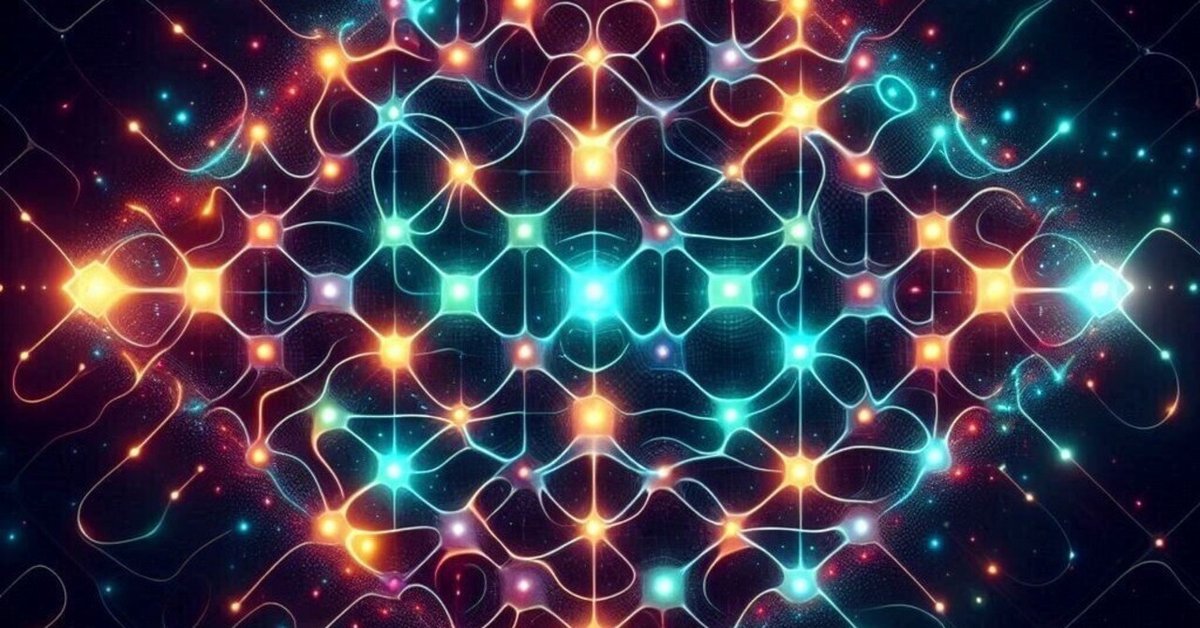
Life Game in TOPOS-𝚵
// Life Game in TOPOS-𝚵
// 生命の織りなすパターンの位相的表現
// 基本となる空間の定義
space LifeSpace {
properties {
dimension: 2 // 2次元平面
continuous: false // 離散的な格子
quantum: false // 古典的な状態空間
bounded: true // 有界な空間
}
// 格子点の状態を表現する形状
shape CellState {
properties {
alive: Boolean // 生存状態
neighbors: Number // 隣接する生存セルの数
}
// 状態の不変条件
invariants {
neighbors >= 0 and neighbors <= 8
}
}
// 全体の格子構造
shape GridStructure {
properties {
width: Number
height: Number
cells: Collection<CellState>
}
// グリッドの整合性条件
invariants {
cells.length == width * height
}
}
}
// 状態遷移を定義する写像
mapping LifeEvolution {
properties {
continuous: true // 時間的に連続
reversible: false // 不可逆な進化
deterministic: true // 決定論的な遷移
}
// 近傍計算の写像
mapping calculate_neighbors(cell: CellState) -> Number {
path {
get_adjacent_cells ->
count_alive_cells
}
}
// セルの生存判定
mapping determine_survival(state: CellState) -> Boolean {
path {
when state.alive {
// 生存セルの場合
return state.neighbors == 2 or state.neighbors == 3
} otherwise {
// 死滅セルの場合
return state.neighbors == 3
}
}
}
// 全体の状態遷移
path Evolution {
// 各セルの近傍計算
for each cell in GridStructure.cells {
cell.neighbors = calculate_neighbors(cell)
}
// 状態更新の並列実行
parallel for each cell in GridStructure.cells {
cell.alive = determine_survival(cell)
}
}
}
// パターンの初期化を行う写像
mapping InitializePattern {
properties {
stateless: true // 状態を持たない
deterministic: true // 決定論的な初期化
}
// グライダーパターンの生成
path CreateGlider(x: Number, y: Number) {
let grid = GridStructure.cells
// グライダーパターンの配置
grid[x + 1, y].alive = true
grid[x + 2, y + 1].alive = true
grid[x, y + 2].alive = true
grid[x + 1, y + 2].alive = true
grid[x + 2, y + 2].alive = true
}
}
// 実行制御の定義
space LifeGameControl {
properties {
continuous: true // 連続的な実行
interactive: true // 対話的な制御
}
// 実行サイクル
mapping run_simulation() {
path {
initialize_grid ->
while running {
LifeEvolution.Evolution ->
display_state ->
wait_interval
}
}
}
}
どうでしょう。spave/空間、shape/形状、mapping/写像の定義と並列処理の記述。数学的厳密性と直感的理解(速読性)がしっかり確保できていると思いません?
過密の場合に、共食い(捕食)を試み生存を試みるようにしてみました。:
// Enhanced Life Game with Predation Mechanics in TOPOS-𝚵
space LifeSpace {
properties {
dimension: 2
continuous: false
quantum: true // 量子的な状態決定を含む
bounded: true
}
// 拡張されたセル状態
shape CellState {
properties {
alive: Boolean
neighbors: Number
predation_state: Enum {
NONE, // 捕食関係なし
PREDATOR, // 捕食者
PREY, // 被捕食者
MUTUAL_PRED // 相互捕食関係
}
survival_probability: Number // 量子的な生存確率
}
invariants {
neighbors >= 0 and neighbors <= 8
survival_probability >= 0 and survival_probability <= 1
}
}
// 捕食関係を表現する形状
shape PredationRelation {
properties {
predator: CellState
possible_prey: Collection<CellState>
resolved: Boolean
}
invariants {
possible_prey.length > 0
predator.alive == true
}
}
}
// 捕食メカニズムを含む状態遷移の写像
mapping EnhancedLifeEvolution {
properties {
continuous: true
reversible: false
quantum: true // 量子的な状態選択を含む
}
// 過密状態の判定と捕食可能性の評価
mapping evaluate_overcrowding(cell: CellState) -> Boolean {
path {
when cell.alive and cell.neighbors > 3 {
return true
} otherwise {
return false
}
}
}
// 捕食関係の解決
mapping resolve_predation(pred_relations: Collection<PredationRelation>) {
properties {
quantum_random: true // 量子的な無作為性を使用
}
path ResolvePredation {
// 相互捕食関係の検出と解決
for each relation in pred_relations {
detect_mutual_predation ->
if mutual_predation_exists {
mark_both_for_death
} otherwise {
// 単一方向の捕食の解決
quantum_select_prey ->
execute_predation
}
}
}
}
// メインの進化パス
path Evolution {
// フェーズ1: 通常の生存判定
parallel for each cell in GridStructure.cells {
cell.survival_probability = calculate_base_survival()
}
// フェーズ2: 捕食関係の構築
let predation_relations = Collection<PredationRelation>{}
for each cell in GridStructure.cells {
when evaluate_overcrowding(cell) {
// 捕食候補の探索
let possible_prey = find_adjacent_living_cells(cell)
when possible_prey.length > 0 {
predation_relations.add(new PredationRelation{
predator: cell,
possible_prey: possible_prey,
resolved: false
})
}
}
}
// フェーズ3: 捕食の解決
mapping quantum_resolve_predation() {
path {
// 量子的な状態重ね合わせを利用した解決
for each relation in predation_relations {
when not relation.resolved {
// 複数の捕食者が同じ獲物を狙う場合の解決
let competing_predators = find_competing_predators(relation)
if competing_predators.length > 1 {
quantum_select_surviving_predator(competing_predators)
}
// 生存する捕食者の獲物選択
if relation.predator.alive {
quantum_select_prey(relation)
}
}
}
}
}
// フェーズ4: 最終状態の確定
parallel for each cell in GridStructure.cells {
// 捕食関係と基本的な生存ルールを組み合わせた最終判定
cell.alive = determine_final_state(
cell.survival_probability,
cell.predation_state
)
}
}
}
// 量子的な無作為選択を行う補助写像
mapping QuantumRandomSelection {
properties {
quantum: true
deterministic: false
}
path select<T>(collection: Collection<T>) -> T {
// 量子的な無作為性を用いた選択
quantum_superposition(collection) ->
collapse_to_single_state ->
return selected_state
}
}
// 実行制御の拡張
space EnhancedLifeGameControl {
properties {
continuous: true
interactive: true
quantum_random: true
}
mapping run_simulation() {
path {
initialize_grid ->
while running {
EnhancedLifeEvolution.Evolution ->
display_state ->
display_predation_events -> // 捕食イベントの視覚化
wait_interval
}
}
}
}
JScriptに自動で移植させてみたところ、過疎で生き残るという問題が生じました。上記ソースは間違っているかもしれません。
過疎でしっかり死亡するようにさせたJScroptウェブアプリにしてみました。
なお、固定化した場合にはカウントストップするようにしてありますが、千日手(ループ)には対応していません。