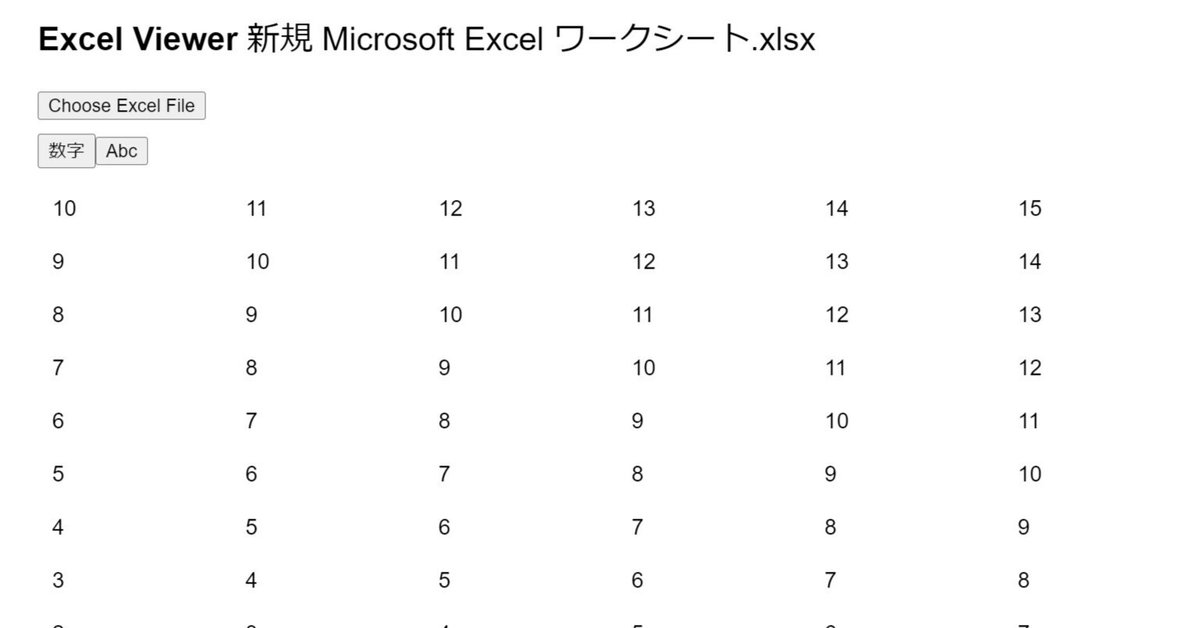
手軽なオンラインのエクセルビューアがないから作った
エクセルの中身だけ確認したいけど、出先でChomebook。
Googleドライブにアップすること面倒という場合のビューアです。
ということでコード載せます。
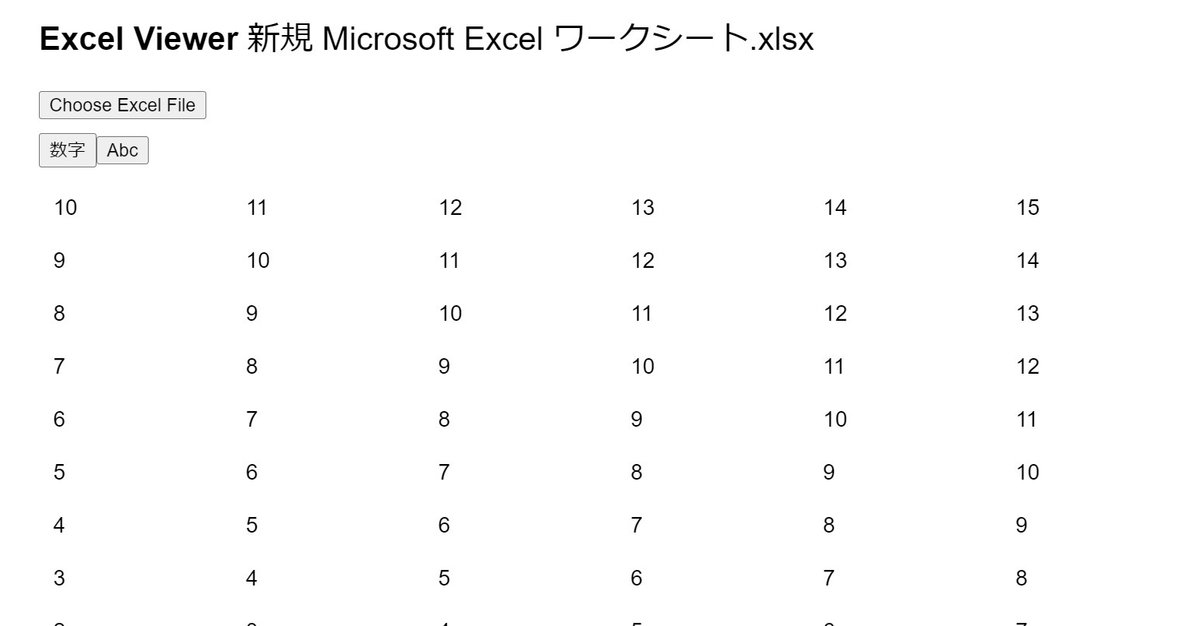
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Excel Viewer</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/xlsx/0.17.0/xlsx.full.min.js"></script>
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
table {
width: 100%;
border-collapse: collapse;
}
th, td {
border: 1px solid white; /* 罫線の色を白に設定 */
padding: 10px;
}
th {
background-color: #f2f2f2;
}
#sheetButtons {
margin: 10px 0; /* ボタンのマージン */
}
</style>
</head>
<body>
<h2>Excel Viewer <span id="fileName" style="font-weight: normal;"></span></h2>
<!-- ボタン -->
<input type="file" id="fileInput" accept=".xlsx, .xls" style="display:none;" />
<button onclick="document.getElementById('fileInput').click();">Choose Excel File</button>
<!-- シート選択ボタン -->
<div id="sheetButtons"></div>
<!-- 結果を表示する場所 -->
<div id="excelData"></div>
<script>
let workbook; // ワークブックの変数を宣言
document.getElementById('fileInput').addEventListener('change', handleFile);
function handleFile(event) {
const file = event.target.files[0];
if (!file) {
return;
}
const reader = new FileReader();
reader.onload = function (e) {
const data = new Uint8Array(e.target.result);
workbook = XLSX.read(data, { type: 'array' });
displaySheetNames(); // シート名のボタンを表示
// ファイル名を表示
document.getElementById('fileName').innerText = file.name;
};
reader.readAsArrayBuffer(file);
}
// シート名のボタンを表示する関数
function displaySheetNames() {
const sheetButtonsDiv = document.getElementById('sheetButtons');
sheetButtonsDiv.innerHTML = ''; // 既存のボタンをクリア
workbook.SheetNames.forEach(sheetName => {
const button = document.createElement('button');
button.innerText = sheetName;
button.onclick = () => displaySheet(sheetName); // シート名をクリックで表示
sheetButtonsDiv.appendChild(button);
});
}
// 選択したシートの内容を表示する関数
function displaySheet(sheetName) {
const sheet = workbook.Sheets[sheetName];
const html = XLSX.utils.sheet_to_html(sheet); // シート内容をHTMLに変換
document.getElementById('excelData').innerHTML = html;
// テーブルの罫線の色を白に設定
const table = document.querySelector('table');
if (table) {
table.style.borderColor = 'white';
}
}
</script>
</body>
</html>