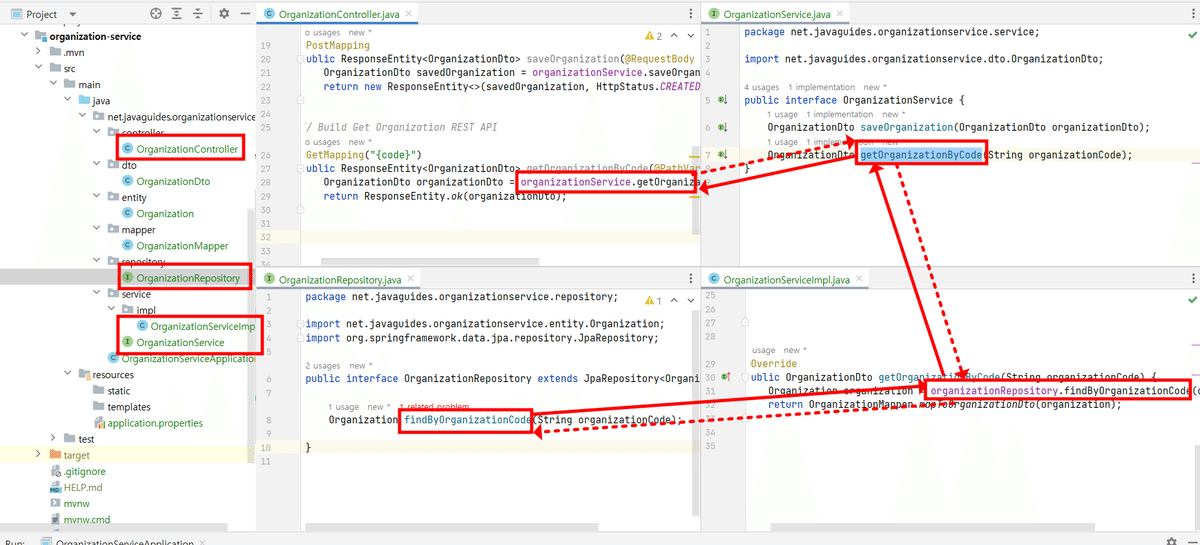
OrganizationRepository
public interface OrganizationRepository extends JpaRepository<Organization, Long> {
Organization findByOrganizationCode(String organizationCode);
}
OrganizationServcie
OrganizationDto getOrganizationByCode(String organizationCode);
OrganizationServcieImpl
@Override
public OrganizationDto getOrganizationByCode(String organizationCode) {
Organization organization = organizationRepository.findByOrganizationCode(organizationCode);
return OrganizationMapper.mapToOrganizationDto(organization);
}
OrganizationController
// Build Get Organization REST API
@GetMapping("{code}")
public ResponseEntity<OrganizationDto> getOrganizationByCode(@PathVariable("code") String organizationCode) {
OrganizationDto organizationDto = organizationService.getOrganizationByCode(organizationCode);
return ResponseEntity.ok(organizationDto);
}
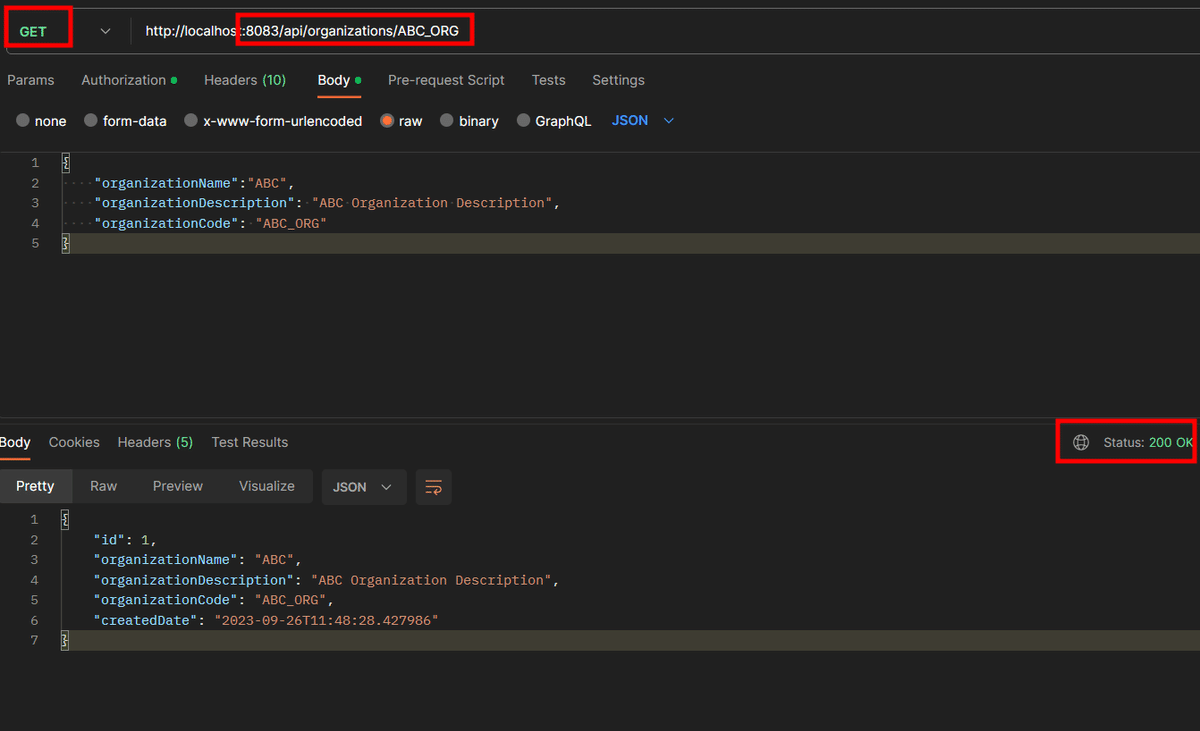
employee-serviceからREST API呼び出し
もし、クライアントがEmployeeがorganizationに属し、employeeが唯一のorganization codeを持つことを要求したらどうしたらいいでしょうか。
Get Employee APIをorganizationと一緒にemployeeを返すように修正する必要があります。
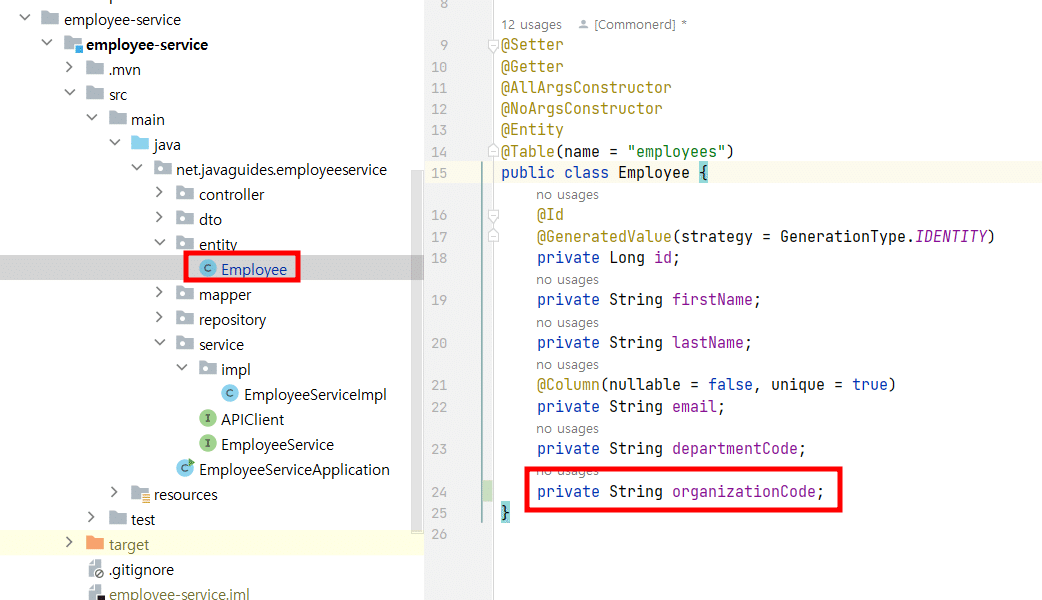
@Setter
@Getter
@AllArgsConstructor
@NoArgsConstructor
@Entity
@Table(name = "employees")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String firstName;
private String lastName;
@Column(nullable = false, unique = true)
private String email;
private String departmentCode;
private String organizationCode;
}
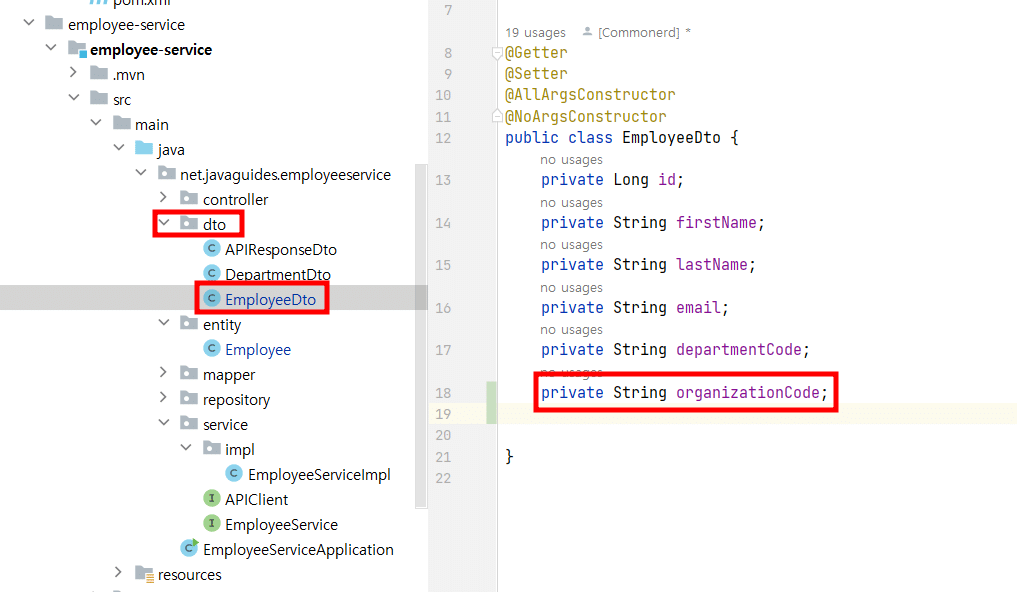
@Getter
@Setter
@AllArgsConstructor
@NoArgsConstructor
public class EmployeeDto {
private Long id;
private String firstName;
private String lastName;
private String email;
private String departmentCode;
private String organizationCode;
}
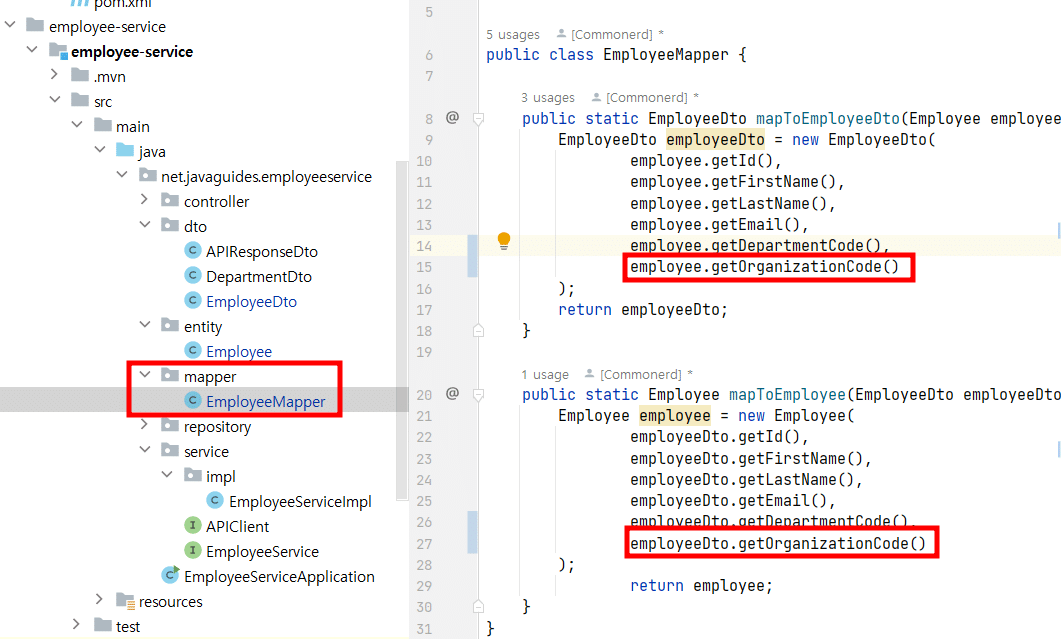
public class EmployeeMapper {
public static EmployeeDto mapToEmployeeDto(Employee employee) {
EmployeeDto employeeDto = new EmployeeDto(
employee.getId(),
employee.getFirstName(),
employee.getLastName(),
employee.getEmail(),
employee.getDepartmentCode(),
employee.getOrganizationCode()
);
return employeeDto;
}
public static Employee mapToEmployee(EmployeeDto employeeDto) {
Employee employee = new Employee(
employeeDto.getId(),
employeeDto.getFirstName(),
employeeDto.getLastName(),
employeeDto.getEmail(),
employeeDto.getDepartmentCode(),
employeeDto.getOrganizationCode()
);
return employee;
}
}
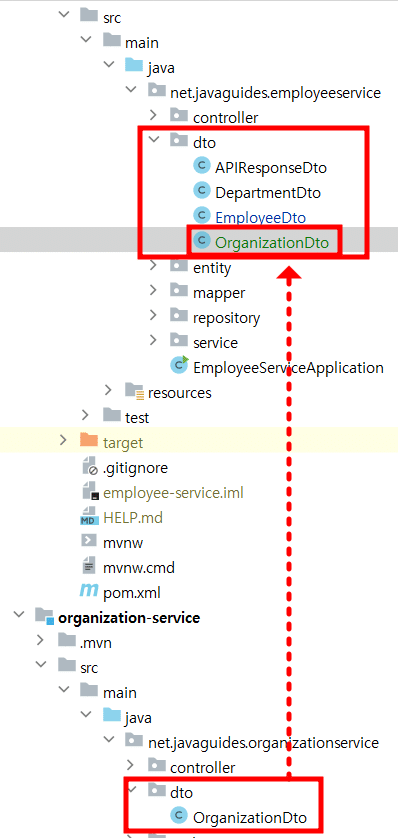
employee-serviceでdtoを作成します。
@Getter
@Setter
@NoArgsConstructor
@AllArgsConstructor
public class OrganizationDto {
private Long id;
private String organizationName;
private String organizationDescription;
private String organizationCode;
private LocalDateTime createdDate;
}
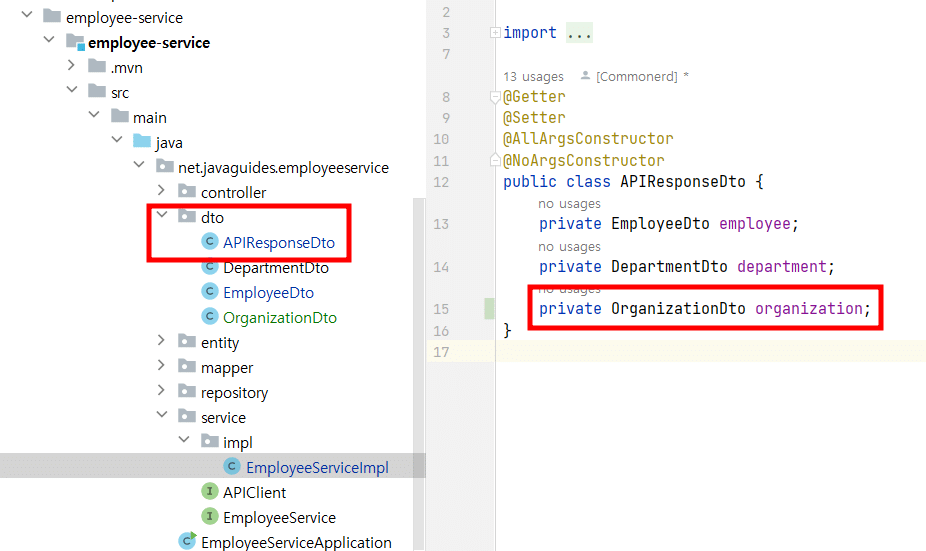
@Getter
@Setter
@AllArgsConstructor
@NoArgsConstructor
public class APIResponseDto {
private EmployeeDto employee;
private DepartmentDto department;
private OrganizationDto organization;
}
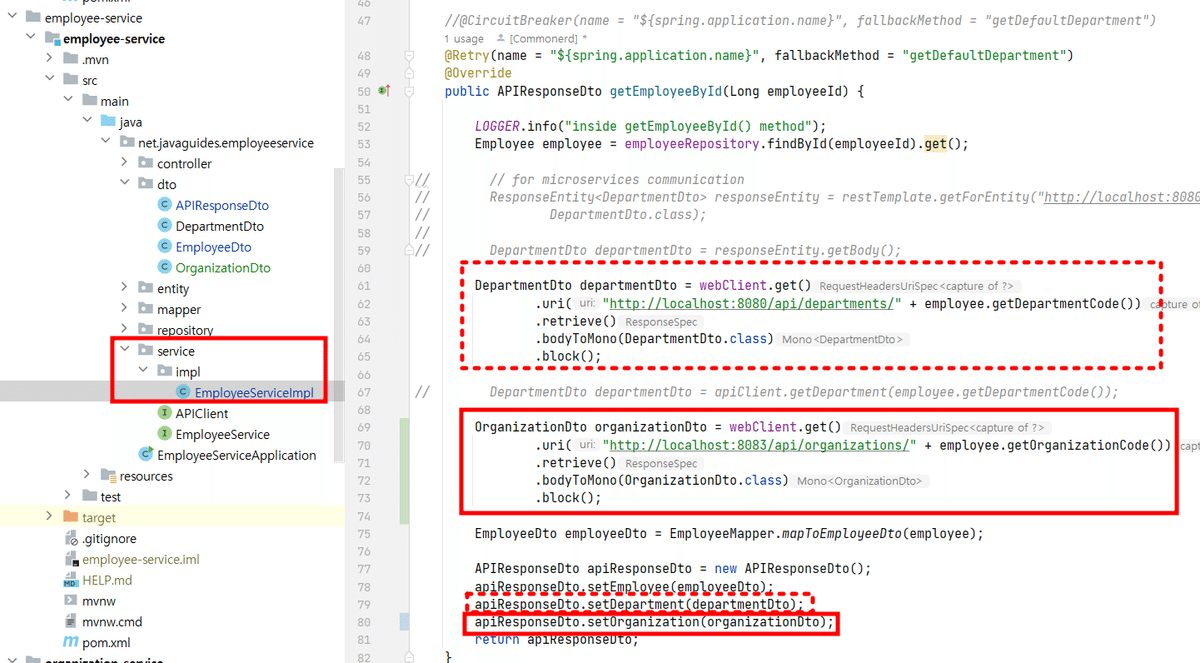
//@CircuitBreaker(name = "${spring.application.name}", fallbackMethod = "getDefaultDepartment")
@Retry(name = "${spring.application.name}", fallbackMethod = "getDefaultDepartment")
@Override
public APIResponseDto getEmployeeById(Long employeeId) {
LOGGER.info("inside getEmployeeById() method");
Employee employee = employeeRepository.findById(employeeId).get();
// // for microservices communication
// ResponseEntity<DepartmentDto> responseEntity = restTemplate.getForEntity("http://localhost:8080/api/departments/" + employee.getDepartmentCode(),
// DepartmentDto.class);
//
// DepartmentDto departmentDto = responseEntity.getBody();
DepartmentDto departmentDto = webClient.get()
.uri("http://localhost:8080/api/departments/" + employee.getDepartmentCode())
.retrieve()
.bodyToMono(DepartmentDto.class)
.block();
// DepartmentDto departmentDto = apiClient.getDepartment(employee.getDepartmentCode());
OrganizationDto organizationDto = webClient.get()
.uri("http://localhost:8083/api/organizations/" + employee.getOrganizationCode())
.retrieve()
.bodyToMono(OrganizationDto.class)
.block();
EmployeeDto employeeDto = EmployeeMapper.mapToEmployeeDto(employee);
APIResponseDto apiResponseDto = new APIResponseDto();
apiResponseDto.setEmployee(employeeDto);
apiResponseDto.setDepartment(departmentDto);
apiResponseDto.setOrganization(organizationDto);
return apiResponseDto;
}
ここまでは一つのサービス(employee-service)からe別のサービス(organization-service)へapiを呼び出す方法を実装したコードでした。
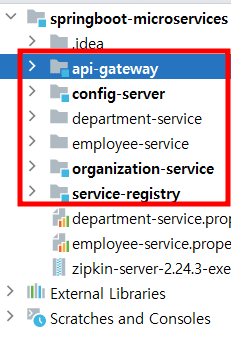
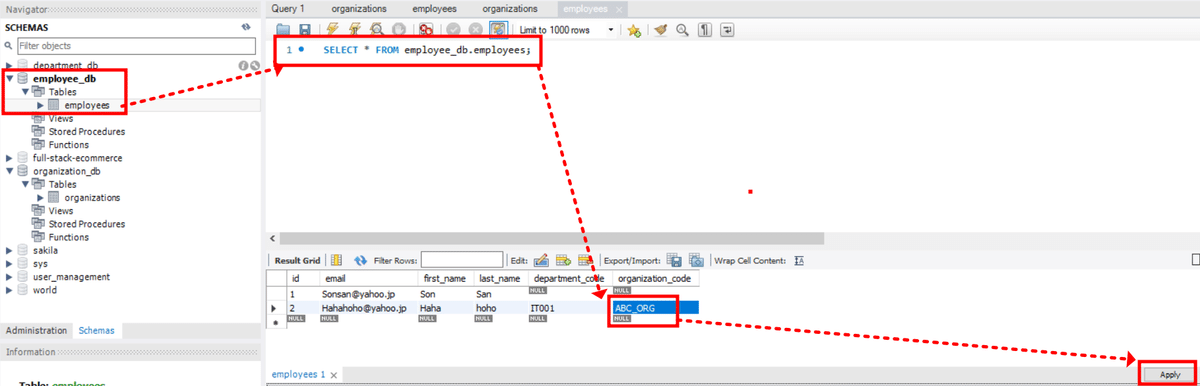
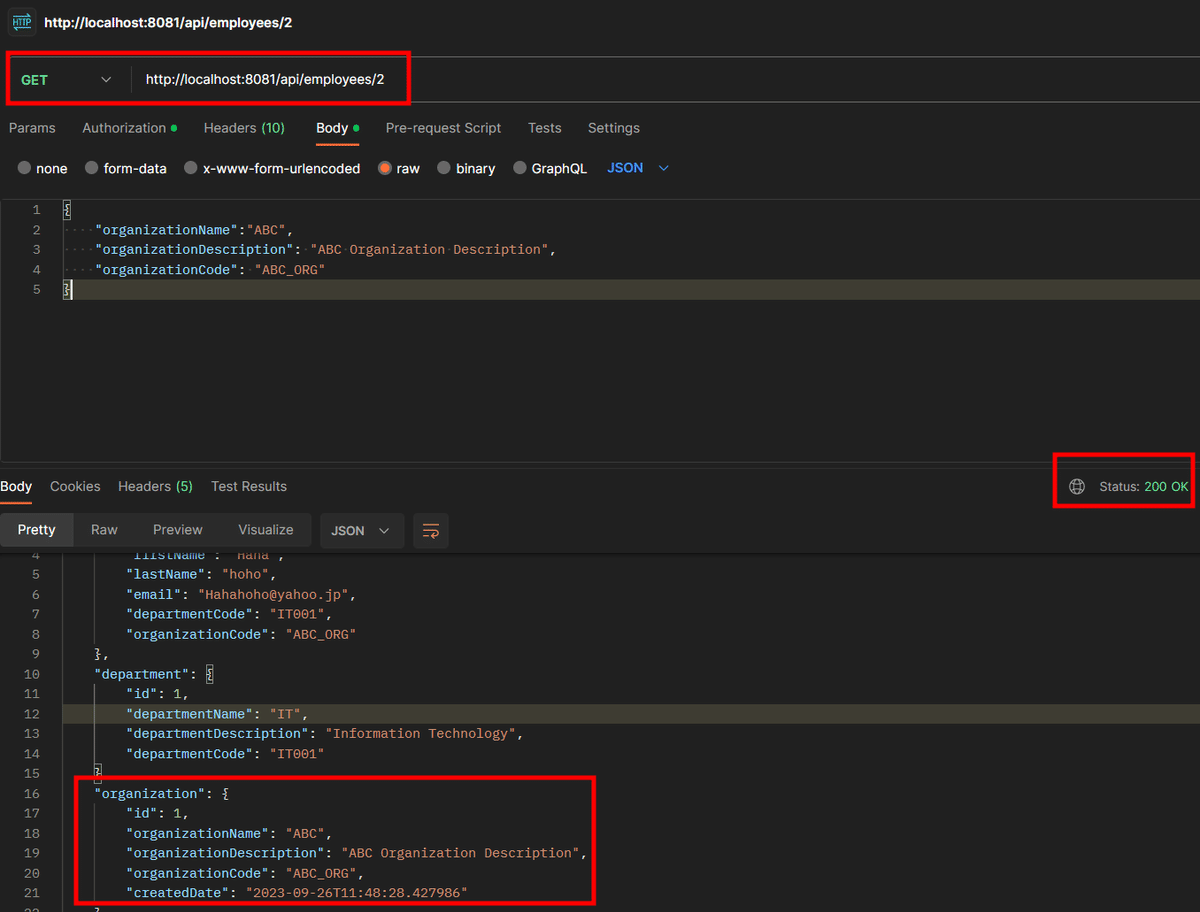

かなり大変ですね!
3. サービスの有機的結合
Eureka Clientに登録
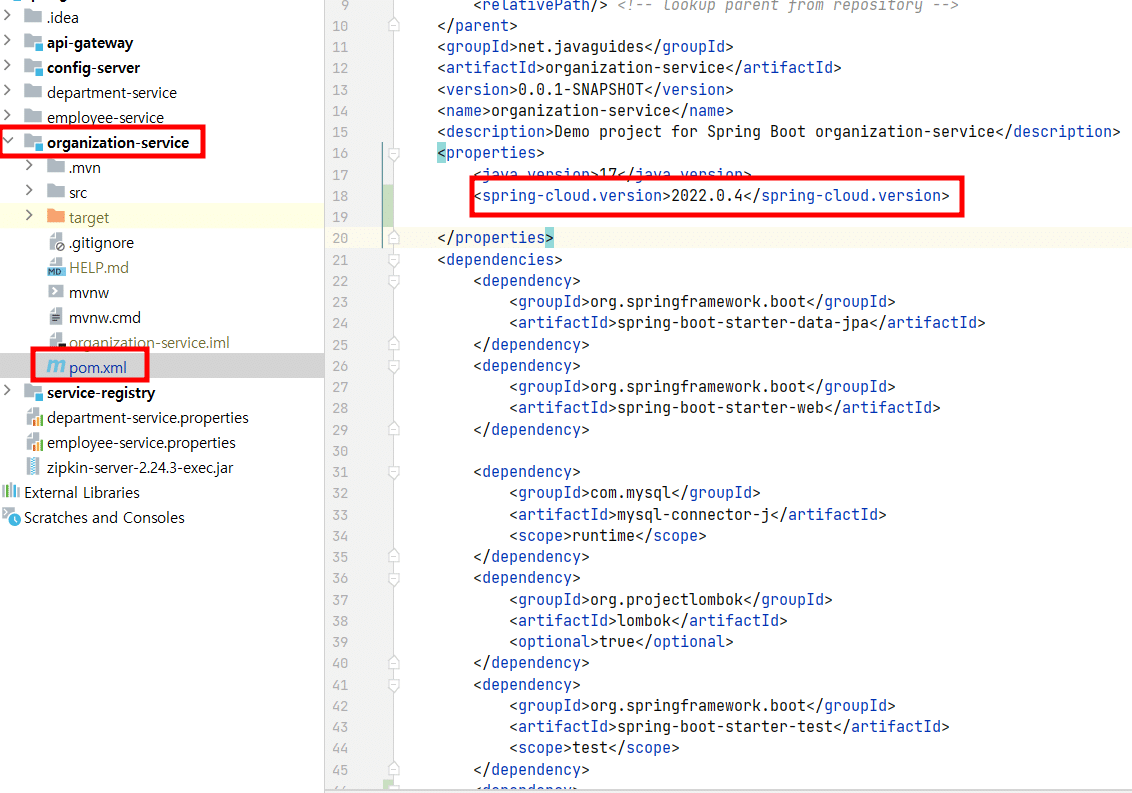
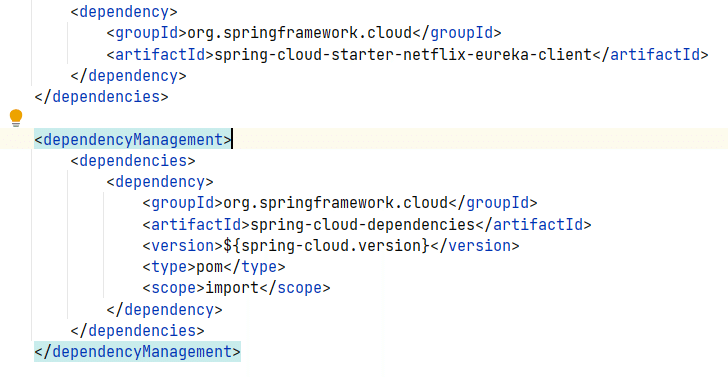
<properties>
<java.version>17</java.version>
<spring-cloud.version>2022.0.4</spring-cloud.version>
</properties>
<dependencies>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
</dependencies>
...
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>${spring-cloud.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<build>
...
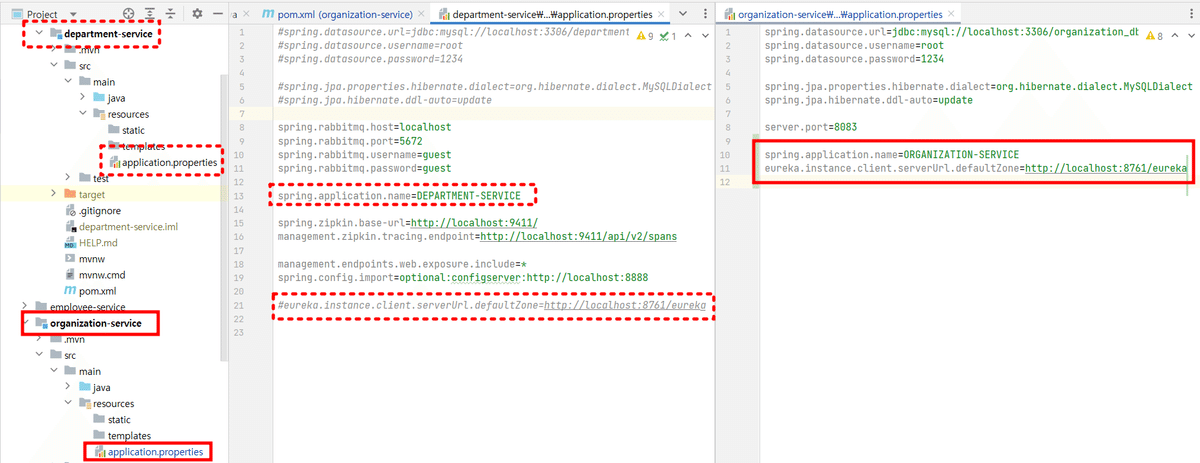
spring.application.name=ORGANIZATION-SERVICE
eureka.instance.client.serverUrl.defaultZone=http://localhost:8761/eureka
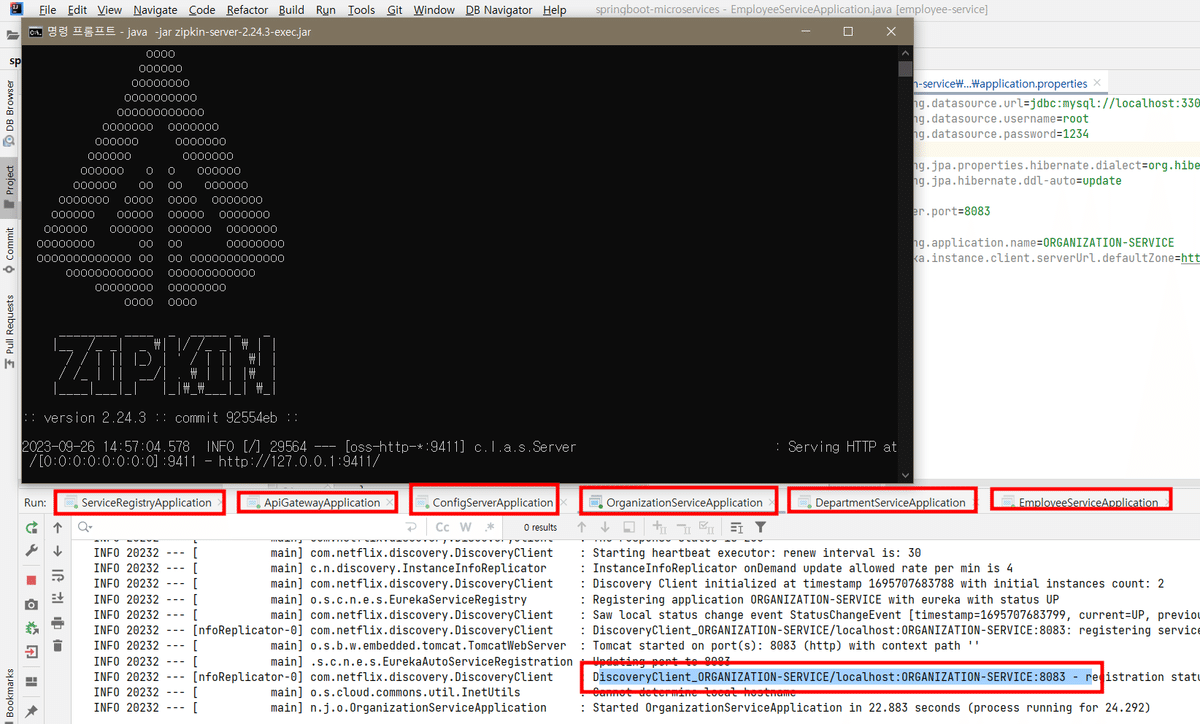
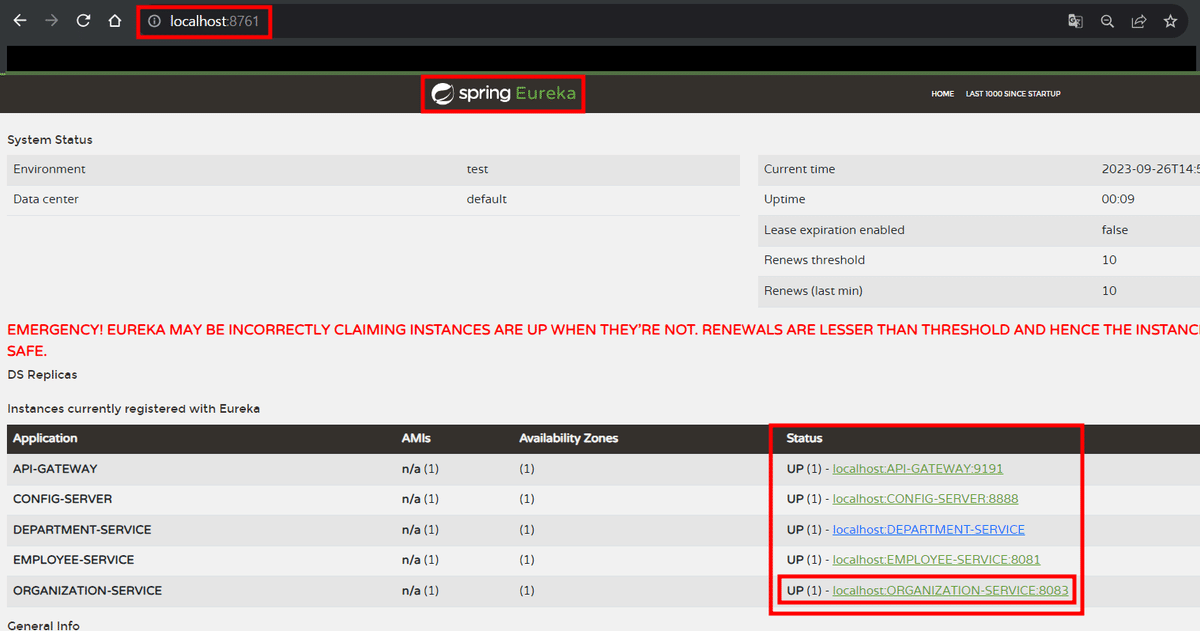
ORGANIZATION-SERVICEがうまく動作しています。
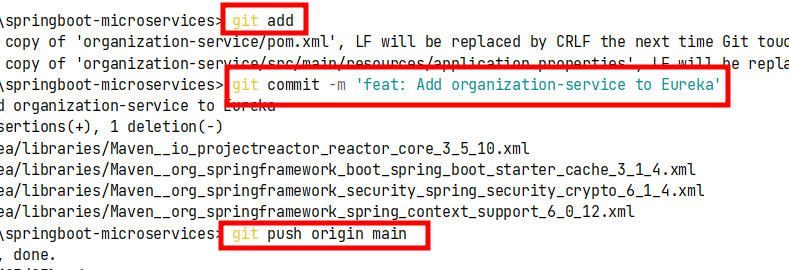
可能であれば一つのコミットには一つの単位を入れるようにしています。
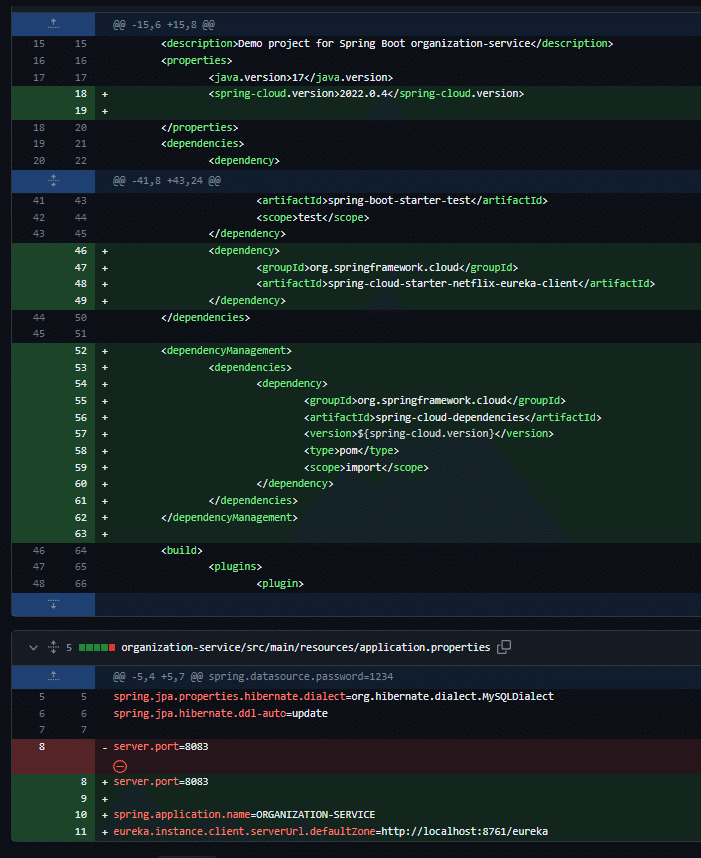
Config Serverに登録
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
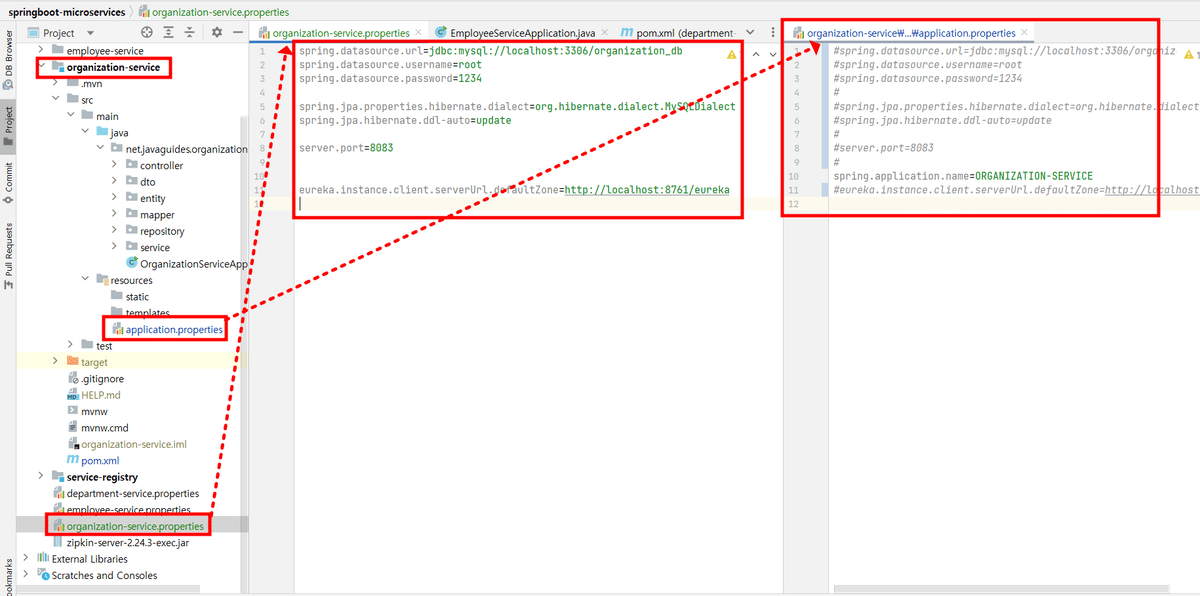
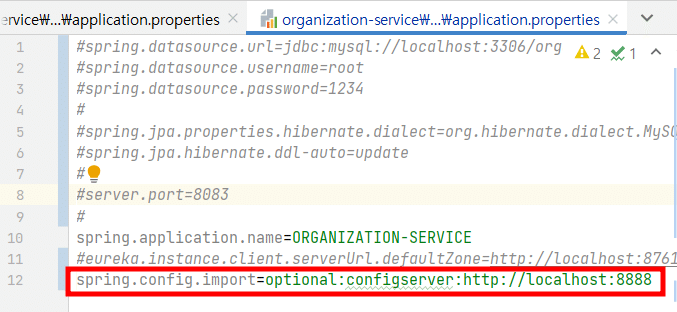
spring.config.import=optional:configserver:http://localhost:8888
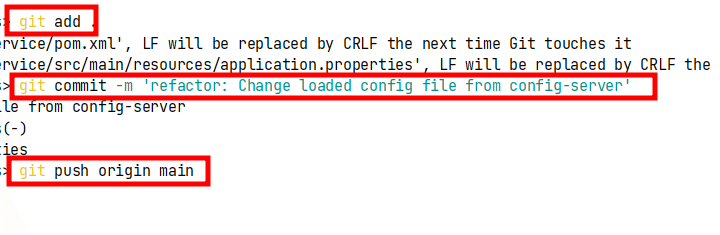
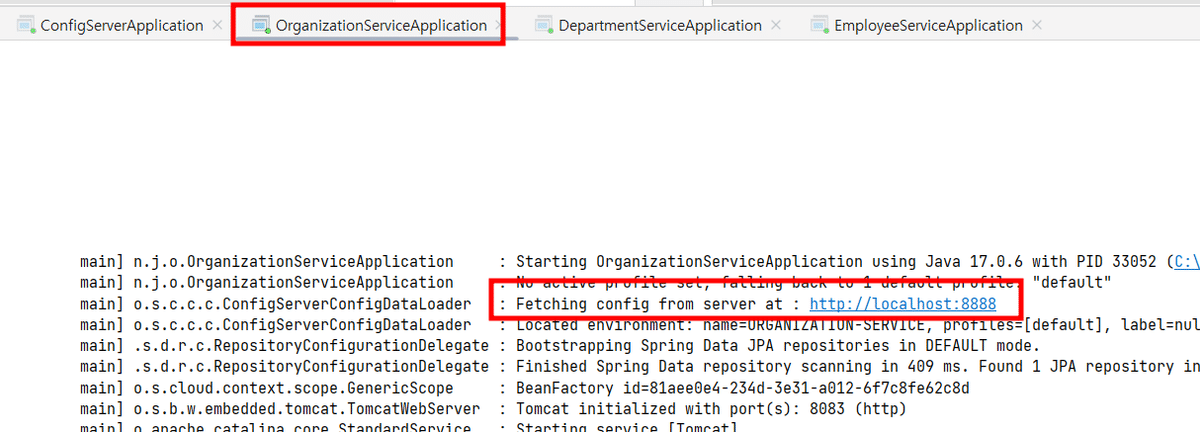
Cloud Bus(RabbitMQ, Actuator)とAPI Gatewayの設定
organization-service/pom.xml
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-bus-amqp</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
organization-serviceのapplicatiop.properties
spring.rabbitmq.host=localhost
spring.rabbitmq.port=5672
spring.rabbitmq.username=guest
spring.rabbitmq.password=guest
management.endpoints.web.exposure.include=*
api-gatewayのapplicatiop.properties
## Routes for Organization Service
spring.cloud.gateway.routes[2].id=ORGANIZATION-SERVICE
spring.cloud.gateway.routes[2].uri=lb://ORGANIZATION-SERVICE
spring.cloud.gateway.routes[2].predicates[0]=Path=/api/organizations/**
api-gateway, organization-service を再起動します。
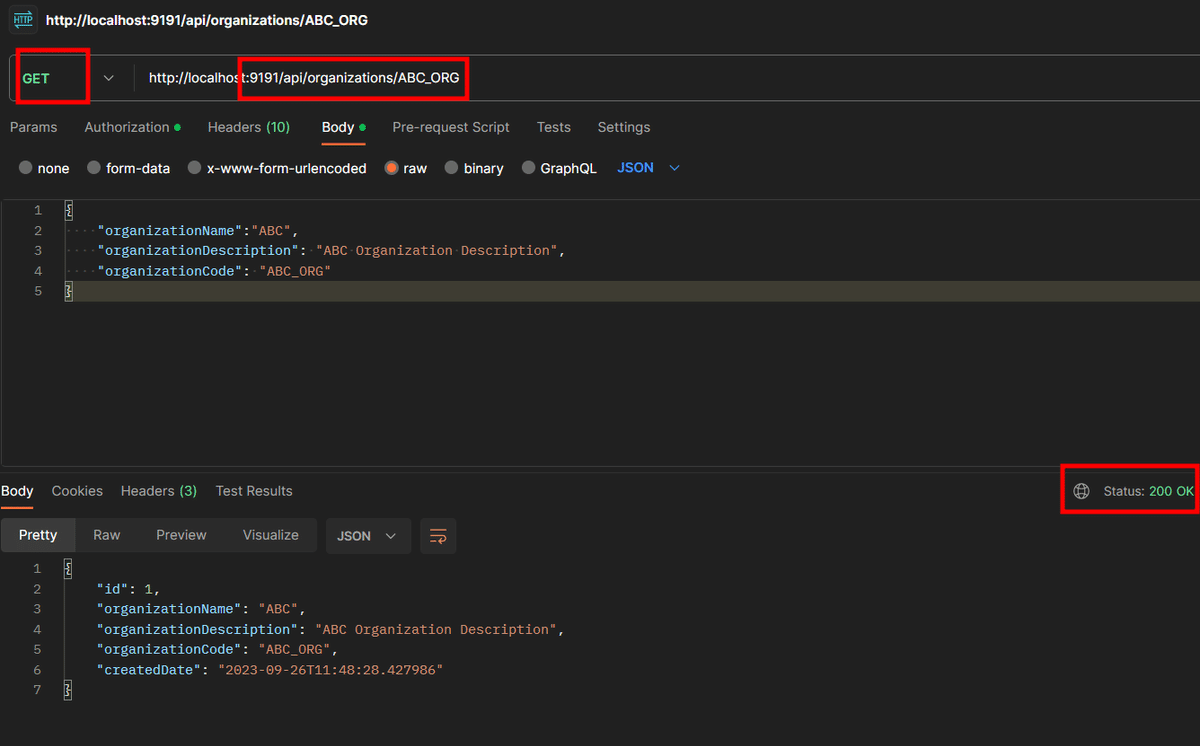
分散トレーシング(Zipkin)の設定
organization-serviceのpom.xml
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-observation</artifactId>
</dependency>
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-tracing-bridge-brave</artifactId>
</dependency>
<dependency>
<groupId>io.zipkin.reporter2</groupId>
<artifactId>zipkin-reporter-brave</artifactId>
</dependency>
<dependency>
<groupId>io.github.openfeign</groupId>
<artifactId>feign-micrometer</artifactId>
</dependency>
orginization-serviceのapplication.properties
spring.zipkin.base-url=http://localhost:9411/
management.zipkin.tracing.endpoint=http://localhost:9411/api/v2/spans
config-serverのorginization-service.properties
management.tracing.sampling.probability=1.0
logging.pattern.level=%5p [${spring.application.name:},%X{traceId:-},%X{spanId:-}]
logging.level.org.springframework.web=DEBUG
management.zipkin.tracing.endpoint=http://localhost:9411/api/v2/spans
management.endpoints.web.exposure.include=*
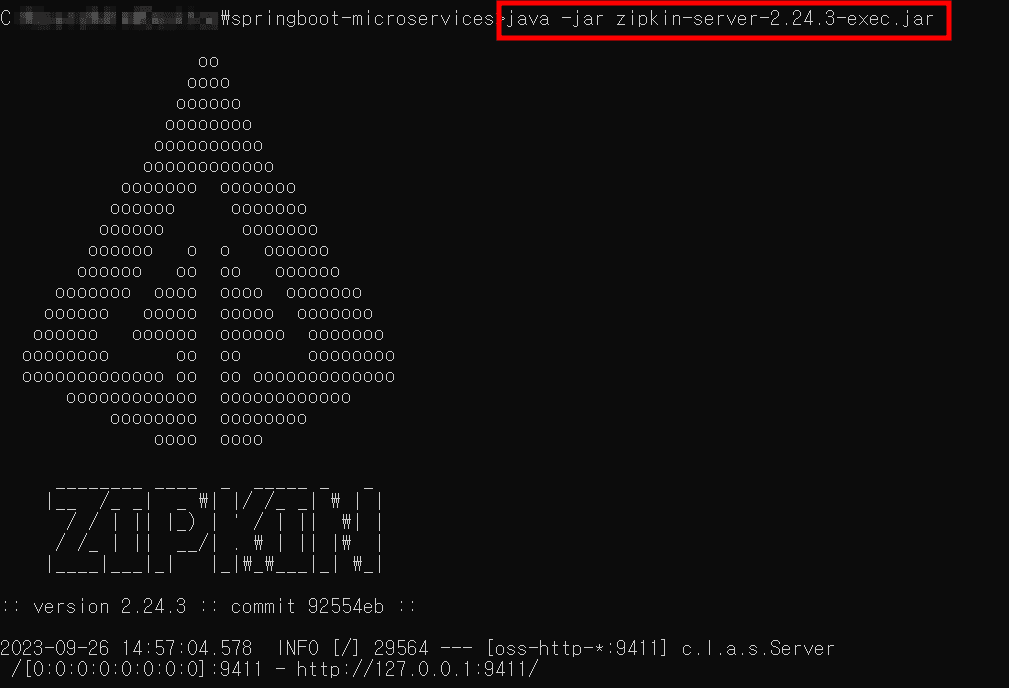
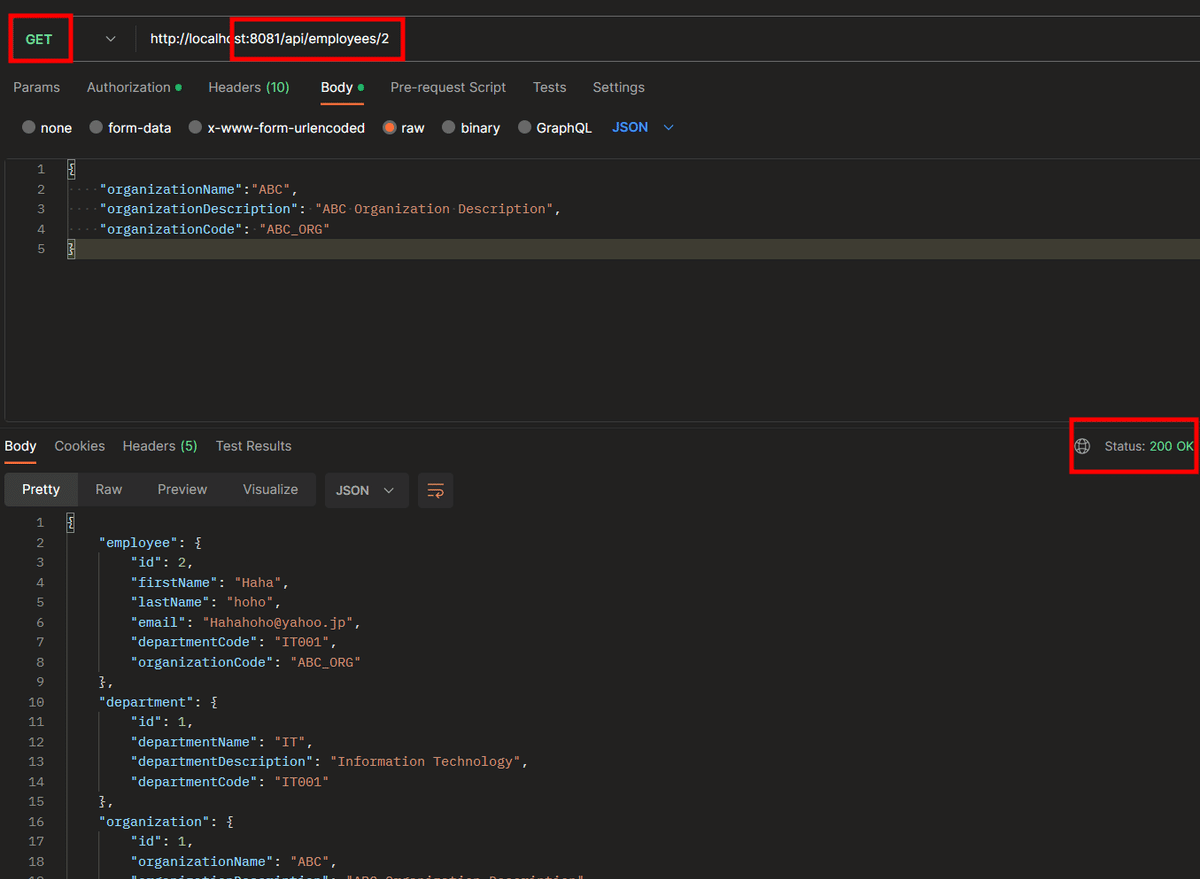

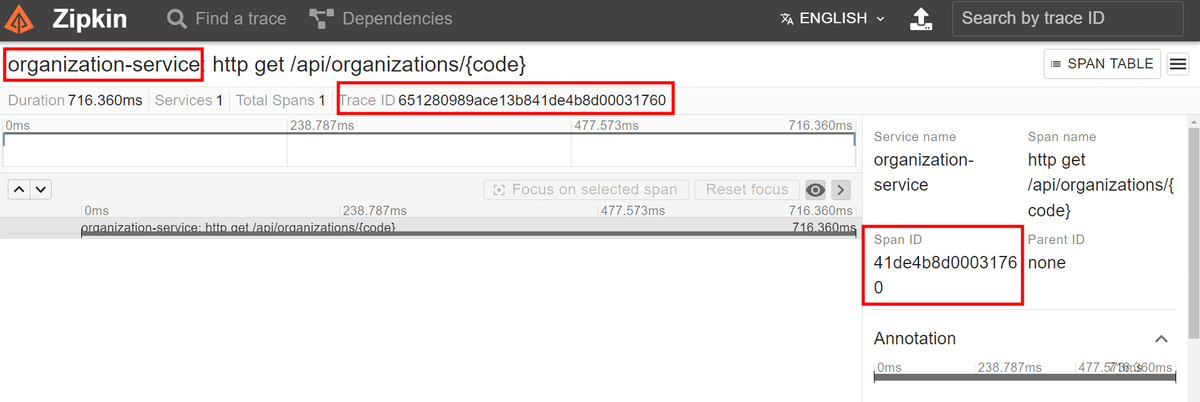
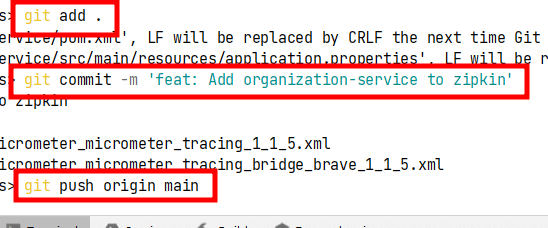
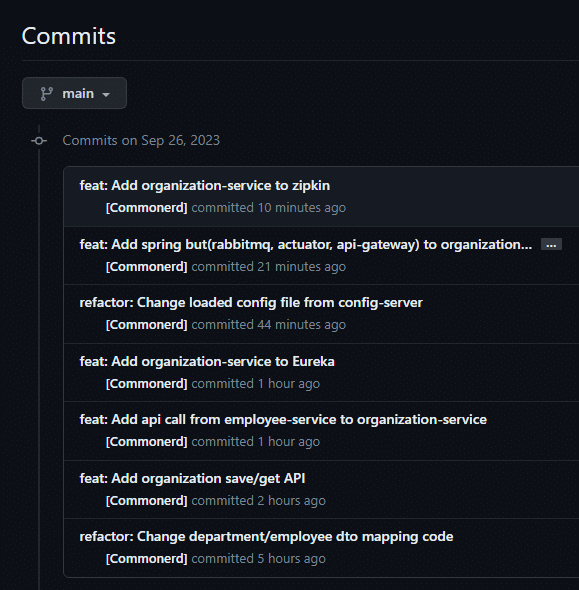
https://github.com/Commonerd/springboot-microservices/commits/main
4. 終わりに
今までdepartment-service, employee-serviceの二つのメインサービスだったマイクロサービスシステムに、organization-serviceを一つ追加してシステムを拡張してみました。
それぞれのサービスが内部的に完結性があって実際に実装してみる味がありますね!本当に面白いです!そして、思ったより一度システムを構築しておけば、Eureka ClientやSpring Cloudなどは似たような原理で適用されるので、再利用性も高く、開発の生産性も良いようですね。 もちろん、これは技術スタックがほぼ同じように適用されたので、そう思うかもしれません。もし、全く違うフレームワークと言語、DBを使うと全く違う状況が展開されるかもしれません。 でも、それでも、
今までバックエンドロジックだけに集中していたら、次回は簡単にフロントエンドの領域をReactフレームワーク(ライブラリで見る人もいますが…)を使って簡単に実装してみようと思います。 かなり有名で人気もありますが、実際に使ったことがないのでワクワクしますね!
エンジニアファーストの会社 株式会社CRE-CO
ソンさん
【参考】
[Udemy] Building Microservices with Spring Boot & Spring Cloud
この記事が気に入ったらサポートをしてみませんか?