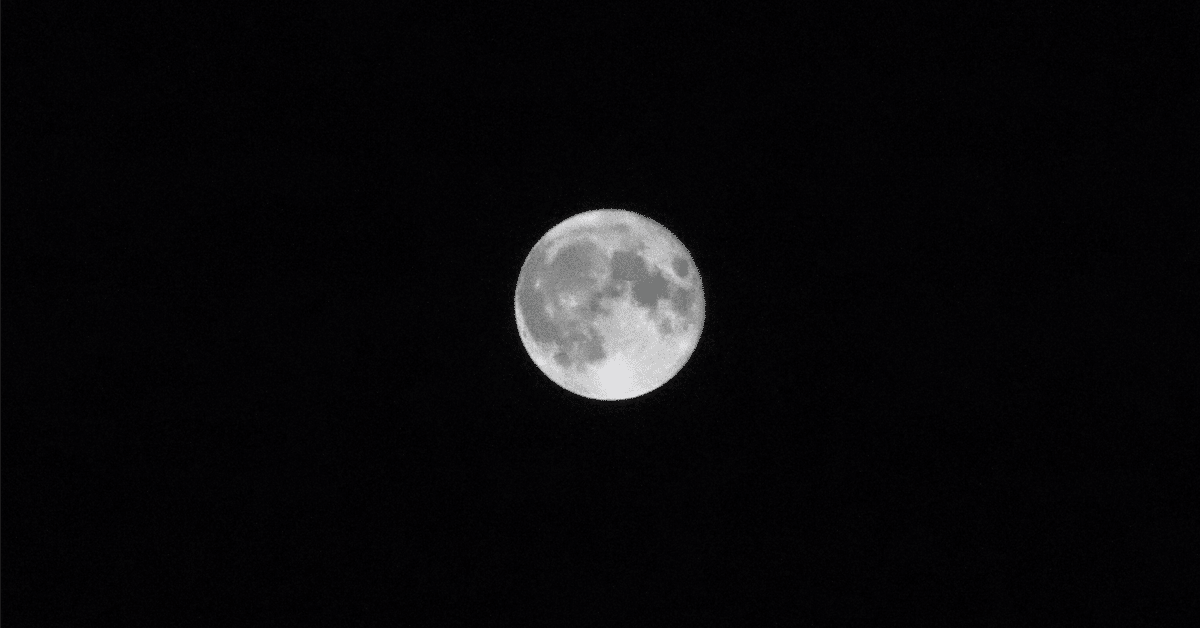
死について教えて
死について教えて
「死」とは生物学的、哲学的、宗教的、文化的な観点から多様に解釈される現象です。以下に、いくつかの側面から死についての概念を説明します。
生物学的な死:
生物学的には、生命活動の終息を指します。人や動物の場合、心停止や脳死がこれに該当するでしょう。しかし、死に至るプロセスやその定義については、医学的・倫理的な議論の対象となっています。
哲学的な死:
死は哲学的にも多くの思索の対象となっています。死後の存在や意味、人はなぜ死を恐れるのか、死とは何か、といった問いが、歴史を通じて多くの哲学者によって議論されてきました。
宗教的な死:
さまざまな宗教には死後の生を説くものが多く、それぞれの教えに基づく死の理解や死後の世界の概念が存在します。例えば、キリスト教では天国と地獄、仏教では輪廻転生などの概念があります。
文化的な死:
文化や社会によって、死や葬儀に関する慣習や考え方は大きく異なります。一部の文化では、死を祝うような明るい葬儀が行われることもあります。一方で、他の文化では喪失感を中心に悲しみを共有することが重視されます。
死は避けられないものとして、多くの人々がそれを受け入れる一方で、死の不確実性や未知の部分への恐れ、そして生きることの価値や意味を再考する契機ともなります。
生きるとは
「生きる」という行為や状態は、生物学的、哲学的、心理的、社会的な観点から様々に解釈されるものです。以下、いくつかの側面から「生きる」とは何かを考察します。
生物学的な観点:
生物学的には、「生きる」とは、細胞が活動し、生命の維持・再生が行われる状態を指します。これは呼吸、摂取、排泄、繁殖などの生命活動を伴います。
哲学的な観点:
「生きる」とは、存在すること、意識を持つこと、または意味を求めることとも言えます。古代ギリシャの哲学者たちは「よく生きる」とは何か、人生の目的や意義について深く考察してきました。さらに、人はどのようにして善き人生を生きるべきかについても議論してきました。
心理的な観点:
ここでは、「生きる」は自己実現、幸福の追求、挑戦や困難を乗り越えること、人間関係を築くことなど、多様な側面を持つものとして捉えられます。
社会的な観点:
社会的には、「生きる」は、共同体の中での役割を果たし、文化や価値観に従って行動することを含む場合が多いです。
人は一人一人が異なる価値観や信念を持っているため、何をもって「生きる」とするかは個人の解釈や経験に左右されます。ある人にとっては家族との関わりが「生きる」の中心であり、別の人にとっては情熱を追い求めること、あるいは自己を超える何かを成し遂げることが「生きる」という行為の核心かもしれません。
このように、多様な観点から「生きる」の意味を考えることができ、それぞれの解釈が共存しているのが現実の世界です。
数式や論理記号で定義して
「生きる」という概念を数式や論理記号で表現するのは非常に難しいです。なぜなら、それは主観的な価値や感情、個人の経験に深く関連しているからです。しかし、論理や数式を用いて「生きる」という概念を抽象的にモデル化する試みをしてみましょう。
生命の存在を \( L \)、生物学的な活動を \( B \)、心理的な活動を \( P \)、社会的な活動を \( S \) と定義します。
「生きる」という状態を \( L \) として表現すると:
\[ L = B \land P \land S \]
ここで、
- \( B \) は生物学的な生命の機能(呼吸、摂取、排泄など)が活発であることを示す。
- \( P \) は感情、意識、思考などの心理的な活動が存在することを示す。
- \( S \) は社会的な役割や関係性の中での活動が存在することを示す。
この数式は極めて単純化されたモデルであり、現実の「生きる」という複雑な概念を完全に表現することはできません。しかし、論理や数式を使って考えることで、複雑な概念を分解し、理解を深める一助となるかもしれません。
Pythonで定義してみて:人間オブジェクトを定義して
Pythonで「人間」を表現するためのシンプルなクラスを作成してみましょう。以下の例では、人間の基本的な特徴や機能を持つHumanクラスを定義しています。
class Human:
def __init__(self, name, age, occupation):
self.name = name # 人の名前
self.age = age # 人の年齢
self.occupation = occupation # 職業や役職
self.alive = True # 生きているかどうかのステータス
def breathe(self):
if self.alive:
return f"{self.name} is breathing."
return f"{self.name} is not breathing."
def eat(self, food):
if self.alive:
return f"{self.name} is eating {food}."
return f"{self.name} cannot eat."
def work(self):
if self.alive:
return f"{self.name} is working as a {self.occupation}."
return f"{self.name} is not working."
def age_up(self):
if self.alive:
self.age += 1
return f"{self.name} is now {self.age} years old."
return f"{self.name} is not aging."
def die(self):
self.alive = False
return f"{self.name} has passed away."
# インスタンスの作成とメソッドのテスト
john = Human("John", 30, "engineer")
print(john.breathe())
print(john.eat("apple"))
print(john.work())
print(john.age_up())
print(john.die())
print(john.breathe())
このクラスは非常に単純化されたもので、実際の「人間」の複雑さや多様性を反映していませんが、オブジェクト指向プログラミングの基本的な考え方を示す一例として参考にしてください。
人間の複雑性とは?
「人間の複雑性」とは、人間の行動、思考、感情、意識、体質、文化、歴史など、多方面にわたる独特の特性や相互関係のことを指します。人間の複雑性は以下の要因に起因すると考えられます:
生物学的な要因:
遺伝: それぞれの人は、遺伝的な情報を元に独特の特性や性質を持っています。
脳の構造と機能: 脳は非常に複雑な構造を持ち、それが思考や感情、意識の形成に関わっています。
生体リズムやホルモンの変動: これらは感情や行動に影響を与えます。
心理的な要因:
思考: 人は複雑な思考パターンや信念を持ち、それが行動や感情に影響を与えます。
感情: 感情は多彩で、時と場面によって異なります。
経験や記憶: 過去の経験や記憶は現在の行動や感情に影響を与えます。
社会的・文化的な要因:
社会的な環境: 家族、友人、職場、コミュニティなど、様々な社会的環境が人の行動や感情に影響を与えます。
文化や教育: 個人が成長する文化や社会は価値観や行動パターンに大きな影響を与えます。
歴史的背景: 個人や社会が直面した歴史的な出来事や経験も、考え方や行動に影響を与えます。
哲学的・霊的な要因:
人は生きる意味、宇宙の存在、道徳や倫理についての独自の解釈や信念を持ちます。
これらの要因は相互に関連し合っており、それが人間の複雑さを形成しています。それぞれの人間はこれらの要因の組み合わせによって独自の存在として形成され、それが多様性や個性として表れます。
オブジェクトを更新して,複雑さを反映して: 必要なら,人間を取り巻く環境もオブジェクトとして定義して
人間の複雑さを反映するための拡張したオブジェクトを考えてみましょう。人間の属性や行動をより詳細にモデル化するとともに、人間を取り巻く環境をEnvironmentクラスとして定義します。
class Environment:
def __init__(self, cultural_context, social_context, historical_events):
self.cultural_context = cultural_context # 文化的背景
self.social_context = social_context # 社会的背景
self.historical_events = historical_events # 歴史的出来事
def influence_person(self, person):
person.beliefs += self.cultural_context
person.social_interactions += self.social_context
person.memories += self.historical_events
class Human:
def __init__(self, name, age, genetics, memories=[], beliefs=[], social_interactions=[]):
self.name = name
self.age = age
self.genetics = genetics # 遺伝的特性
self.brain = {"thoughts": [], "emotions": []} # 思考や感情
self.memories = memories # 過去の経験や記憶
self.beliefs = beliefs # 信念や価値観
self.social_interactions = social_interactions # 社会的な相互作用
self.alive = True
def think(self, thought):
self.brain['thoughts'].append(thought)
def feel(self, emotion):
self.brain['emotions'].append(emotion)
def interact(self, interaction):
self.social_interactions.append(interaction)
def remember(self, memory):
self.memories.append(memory)
def believe(self, belief):
self.beliefs.append(belief)
def age_up(self):
self.age += 1
return f"{self.name} is now {self.age} years old."
# インスタンスの作成とテスト
japanese_context = Environment("Japanese culture", "Tokyo urban life", "Post-war economic growth")
john = Human("John", 30, "AA genotype")
japanese_context.influence_person(john)
john.think("I wonder about the universe.")
john.feel("happiness")
john.interact("Met with a friend in Shibuya.")
john.remember("Childhood trip to Kyoto.")
john.believe("Hard work pays off.")
print(john.brain)
print(john.memories)
print(john.beliefs)
print(john.social_interactions)
このコードは、人間の複雑さをより詳細にモデル化する試みの一つです。実際の人間の複雑性を完全にモデル化するのは非常に難しいですが、上記の例はその方向性を示す一つの方法として参考にしてください。