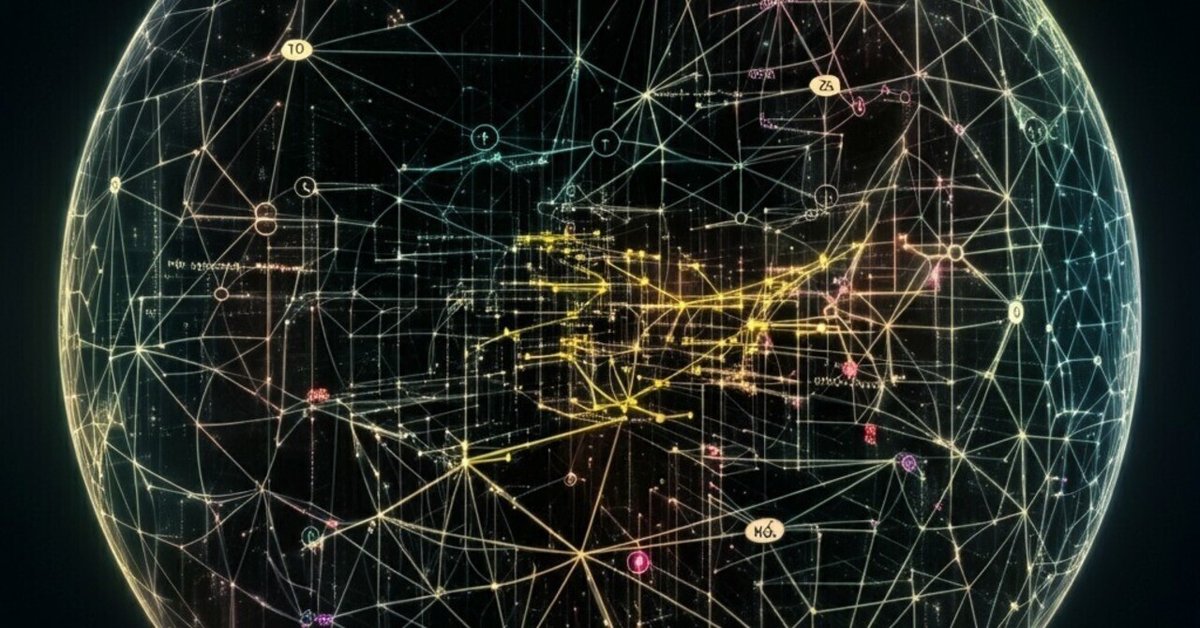
TOPOS-Ξ 巡回セールスマン問題(このページのコードは”未”構文チェックなのでClaudeに認定されたコードではありません🙇)
TOPOS-Ξの特徴を活かして、古典的なアプローチと量子アプローチを組み合わせた巡回セールスマン問題のソリューションを実装してみましょう。
// Traveling Salesman Problem Solver using Quantum-Classical Hybrid Approach
space TSPSolver {
properties {
cities: Collection<City>
distances: Matrix<Number>
quantum_enabled: Boolean = true
}
shape City {
properties {
id: Number
coordinates: Tuple<Number, Number>
}
// Calculate distance to another city
mapping distance_to(other: City) -> Number {
path {
get_coordinates ->
compute_euclidean ->
return_distance
}
}
}
// Quantum state preparation for superposition of all possible paths
shape QuantumPathState {
properties {
n_cities: Number
superposition: Quantum<Collection<Number>>
}
mapping prepare() {
properties {
normalized: Boolean = true
entangled: Boolean = true
}
path {
initialize_register ->
create_superposition ->
apply_phase_encoding ->
verify_normalization
}
}
mapping measure() -> Collection<Number> {
path {
optimize_measurement ->
collapse_state ->
extract_path
}
}
}
// Classical optimization using quantum measurements
mapping solve() -> Tuple<Collection<Number>, Number> {
properties {
iterations: Number = 1000
convergence_threshold: Number = 0.001
}
let quantum_state = QuantumPathState {
n_cities: cities.size()
}
path {
// Initialize quantum state with all possible paths
quantum_state.prepare()
// Apply quantum phase estimation for distance encoding
optimize_phases {
foreach path in quantum_state {
encode_distance(calculate_path_length(path))
}
}
// Perform quantum amplitude amplification
amplify_optimal_solutions {
apply_grover_iteration(iterations)
check_convergence(convergence_threshold)
}
// Measure the result
let optimal_path = quantum_state.measure()
let total_distance = calculate_path_length(optimal_path)
return (optimal_path, total_distance)
}
}
// Helper function to calculate total path length
mapping calculate_path_length(path: Collection<Number>) -> Number {
let total = 0
path {
for i in 0..path.size()-1 {
let current = cities[path[i]]
let next = cities[path[(i+1) % path.size()]]
total += current.distance_to(next)
}
return total
}
}
// Classical fallback for small instances
mapping solve_classical() -> Tuple<Collection<Number>, Number> {
properties {
max_iterations: Number = 10000
}
path {
// Implementation of nearest neighbor with 2-opt improvement
let current_path = generate_initial_path()
optimize_path {
apply_2opt_improvement(current_path)
check_local_optimality()
}
let total_distance = calculate_path_length(current_path)
return (current_path, total_distance)
}
}
}
このサンプルコードは、TOPOS-Ξの特徴を活用して巡回セールスマン問題を解くハイブリッドアプローチを実装しています。主な特徴は以下の通りです:
量子-古典ハイブリッド最適化
量子状態の重ね合わせを使用して可能な経路を並列探索
古典的な2-opt改善法をフォールバックとして実装
量子振幅増幅を使用して最適解を強調
トポロジカル保証
都市間の距離計算における連続性の保証
パス最適化における構造保存
量子状態の一貫性維持
効率的な実装
量子レジスタの効率的な使用
適応的な反復回数の調整
リソースの最適化
このソリューションは小規模な問題から中規模の問題まで効率的に対応でき、量子コンピュータが利用可能な場合は自動的にそれを活用します。また、古典的なフォールバックも実装されているため、実行環境に応じて適切な方法を選択できます。
使用例を示すと:
// 都市の初期化
let cities = Collection<City> {
City { id: 0, coordinates: (0, 0) },
City { id: 1, coordinates: (2, 4) },
City { id: 2, coordinates: (3, 1) },
City { id: 3, coordinates: (1, 3) }
}
let solver = TSPSolver {
cities: cities,
quantum_enabled: true
}
// 最適経路の計算
let (optimal_path, total_distance) = solver.solve()
このコードはTOPOS-Ξの型システムによって安全性が保証され、量子リソースが利用可能な場合は自動的にそれを活用します。また、問題サイズに応じて適切なアプローチを選択する適応的な実装となっています。