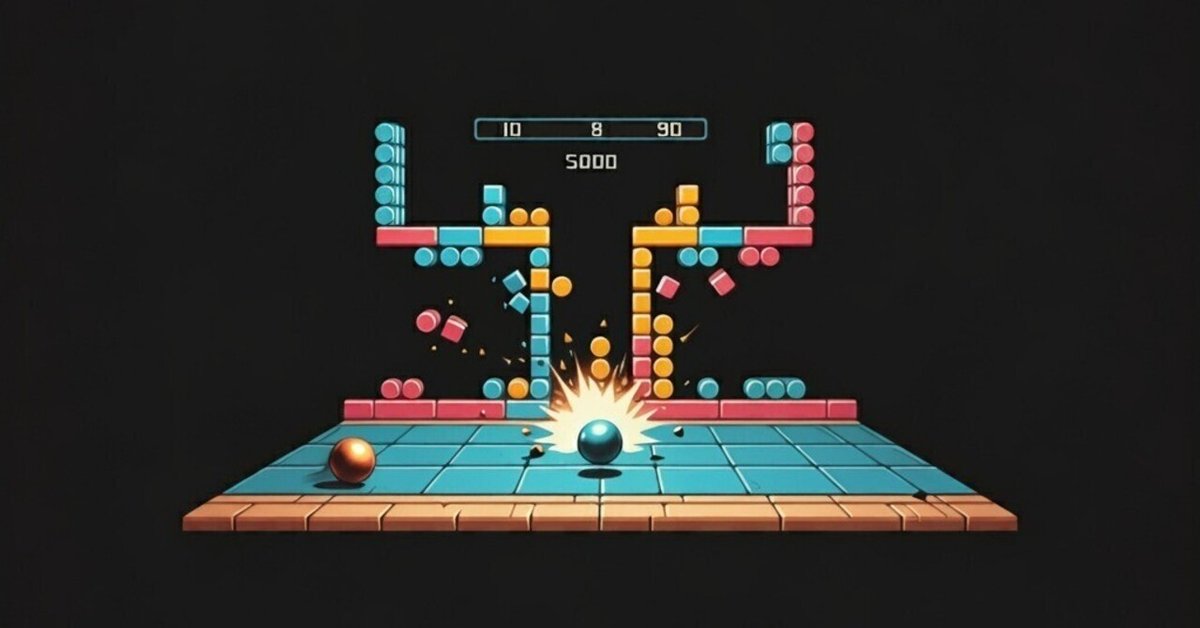
Break out in TOPOS-Ξ
space BreakoutGame {
properties {
dimension: Topology<Number>(2)
continuous: Topology<Boolean>(false)
temporal: Topology<Boolean>(true)
}
// ゲーム時間の構造
shape TimeFlow {
properties {
delta: Topology<Number>
continuous: Topology<Boolean>(false)
frame_rate: Topology<Number>(60)
}
// 時間発展
mapping evolve() {
properties {
continuous: false
deterministic: true
periodic: true
}
path {
update_game_state ->
process_collisions ->
render_frame
}
}
}
// ボールの定義
shape Ball {
properties {
position: Topology<Vector2D>
velocity: Topology<Vector2D>
radius: Topology<Number>
active: Topology<Boolean>
}
// ボールの移動
mapping move() {
properties {
continuous: true
temporal: true
}
path {
update_position ->
check_boundaries ->
handle_collisions
}
}
// 衝突判定と反射
mapping collide() {
properties {
reversible: true
elastic: true
}
path {
detect_collision ->
calculate_reflection ->
update_velocity
}
}
}
// パドルの定義
shape Paddle {
properties {
position: Topology<Vector2D>
width: Topology<Number>
speed: Topology<Number>
}
// パドルの移動
mapping move() {
properties {
continuous: false
input_driven: true
}
path {
read_input ->
validate_position ->
update_position
}
}
}
// ブロックの定義
shape Block {
properties {
position: Topology<Vector2D>
size: Topology<Vector2D>
active: Topology<Boolean>
points: Topology<Number>
}
// ブロックの状態更新
mapping update_state() {
properties {
discrete: true
reversible: false
}
path {
check_collision ->
update_active_state ->
calculate_score
}
}
}
// 入力制御システム
shape InputController {
properties {
key_states: Topology<Map<String, Boolean>>
response_time: Topology<Number>
}
// キー入力の処理
mapping process_input() {
properties {
discrete: true
event_driven: true
}
path {
read_key_states ->
map_to_actions ->
update_game_state
}
}
}
// ゲーム状態管理
shape GameState {
properties {
score: Topology<Number>
lives: Topology<Number>
level: Topology<Number>
active: Topology<Boolean>
}
// ゲーム状態の更新
mapping update() {
properties {
temporal: true
discrete: true
}
path {
check_win_condition ->
check_loss_condition ->
update_game_status
}
}
}
// メインゲームループ
mapping game_loop() {
properties {
continuous: true
temporal: true
input_responsive: true
}
path {
while(game_active) {
process_input ->
update_ball ->
update_paddle ->
check_collisions ->
update_blocks ->
update_score ->
render_frame
}
}
}
// 衝突検出システム
shape CollisionSystem {
properties {
precision: Topology<Number>
response_type: Topology<String>
}
mapping detect_and_resolve() {
properties {
continuous: false
deterministic: true
}
path {
check_ball_paddle ->
check_ball_blocks ->
check_ball_walls ->
resolve_collisions
}
}
}
}
// 実装詳細
space GameImplementation {
// 物理演算
mapping physics_update() {
properties {
temporal: true
deterministic: true
}
path {
update_positions ->
handle_collisions ->
apply_constraints
}
}
// レベル生成
mapping generate_level() {
properties {
discrete: true
deterministic: true
}
path {
create_block_layout ->
initialize_positions ->
set_block_properties
}
}
// スコアリングシステム
mapping score_system() {
properties {
accumulative: true
non_negative: true
}
path {
calculate_points ->
update_score ->
check_high_score
}
}
}
プレイできるレベルになってないですけどwww